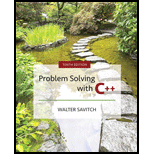
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 7, Problem 4PP
Program Plan Intro
Program Plan:
- Include the appropriate headers into program.
- Declare and define the constant variable “LEN”.
- Declare the appropriate functions.
- Define the “main()” function.
- Declare the array variable “arr[]” in type of double.
- Declare the variables “stdDeviation” and “avg” in type of double.
- Declare the variable “ans” in type of character.
- Using “do…while” loop, prompt the user for array values.
- Call the function “stdDev()” which passes array and size of array and store the result in “stdDeviation”.
- Prompt and get the sentinel value from user.
- Check the loop with condition for sentinel value.
- Return “0” to called function.
- Define the function named “stdDev()” which passes the arguments “arr[]” in type of character and “size” in type of integer.
- Declare and initialize the “sumSquares” in type of “double”.
- Call the function “average()” which passes array and size of array as arguments and stores the result in “avg” variable.
- Using “for” loops, calculate the summation value.
- Return the square root of summation value.
- Define the function named “average()” which passes “arr[]” and “size” as arguments.
- Declare and initialize the “sum” in type of “double”.
- Using “for” loop, calculate the total of array values.
- Return the average of array values.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Set Let S = {1, 4} and T = {a, b, c}, computer:
S X T =
S X S =
|T| =
T − S =
P(S) =
the value of factor(P/F,i,10) can be found by getting the factor values for(P/F,i,4) and(P/f,i,6)and adding it to
O(nlgn) means that there is function f(n) that is O(nlgn) which is an upper bound for the running time at large n
Select one:
True
False
Chapter 7 Solutions
Problem Solving with C++ (10th Edition)
Ch. 7.1 - Prob. 1STECh. 7.1 - In the array declaration double score(5); state...Ch. 7.1 - Identity any errors in the following array...Ch. 7.1 - What is the output of the following code? char...Ch. 7.1 - What is the output of the following code? double a...Ch. 7.1 - What is the output of the following code? int i,...Ch. 7.1 - Prob. 7STECh. 7.1 - Suppose we expect the elements of the array a to...Ch. 7.1 - Prob. 9STECh. 7.1 - Suppose you have the following array declaration...
Ch. 7.2 - Consider the following function definition: void...Ch. 7.2 - Prob. 12STECh. 7.2 - Write a function definition for a function called...Ch. 7.2 - Consider the following function definition: void...Ch. 7.2 - Insert const before any of the following array...Ch. 7.2 - Write a function named outOfOrder that takes as...Ch. 7.3 - Write a program that will read up to ten...Ch. 7.3 - Write a program that will read up to ten letters...Ch. 7.3 - Following is the declaration for an alternative...Ch. 7.4 - Prob. 20STECh. 7.4 - Write code that will fill the array a (declared...Ch. 7.4 - Prob. 22STECh. 7 - Write a function named firstLast2 that takes as...Ch. 7 - Write a function named countNum2s that takes as...Ch. 7 - Write a function named swapFrontBack that takes as...Ch. 7 - The following code creates a small phone book. An...Ch. 7 - There are three versions of this project. Version...Ch. 7 - Hexadecimal numerals are integers written in base...Ch. 7 - Solution to Programming Project 7.3 Write a...Ch. 7 - Prob. 4PPCh. 7 - Write a program that reads in a list of integers...Ch. 7 - Prob. 6PPCh. 7 - An array can be used to store large integers one...Ch. 7 - Write a program that will read a line of text and...Ch. 7 - Write a program to score five-card poker hands...Ch. 7 - Write a program that will allow two users to play...Ch. 7 - Write a program to assign passengers seats in an...Ch. 7 - Prob. 12PPCh. 7 - The mathematician John Horton Conway invented the...Ch. 7 - Redo (or do for the first time) Programming...Ch. 7 - Redo (or do for the first time) Programming...Ch. 7 - A common memory matching game played by young...Ch. 7 - Your swim school has two swimming instructors,...Ch. 7 - Your swim school has two swimming instructors,...Ch. 7 - Prob. 19PPCh. 7 - The Social Security Administration maintains an...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Input: A set of n movies. A positive integer k. A positive integer q. A function f that takes two movies X, Y, and gives a positive similarity score f(X,Y) in constant time. Task: Design an efficient algorithm that partitions the movies into exactly k disjoint sets such that movies from different sets have a similarity score at most q. If such groups cannot be made, then print 'Unsatisfiable'. Give an efficient algorithm (to the best of your knowledge) and a formal proof of correctness for your algorithm. An answer without any formal proof will be considered incomplete. Analyze the running time of the algorithm. You must write down your algorithm as pseudocode or describe it as a set of steps (as we do in the class). Do not provide code that is written in a code editor using C/C++/Python/Java, etc.arrow_forwardA decreasing sequence of numbers is a sequence of integers where every integer in the sequence is smaller than all other previous integers in that sequence. For example, •35, 16, 7, 2, 0, -3, -9 is a decreasing sequence of numbers. The length of this sequence is 7 (total numbers in the sequence) and the difference of this sequence is 35 - (-9) -44. • 5 is a decreasing sequence of numbers with length 1 and difference 5-5 = 0 •99,-99 is a decreasing sequence of numbers with length 2 and difference 99-(-99) = 198 •17, 23, 11, 8, -5, -3 is not a decreasing sequence of %3D numbers. Write a program that contains a main() function. The main function repeatedly asks the user to enter an integer if the previously entered integers form a decreasing sequence of numbers. This process stops as soon as the latest user input breaks the decreasing sequence. Then your function should print the length and difference of the decreasing sequence. Finally, call the main() function such that the call will be…arrow_forwardInput: A set of n movies. A positive integer k. A positive integer q. A function f that takes two movies X, Y, and gives a positive similarity score f(X, Y) in constant time. Task: Design an efficient algorithm that partitions the movies into exactly k disjoint sets such that movies from different sets have a similarity score at most q. If such groups cannot be made, then print 'Unsatisfiable'. Yeah, please what is the time complexity for this algorithm, thanks.arrow_forward
- Generate 10000 random numbers that are normally distributed with u=5.0 and O=2.0. Then, calculate the root mean square (RMS) of these random numbers.arrow_forwardAs an investor, I always check the stock market in order to find good companies to invest in. Recently, I found that the best companies to invest in, are the ones that have largest sum formed by a strictly increasing set of numbers (a set where the next element is always greater than the current element). But before I invest, I need to know the position of the first element of the consecutive increasing numbers. Help me so we can start investing already! Note: If it is already the last element of the row in the array, the next element is the first element of the next row, if there exists a next row. Input 1. Number of rows Description This is the number of rows of the multidimensional array. 2. Number of columns Description This is the number of columns of the multidimensional array. 3. Elements of the multidimensional array Output The first line will contain a message prompt to input the number of rows. The second line will contain a message prompt to input the…arrow_forwardBowling Stat's D.B W R В — 1 В - 2 В — 3 X%3D В —4 В — 5 4 10 2 27 1 14 13 40 3 5 7 12 2 3 22 37, As you can see, a particular row corresponds to a bowler, while the columns represent the information of overs bowled (O), dot-balls (D.B), wickets taken (W) and runs conceded (R). For example, Bowler (B-2) bowled 3 overs, 5 dot-balls, 3 wickets taken, and 14 runs conceded. Stats for other bowlers are represented by the other rows in a similar fashion. Write a MATLAB® script to compute a) The economy rate for each bowler. (hint: In cricket, a bowler's economy rate is total number of runs they have conceded per over bowled.) b) Which bowler has the lowest bowling average. (a player's bowling average is the number of runs they have conceded per wicket taken. hint: min command with index value will be helpful here). c) The total number of dot balls bowled by all five bowlers. (the sum command with appropriate dimension argument will be helpful here; see sum documentation for further help). d)…arrow_forward
- Q4/Full the following blanks (1101 1010)BCD(5211) = ( )BCD(3321)=()EX-3=()16 * (1101 1010)BCD(5211) = (1110 1100)BCD(3321)=(1010110)EX-3= (A6)16 (1101 1010)BCD(5211) = (1110 0111)BCD(3321)=(1011001)EX-3= (56)16 (1101 1010)BCD(5211) = (1101 0111)BCD(3321)=(1011001)EX-3= (2B)16 (1101 1010)BCD(5211) = (1011 1110)BCD(3321)=(1000111)EX-3= (44)16 None of them IIarrow_forwardOne-dimensional range searching can be done with O(N log N) steps for preprocessing and O(R+log N) for range searching, where R is the number of points actually falling in the range.arrow_forwardSuppose the function X₂+1 = (ax₂ + c) mod m is used to generate pseudo random number. Assume: m=11₁a-6,c=2, xo=5, what are x₁, x2 and x3 ?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
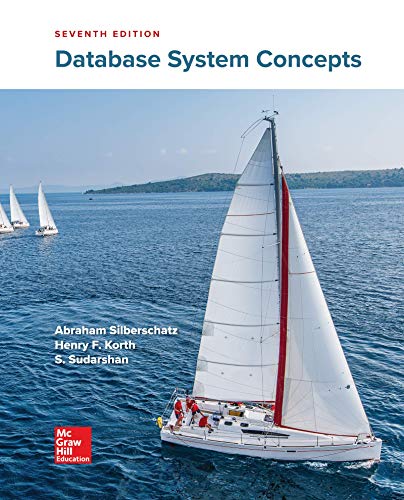
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
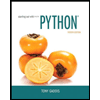
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
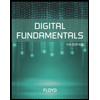
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
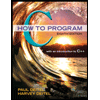
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
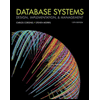
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
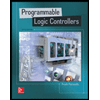
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education