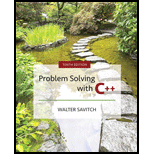
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 7.2, Problem 11STE
Consider the following function definition:
void tripler (int& n)
{
n = 3*n;
}
Which of the following are acceptable function calls?
int a[3] = {4, 5, 6}, number = 2;
tripler (number);
tripler (a[2]);
tripler (a[3]);
tripler (a[number]);
tripler (a);
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
I want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)?When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene.Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency.To elaborate, what…
Male comedians were typically the main/dominant star of television sitcoms made during the FCC licensing freeze.
Question 19 options:
True
False
In the episode of The Honeymooners that you watched this week, why did Alice decide to get a job outside of the home?
Question 1 options:
to earn enough money to buy a mink coat
to have something to do while the kids were at school
to pay the bills after her husband got laid off
After the FCC licensing freeze was lifted, sitcoms featuring urban settings and working class characters became far less common.
Question 14 options:
True
False
Chapter 7 Solutions
Problem Solving with C++ (10th Edition)
Ch. 7.1 - Prob. 1STECh. 7.1 - In the array declaration double score(5); state...Ch. 7.1 - Identity any errors in the following array...Ch. 7.1 - What is the output of the following code? char...Ch. 7.1 - What is the output of the following code? double a...Ch. 7.1 - What is the output of the following code? int i,...Ch. 7.1 - Prob. 7STECh. 7.1 - Suppose we expect the elements of the array a to...Ch. 7.1 - Prob. 9STECh. 7.1 - Suppose you have the following array declaration...
Ch. 7.2 - Consider the following function definition: void...Ch. 7.2 - Prob. 12STECh. 7.2 - Write a function definition for a function called...Ch. 7.2 - Consider the following function definition: void...Ch. 7.2 - Insert const before any of the following array...Ch. 7.2 - Write a function named outOfOrder that takes as...Ch. 7.3 - Write a program that will read up to ten...Ch. 7.3 - Write a program that will read up to ten letters...Ch. 7.3 - Following is the declaration for an alternative...Ch. 7.4 - Prob. 20STECh. 7.4 - Write code that will fill the array a (declared...Ch. 7.4 - Prob. 22STECh. 7 - Write a function named firstLast2 that takes as...Ch. 7 - Write a function named countNum2s that takes as...Ch. 7 - Write a function named swapFrontBack that takes as...Ch. 7 - The following code creates a small phone book. An...Ch. 7 - There are three versions of this project. Version...Ch. 7 - Hexadecimal numerals are integers written in base...Ch. 7 - Solution to Programming Project 7.3 Write a...Ch. 7 - Prob. 4PPCh. 7 - Write a program that reads in a list of integers...Ch. 7 - Prob. 6PPCh. 7 - An array can be used to store large integers one...Ch. 7 - Write a program that will read a line of text and...Ch. 7 - Write a program to score five-card poker hands...Ch. 7 - Write a program that will allow two users to play...Ch. 7 - Write a program to assign passengers seats in an...Ch. 7 - Prob. 12PPCh. 7 - The mathematician John Horton Conway invented the...Ch. 7 - Redo (or do for the first time) Programming...Ch. 7 - Redo (or do for the first time) Programming...Ch. 7 - A common memory matching game played by young...Ch. 7 - Your swim school has two swimming instructors,...Ch. 7 - Your swim school has two swimming instructors,...Ch. 7 - Prob. 19PPCh. 7 - The Social Security Administration maintains an...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
Consider the following C program void fun (void) { int a, b, c; / defiinition.1 / . . . while (. . .) int b, c,...
Concepts Of Programming Languages
The file produced by the Java compiler containsthat are executed by the Java Virtual Machine.
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Find the no-load value of υo in the circuit shown.
Find υo when RL is 150 Ω.
How much power is dissipated in th...
Electric Circuits. (11th Edition)
Repeat Practice Program 5 of Chapter 3, but use a loop so the user can convert other temperatures. If the user ...
Java: An Introduction to Problem Solving and Programming (8th Edition)
Porter’s competitive forces model: The model is used to provide a general view about the firms, the competitors...
Management Information Systems: Managing The Digital Firm (16th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- solve this questions for me .arrow_forwarda) first player is the minimizing player. What move should be chosen?b) What nodes would not need to be examined using the alpha-beta pruning procedure?arrow_forwardConsider the problem of finding a path in the grid shown below from the position S to theposition G. The agent can move on the grid horizontally and vertically, one square at atime (each step has a cost of one). No step may be made into a forbidden crossed area. Inthe case of ties, break it using up, left, right, and down.(a) Draw the search tree in a greedy search. Manhattan distance should be used as theheuristic function. That is, h(n) for any node n is the Manhattan distance from nto G. The Manhattan distance between two points is the distance in the x-directionplus the distance in the y-direction. It corresponds to the distance traveled along citystreets arranged in a grid. For example, the Manhattan distance between G and S is4. What is the path that is found by the greedy search?(b) Draw the search tree in an A∗search. Manhattan distance should be used as thearrow_forward
- whats for dinner? pleasearrow_forwardConsider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand. ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page) main()arrow_forwardWhat is the correct python code for the function def countWords(string) that will return a count of all the words in the string string of workds that are separated by spaces.arrow_forward
- Consider the following program that counts the number of spaces in a user-supplied string. Modify the program to define and use a function, countSpaces, instead. def main() : userInput = input("Enter a string: ") spaces = 0 for char in userInput : if char == " " : spaces = spaces + 1 print(spaces) main()arrow_forwardWhat is the python code for the function def readFloat(prompt) that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Here is a typical usage: salary = readFloat("Please enter your salary:") percentageRaise = readFloat("What percentage raise would you like?")arrow_forwardassume python does not define count method that can be applied to a string to determine the number of occurances of a character within a string. Implement the function numChars that takes a string and a character as arguments and determined and returns how many occurances of the given character occur withing the given stringarrow_forward
- Consider the ER diagram of online sales system above. Based on the diagram answer the questions below, a) Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key. b) Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M c) Is there a direct relationship that exists between Store and Customer entities? Answer Yes/No? d) Which of the 4 Entities mention in the diagram can have a recursive relationship? e) If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type?arrow_forwardNo aiarrow_forwardGiven the dependency diagram of attributes {C1,C2,C3,C4,C5) in a table shown in the following figure, (the primary key attributes are underlined)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
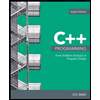
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
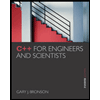
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
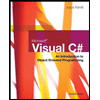
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
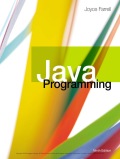
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
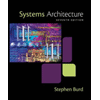
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
What Are Data Types?; Author: Jabrils;https://www.youtube.com/watch?v=A37-3lflh8I;License: Standard YouTube License, CC-BY
Data Types; Author: CS50;https://www.youtube.com/watch?v=Fc9htmvVZ9U;License: Standard Youtube License