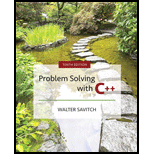
Following is the declaration for an alternative version of the function search defined in Display 7.12. In order to use this alternative version of the search function, we would need to rewrite the program slightly, but for this exercise all you need to do is to write the function definition for this alternative version of search.
bool search (const int a[], int numberUsed,
int target, int& where);
//Precondition: numberUsed is <= the declared size of the
//array a; a[0] through a[numberUsed – 1] have values.
//Postcondition: If target is one of the elements a[0]
//through a[numberUsed – 1], then this function returns
//true and sets the value of where so that a[where] ==
//target; otherwise this function returns false and the
//value of where is unchangad.

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Starting Out With Visual Basic (8th Edition)
Starting Out with Python (3rd Edition)
Java How To Program (Early Objects)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Concepts Of Programming Languages
Artificial Intelligence: A Modern Approach
- Create a program that accepts an integer N, and pass it to the function generatePattern. generatePattern() function which has the following description: Return type - void Parameter - integer n This function prints a right triangular pattern of letter 'T' based on the value of n. The top of the triangle starts with 1 and increments by one down on the next line until the integer n. For each row of in printing the right triangle, print "T" for n times. In the main function, call the generatePattern() function. Input 1. One line containing an integer Output Enter N: 4 T TT TTT TTTTarrow_forwardIn java there must be at least two calls to the function with different arguments and the output must clearly show the task being performed. (ONLY ARRAYS or ARRAYLIST) Develop a function that accepts an array and returns true if the array contains any duplicate values or false if none of the values are repeated. Develop a function that returns true if the elements are in decreasing order and false otherwise. A “peak” is a value in an array that is preceded and followed by a strictly lower value. For example, in the array {2, 12, 9, 8, 5, 7, 3, 9} the values 12 and 7 are peaks. Develop a function that returns the number of peaks in an array of integers. Note that the first element does not have a preceding element and the last element is not followed by anything, so neither the first nor last elements can be peaks. Develop a function that finds the starting index of the longest subsequence of values that is strictly increasing. For example, given the array {12, 3, 7, 5, 9, 8,…arrow_forwardWrite in C++ Alice is trying to monitor how much time she spends studying per week. She going through her logs, and wants to figure out which week she studied the least, her total time spent studying, and her average time spent studying per week. To help Alice work towards this goal, write three functions: min(), total(), and average(). All three functions take two parameters: an array of doubles and the number of elements in the array. Then, they make the following computations: min() - returns the minimum value in the array sum() - returns the sum of all the values in the array average() - returns the average of all the values in the array You may assume that the array will be non-empty. Function specifications: Function 1: Finding the minimum hours studied Name: min() Parameters (Your function should accept these parameters IN THIS ORDER): arr double: The input array containing Alice's study hours per week arr_size int: The number of elements stored in the array Return Value:…arrow_forward
- Please complete this program in C++ Please enusre that there is plenty of comments. PLENTY even if unessessary. Please include screenshots of actual code, screenshots of output, and code in the browser so I can copy and paste. Thank you.arrow_forwardUse Clojure: a. The built-in function type is useful for checking what kind of object an expression evaluates to. Write a function both-same-type? that takes two arguments, and returns true when they both have the same type, and false otherwise. b. Write a function list-longer-than? which takes two arguments: an integer n, and a list lst and returns true if lst has more than n elements, and false otherwise. For example, (list-longer-than? 3 '(1 2 3)) should return false, and (list-longer-than? 2 '(1 2 3)) should return true.arrow_forwardIn c++arrow_forward
- C++ Format There's an old joke: "Why is 6 scared of 7? Because 7 8 9 (seven ate nine)." But since hearing that joke, I've had an irrational fear of the number 7. Write a templated function, called "safe_add" that adds two numbers (ints, floats, doubles, etc) and returns there sum. However, if either number (or their sum) contains the number 7, raise a runtime_error stating "can't add because 6+1 appears in it".arrow_forwardIn C++ Write a recursive function that displays a string reversely on the console using the following header:void reverseDisplay(const string& s) For example, reverseDisplay("abcd") displays dcba. Write a test programthat prompts the user to enter a string and displays its reversal.arrow_forwardint p =5 , q =6; void foo ( int b , int c ) { b = 2 * c ; p = p + c ; c = 1 + p ; q = q * 2; print ( b + c ); } main () { foo (p , q ); print p , q ; } Explain and print the output of the above code when the parameters to the foo function are passed by value. Explain and print the output of the above code when the parameters to the foo function are passed by reference. Explain and print the output of the above code when the parameters to the foo function are passed by value result. Explain and print the output of the above code when the parameters to the foo function are passed by name.arrow_forward
- void getChar(char& c, istream& infile, int& i){ infile.get(c); if (c == '\n') i++;} COULD YOU REWRITTE THE FUNCTION ABOVE. AND BY REWRITE. I MEAN CHANGE THE FUNCTION NAME, PARAMETERS, AND ITS IMPLEMENTATION. HOWEVER, THE FUNCTION MUST STILL ACHEIVE THE SAME GOAL.arrow_forwardin c++ language pleasssearrow_forwardWrite in C++ When you're processing data, it’s useful to break up a text string into pieces using a delimiter. Write a function split() that takes a string, splits it at every occurrence of a delimiter, and then populates an array of strings with the split pieces, up to the provided maximum number of pieces. Function specifications: Name: split() Parameters (Your function should accept these parameters IN THIS ORDER): input_string string: The text string containing data separated by a delimiter separator char: The delimiter marking the location where the string should be split up arr string array: The array that will be used to store the input text string's individual string pieces arr_size int: The number of elements that can be stored in the array Return Value: int: The number of pieces the input text string was split into Note: No input will have delimiters in the beginning or the end of the string. (Eg: ",apple, orange" OR "apple, orange,") No input will have multiple…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
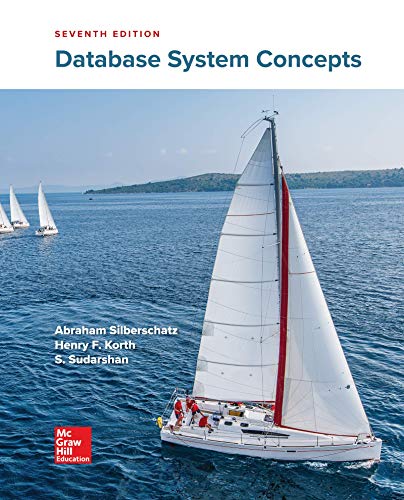
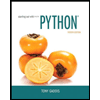
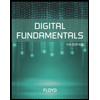
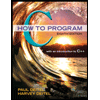
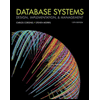
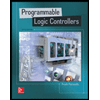