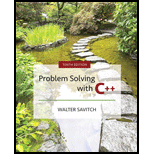
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 7.4, Problem 20STE
Program Plan Intro
Purpose of given code:
The purpose of given code is to store the values in multidimensional array and print these values.
Given code:
/*Include the appropriate headers*/
#include<iostream>
using namespace std;
//Main method
int main()
{
/*Declaration of variables*/
int myArray[4][4], index1, index2;
/*Loop for rows*/
for (index1 = 0; index1 < 4; index1++)
/*Loop for columns*/
for (index2 = 0; index2 < 4; index2++)
/*Assign the values into array*/
myArray[index1][index2] = index2;
/*Loop for rows*/
for (index1 = 0; index1 < 4; index1++)
{
/*Loop for columns*/
for (index2 = 0; index2 < 4; index2++)
/*Print statement with values*/
cout << myArray[index1][index2] << " ";
//Print statement
cout << endl;
}
}
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
typedef struct node_t node_t;
struct node_t {
};
int32_t value;
node_t* next;
node_t* insert (node_t* list, int32_t v, int32_t* flag);
3) As you may have noticed from MT1, Howie is lazy. He wants you to write the interface
for the following implementation of function copy_to_linked_list. He really appreciates your help!
/* copy_to_linked_list
int32_t copy_to_linked_list (int32_t a[], int32_t size, node_t** list_p) {
int32_t flag, i;
for (i = 0; i < size; ++i) {
*list_p = insert (*list_p, a[i], &flag);
if (-1== flag)
return -1;
}
return 0;
def drop_uninformative_objects(X, y):# your code herereturn X_subset, y_subset
# TEST drop_uninformative_objects functionA = pd.DataFrame(np.array([[0, 3, np.nan],[4, np.nan, np.nan],[np.nan, 6, 7],[np.nan, np.nan, np.nan],[5, 5, 5],[np.nan, 8, np.nan],]))b = pd.Series(np.arange(6))A_subset, b_subset = drop_uninformative_objects(A, b)
assert A_subset.shape == (3, 3)assert b_subset.shape == (3,)
ANSWER THIS QUESTION
Why should the **head in insert_atFront function has two asterisk (*)?
Why should the searchNode return a pointer?
Chapter 7 Solutions
Problem Solving with C++ (10th Edition)
Ch. 7.1 - Prob. 1STECh. 7.1 - In the array declaration double score(5); state...Ch. 7.1 - Identity any errors in the following array...Ch. 7.1 - What is the output of the following code? char...Ch. 7.1 - What is the output of the following code? double a...Ch. 7.1 - What is the output of the following code? int i,...Ch. 7.1 - Prob. 7STECh. 7.1 - Suppose we expect the elements of the array a to...Ch. 7.1 - Prob. 9STECh. 7.1 - Suppose you have the following array declaration...
Ch. 7.2 - Consider the following function definition: void...Ch. 7.2 - Prob. 12STECh. 7.2 - Write a function definition for a function called...Ch. 7.2 - Consider the following function definition: void...Ch. 7.2 - Insert const before any of the following array...Ch. 7.2 - Write a function named outOfOrder that takes as...Ch. 7.3 - Write a program that will read up to ten...Ch. 7.3 - Write a program that will read up to ten letters...Ch. 7.3 - Following is the declaration for an alternative...Ch. 7.4 - Prob. 20STECh. 7.4 - Write code that will fill the array a (declared...Ch. 7.4 - Prob. 22STECh. 7 - Write a function named firstLast2 that takes as...Ch. 7 - Write a function named countNum2s that takes as...Ch. 7 - Write a function named swapFrontBack that takes as...Ch. 7 - The following code creates a small phone book. An...Ch. 7 - There are three versions of this project. Version...Ch. 7 - Hexadecimal numerals are integers written in base...Ch. 7 - Solution to Programming Project 7.3 Write a...Ch. 7 - Prob. 4PPCh. 7 - Write a program that reads in a list of integers...Ch. 7 - Prob. 6PPCh. 7 - An array can be used to store large integers one...Ch. 7 - Write a program that will read a line of text and...Ch. 7 - Write a program to score five-card poker hands...Ch. 7 - Write a program that will allow two users to play...Ch. 7 - Write a program to assign passengers seats in an...Ch. 7 - Prob. 12PPCh. 7 - The mathematician John Horton Conway invented the...Ch. 7 - Redo (or do for the first time) Programming...Ch. 7 - Redo (or do for the first time) Programming...Ch. 7 - A common memory matching game played by young...Ch. 7 - Your swim school has two swimming instructors,...Ch. 7 - Your swim school has two swimming instructors,...Ch. 7 - Prob. 19PPCh. 7 - The Social Security Administration maintains an...
Knowledge Booster
Similar questions
- please go in to the detail and explain in detail the purpose and function to each line & function of code listed below. #include <stdio.h>#include <stdlib.h> struct node{int data;struct node *next;}; struct node *head, *tail = NULL; void addNode(int data) {struct node *newNode = (struct node*)malloc(sizeof(struct node));newNode->data = data;newNode->next = NULL; if(head == NULL) {head = newNode;tail = newNode;}else {tail->next = newNode;tail = newNode;}} void removeDuplicate() {struct node *current = head, *index = NULL, *temp = NULL; if(head == NULL) {return;}else {while(current != NULL){temp = current;index = current->next; while(index != NULL) {if(current->data == index->data) {temp->next = index->next;}else {temp = index;}index = index->next;}current = current->next;}}} void display() {struct node *current = head;if(head == NULL) {printf("List is empty \n");return;}while(current != NULL) {printf("%d ", current->data);current =…arrow_forwardPlease check the instructions on the images given. Sample output are also given on the image while the code for modification is provided below. hash.c #include <stdlib.h>#include <stdio.h> struct Node { int val; struct Node * next;}; struct HashTable { int size; struct Node ** table;}; struct Node * create_node(int val);struct HashTable * create_table(int size);void destroy_table(struct HashTable * h);void display_table(struct HashTable *h);int hash_function(int key, int size);void insert(struct HashTable *h, int val);int search(struct HashTable * h, int val);struct Node * delete(struct HashTable * h, int val); void main(){struct HashTable * h = create_table(8);display_table(h); int val_insert[12] = {12, 31, 45, 83, 72, 29, 84, 39, 61, 24, 10, 50}; for (int i=0; i<12; i++) { insert(h,val_insert[i]);}display_table(h); int val_search[4] = {29, 99, 72, 20};for (int i=0; i<4; i++) { int result = search(h,val_search[i]); printf("Search value %2d:…arrow_forwardswap_nums seems to work, but not swap_pointers. Fix it. #include <stdio.h>void swap_nums(int *x, int *y) { int tmp; tmp = *x; *x = *y; *y = tmp; } void swap_pointers(char *x, char *y) { char *tmp; tmp = x; x = y; y = tmp; } int main() { int a,b; char *s1,*s2; a = 3; b=4; swap_nums(&a,&b); printf("a is %d\n", a); printf("b is %d\n", b); s1 = "I should print second"; s2 = "I should print first"; swap_pointers(s1,s2); printf("s1 is %s\n", s1); printf("s2 is %s\n", s2); return 0; }arrow_forward
- 10. write code in c++arrow_forwardchar buffer [BUFSIZE]; int fd = open("fooey", O_WRONLY|O_CREAT|O_TRUNC,0777); strcpy(buffer, "First data"); write(fd, buffer, strlen(buffer)); 1seek (fd, 90, SEEK_SET); strcpy (buffer, "Second data"); write(fd, buffer, strlen(buffer)); 1seek (fd, 100, SEEK_END); strpcy (buffer, "Third data"); write(fd, buffer, strlen(buffer)); close(fd); a. What is the size of the file at the end of this section of code? b. Describe the contents of the file say exactly what byte offsets contain actual data, and what offsets constitute "holes". c. Why might holey files be useful? d. Suppose, after the above sequence of writes, the same program opens the file for reading and then does:arrow_forwardConsider the following code snippet: vector num (3) ; num [0] = 1; num [1] = 2; num [2] = 3; num.pop_back(); Which element or elements of the vector are removed by the pop_back() function in the given code snippet? The element with index 0 The element with index 1 The element with index 2 All the elementsarrow_forward
- The following code doesn't correctly return the index values of the minimum contiguous subsequence. Please modify it so that it does, with comments if possible. def minSumRec(a, left, right): if left == right: return [a[left], left, right] center = int((left + right) / 2) min_left_sum = minSumRec(a, left, center) min_right_sum = minSumRec(a, center + 1, right) min_left_border_sum, left_border_sum = 1e9, 0 for i in range(center, left - 1, -1): left_border_sum += a[i] if left_border_sum < min_left_border_sum: min_left_border_sum = left_border_sum min_right_border_sum, right_border_sum = 1e9, 0 for i in range(center + 1, right + 1): right_border_sum += a[i] if right_border_sum < min_right_border_sum: min_right_border_sum = right_border_sum min_border_sum = min_right_border_sum + min_left_border_sum minSum = min(min_left_sum[0], min_right_sum[0], min_border_sum) if minSum == min_left_sum: return min_left_sum if minSum == min_right_sum: return min_right_sum return…arrow_forwardHow does the --> work in dereferencing a pointer?arrow_forwardm = int(temp[1]) IndexError: list index out of range line 3, in <module> m = int(temp[10])IndexError: list index out of range when i ran this test case input: 3 jun3 Jin1 Li2 Kitty2 Josh3 Bob1 Dave2 Jose1 David3 Rob3 Anne3 Ann2 Kevin2 Lara1 ALI3 Xin expected output: Li Dave David ALI Kitty Josh Kevin Lara jun Bob Anne Ann Xinarrow_forward
- My homework was to design and implement a simple social network program in Java. I should use an adjacency matrix data structure in my implementation. Write a social network program in Java. The default information for this network is stored in two files: index.txt and friend.txt. The file index.txt stores the names of the people in the network – you may assume that we only store the given names and these names are unique; and friend.txt stores who knows whom. The program must read these two files. The following section describes the format of these two files. The friend.txt takes the following format. The first line is the number of pairs of friends. Each subsequent line has two integer numbers. The first two numbers are the indices of the names. The following is an example of friend.txt:50 31 30 12 41 5 The index.txt stores the names of the people in the network. The first line is the number of people in the file; for example:60 Gromit1 Gwendolyn2 Le-Spiderman3 Wallace4 Batman5…arrow_forward# viewGame(G, i) takes a game description G (a dictionary of the type# produced by newGame()) and an integer player index i and returns a# string that, when printed, describes the state of the game from# player i's perspective.## Example:# >>> viewGame(G, 1)# '\nPlayer2 to play (score=0):\n 1[ ] 2[ ] 3[ ]'## Note the spacing and explicit newlines, and the fact that the player# numbers use 1-based indexing even though internally the game data# structures use Pythonic 0-based indexing.arrow_forwardHef sharks_minnows (minnows, sharks): shark_count = 0 minnow_count = len (minnows) for i in range(minnow_count): curr_shark_height = minnows [i] if curr_shark_height is not None: minnows [i] = None for j in range (i + 1, minnow_count): if minnows [j] == curr_shark_height: minnows [j] = None curr_shark_height -- 1 shark_count += 1 return shark_count <= sharks The provided code is imperfect, in that it sometimes returns True when it should return False, and sometimes returns False when it should return True. (a) Provide an example of a function call where the provided code will correctly return True (i.e. a True Positive) (b) Provide an example of a function call where the provided code will correctly return False (i.e. a True Negative) (c) Provide an example of a function call where the provided code will incorrectly return True (i.e. a False Positive) (d) Provide an example of a function call where the provided code will incorrectly return False (i.e. a False Negative)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
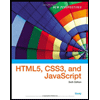
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning