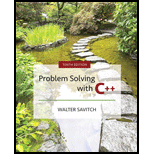
Concept explainers
Write a

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Web Development and Design Foundations with HTML5 (8th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Modern Database Management
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Vector Mechanics for Engineers: Statics and Dynamics
- Program thisarrow_forwardA positive integer greater than 1 is said to be prime if it has no divisors other than 1 and itself. Write a program that asks the user to input an integer greater than 1, then display all of the prime numbers that less than or equal to the number entered. The program should work as follows: • Once the user has entered a number, the program should display an array with all of the integers from 2 up through the value entered. • The program should then use a loop to step through the array. The loop should pass each element to a method that displays the element whether it is prime number. Your result should look like, for example: Enter an integer greater than 1: 9 2, 3, 4, 5, 6, 7, 8, 9 2 is prime 3 is prime 4 is not prime 5 is prime 6 is not prime 7 is prime 8 is not prime 9 is not prime Using java programmingarrow_forwardJava Program - Functions with 2D Arrays Write a function named displayElements that takes a two-dimensional array, the size of its rows and columns, then prints every element of a two-dimensional array. Separate every row by a new line and every column by a space. In the main function, write a program that asks for integer input to be assigned on a 3 x 3 array and call the function displayElements to print the contents on the next line. Input 1. Multiple lines containing integer each line Output R1C1: 1 R1C2: 2 R1C3: 3 R2C1: 4 R2C2: 5 R2C3: 6 R3C1: 7 R3C2: 8 R3C3: 9 1 2 3 4 5 6 7 8 9arrow_forward
- This program will store roster and rating information for a soccer team. Coaches rate players during tryouts to ensure a balanced team. (1) Prompt the user to input five pairs of numbers: A player's jersey number (0 - 99) and the player's rating (1 - 9). Store the jersey numbers in one int array and the ratings in another int array. Output these arrays (i.e., output the roster). Ex: Enter player 1's jersey number: 84 Enter player 1's rating: 7 Enter player 2's jersey number: 23 Enter player 2's rating: 4 Enter player 3's jersey number: 4 Enter player 3's rating: 5 Enter player 4's jersey number: 30 Enter player 4's rating: 2 Enter player 5's jersey number: 66 Enter player 5's rating: 9 ROSTER Player 1 -- Jersey number: 84,Rating: 7 Player 2 -- Jersey number: 23, Rating: 4 ... (2) Implement a menu of options for a user to modify the roster. Each option is represented by a single character. The program initially outputs the menu, and outputs the menu after a user chooses an option. The…arrow_forwardThis program will store roster and rating information for a soccer team. Coaches rate players during tryouts to ensure a balanced team. (1) Prompt the user to input five pairs of numbers: A player's jersey number (0 - 99) and the player's rating (1 - 9). Store the jersey numbers in one int array and the ratings in another int array. Output these arrays (i.e., output the roster). Ex: Enter player 1's jersey number: 84 Enter player 1's rating: 7 Enter player 2's jersey number: 23 Enter player 2's rating: 4 Enter player 3's jersey number: 4 Enter player 3's rating: 5 Enter player 4's jersey number: 30 Enter player 4's rating: 2 Enter player 5's jersey number: 66 Enter player 5's rating: 9 ROSTER Player 1 -- Jersey number: 84, Rating: 7 Player 2 -- Jersey number: 23, Rating: 4 (2) Implement a menu of options for a user to modify the roster. Each option is represented by a single character. The program initially outputs the menu, and outputs the menu after a user chooses an option. The…arrow_forwardMulti Dimensional Arrays in C Program Ask the user for the number of rows and columns of a two-dimensional array and then its integer elements. Then, ask the user for another integer value which represents a boogeyman. A boogeyman is a value that we intend to find from a given array. Find and output the coordinates of the boogeyman by this format: "BOOGEYMAN LOCATED AT ROW {row_number}, COLUMN {column_number}!" Input 1. One line containing an integer for the number of rows 2. One line containing an integer for the number of columns 3. Multiple lines containing at least one integer each line for the elements of the array Sample 1 4 2 3 9 1 2 3 4 2 1 1 9 2 8 3 4. One line containing an integer for the boogeyman's value Output Print the row of the position first and then the column of the position. Note that the row and the column is 0-based (i.e. it starts at 0 and not at 1). It is guaranteed that the boogeyman exists and only once in the 2D array. Enter# of rows: 4 Enter…arrow_forward
- JAVA Your teacher has created two arrays, each holding the results of tests, say Test 1 and Test 2. You need to create a new array which holds the averages of these two tests. You may assume that the first element of the first test array refers to the student who also has the grade in the first element of the second test, and the last element in each array are the grades the last student earned for each test. All students received grades for both tests, meaning both test arrays are of the same length. Complete the method, named makeAverage, in the class named Grades.java. There are two parameters to this method: the first is the integer array representing the grades of the first test, and the second is the array containing the grades of the second test. The new average array should be returned by the method. The grades should be treated as double variables. For example, consider the test grades for the five students in the following arrays: [ 87 ] [ 91 ] [ 76 ] [ 76 ] [ 94 ]…arrow_forward8. A magic square is an n by n square in which each element is an integer between 1 and n*n and all column sums, row sums and diagonal sums are equal. For example, the following is a 3 by 3 magic square in which each row, each column, and each diagonal adds up to 15. 4 3 8 9 1 7 6. Write a program that accepts a two-dimensional array size 10 by 10. The program should check and output whether it is a magic square or not. What is the output of the following code?arrow_forwardIn C++Monkey Business A local zoo wants to keep track of how many pounds of food each of its three monkeys eats each day during a typical week. Write a program that stores this information in a two-dimensional 3 × 5 array, where each row represents a different monkey, and each column represents a different day of the week. The program should first have the user input the data for each monkey. Then, it should create a report that includes the following information: Average amount of food eaten per day by the whole family of monkeys. The least amount of food eaten during the week by any one monkey. The greatest amount of food eaten during the week by any one monkey. Write functions: average() least() greatest() main()arrow_forward
- The Lo Shu Magic Square is a grid with 3 rows and 3 columns shown below. The Lo Shu Magic Square has the following properties: The grid contains the numbers 1 – 9 exactly The sum of each row, each column and each diagonal all add up to the same number. This is shown below: Write a program that simulates a magic square using 3 one dimensional parallel arrays of integer type. Do not use two-dimensional array. Each one the arrays corresponds to a row of the magic square. The program asks the user to enter the values of the magic square row by row and informs the user if the grid is a magic square or not. Project Specifications Input for this project: Values of the grid (row by row) Output for this project: Whether or not the grid is magic square Processing Requirements Use the following template to start your project: #include<iostream> using namespace std; // Global constants const int ROWS = 3; // The number of rows in the array const int COLS = 3; // The number of columns…arrow_forwardThe Lo Shu Magic Square is a grid with 3 rows and 3 columns shown below. The Lo Shu Magic Square has the following properties: The grid contains the numbers 1 – 9 exactly The sum of each row, each column and each diagonal all add up to the same number. This is shown below: Write a program that simulates a magic square using 3 one dimensional parallel arrays of integer type. Do not use two-dimensional array. Each one the arrays corresponds to a row of the magic square. The program asks the user to enter the values of the magic square row by row and informs the user if the grid is a magic square or not. Processing Requirements - c++ Use the following template to start your project: #include<iostream> using namespace std; // Global constants const int ROWS = 3; // The number of rows in the array const int COLS = 3; // The number of columns in the array const int MIN = 1; // The value of the smallest number const int MAX = 9; // The value of the largest number //…arrow_forwardMake a program in JavaIn this simulation there are 7 countries competing { Mexico, Canada, China, Japan, Spain, Australia, Italy}2.There are three types of dents {Gold, Silver, Bronze}3.Use constant values for array dimensions4.Country names and medal types should be stored in an array.5.Randomly assign values between 1 and 10 that represent the number of medals of the corresponding type that the corresponding country has won.6.Show a table that represents the information of the medal table. Table must include country header names and medal type7.Show the total number of medals that each country obtained8.Show the name of the country that got the most medals, no matter the type of medal.9.Show the name of the country that obtained the most Gold medalsarrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
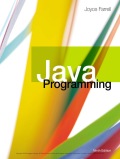