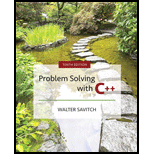
Suppose we expect the elements of the array a to be ordered so that
a [0] ≤ a [1] ≤ a [2] ≤ …
However, to be safe we want our program to test the array and issue a warning in case it turns out that some elements are out of order. The following code is supposed to output such a warning, but it contains a bug. What is it?
double a[10];
<Some code to fill the array a goes here.>
for (int index = 0; index < 10; index++)
if (a[index] > a[index + 1])
cout << “Array elements ” << index << “ and”
<< (index +1) << “are out of order.”;

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Concepts Of Programming Languages
Java: An Introduction to Problem Solving and Programming (8th Edition)
Database Concepts (8th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Web Development and Design Foundations with HTML5 (8th Edition)
- You are asked to explain what a computer virus is and if it can affect computer’shardware or software. How do you protect your computer against virus? give one reference with your answer.arrow_forwardDistributed Systems: Consistency Models fer to page 45 for problems on data consistency. structions: Compare different consistency models (e.g., strong, eventual, causal) for distributed databases. Evaluate the trade-offs between availability and consistency in a given use case. Propose the most appropriate model for the scenario and explain your reasoning. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440AZF/view?usp=sharing]arrow_forwardOperating Systems: Deadlock Detection fer to page 25 for problems on deadlock concepts. structions: • Given a system resource allocation graph, determine if a deadlock exists. If a deadlock exists, identify the processes and resources involved. Suggest strategies to prevent or resolve the deadlock and explain their trade-offs. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forward
- Artificial Intelligence: Heuristic Evaluation fer to page 55 for problems on Al search algorithms. tructions: Given a search problem, propose and evaluate a heuristic function. Compare its performance to other heuristics based on search cost and solution quality. Justify why the chosen heuristic is admissible and/or consistent. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 75 for graph-related problems. Instructions: • Implement a greedy graph coloring algorithm for the given graph. • Demonstrate the steps to assign colors while minimizing the chromatic number. • Analyze the time complexity and limitations of the approach. Link [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 150 for problems on socket programming. Instructions: • Develop a client-server application using sockets to exchange messages. • Implement both TCP and UDP communication and highlight their differences. • Test the program under different network conditions and analyze results. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward
- Refer to page 80 for problems on white-box testing. Instructions: • Perform control flow testing for the given program, drawing the control flow graph (CFG). • Design test cases to achieve statement, branch, and path coverage. • Justify the adequacy of your test cases using the CFG. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qo Hazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 10 for problems on parsing. Instructions: • Design a top-down parser for the given grammar (e.g., recursive descent or LL(1)). • Compute the FIRST and FOLLOW sets and construct the parsing table if applicable. • Parse a sample input string and explain the derivation step-by-step. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 20 for problems related to finite automata. Instructions: • Design a deterministic finite automaton (DFA) or nondeterministic finite automaton (NFA) for the given language. • Minimize the DFA and show all steps, including state merging. • Verify that the automaton accepts the correct language by testing with sample strings. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward
- Refer to page 60 for solving the Knapsack problem using dynamic programming. Instructions: • Implement the dynamic programming approach for the 0/1 Knapsack problem. Clearly define the recurrence relation and show the construction of the DP table. Verify your solution by tracing the selected items for a given weight limit. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440AZF/view?usp=sharing]arrow_forwardRefer to page 70 for problems related to process synchronization. Instructions: • • Solve a synchronization problem using semaphores or monitors (e.g., Producer-Consumer, Readers-Writers). Write pseudocode for the solution and explain the critical section management. • Ensure the solution avoids deadlock and starvation. Test with an example scenario. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward15 points Save ARS Consider the following scenario in which host 10.0.0.1 is communicating with an external SMTP mail server at IP address 128.119.40.186. NAT translation table WAN side addr LAN side addr (c), 5051 (d), 3031 S: (e),5051 SMTP B D (f.(g) 10.0.0.4 server 138.76.29.7 128.119.40.186 (a) is the source IP address at A, and its value. S: (a),3031 D: (b), 25 10.0.0.1 A 10.0.0.2. 1. 138.76.29.7 10.0.0.3arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
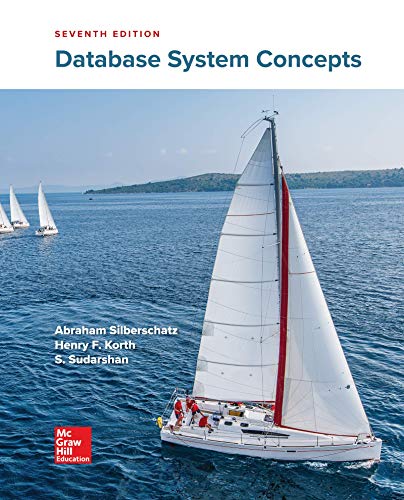
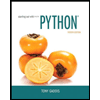
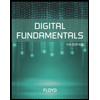
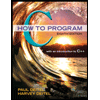
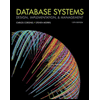
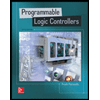