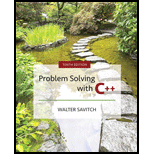
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 7.4, Problem 22STE
Explanation of Solution
Function definition:
//Function definition
void echo(const int a[][5], int len)
{
//Loop for rows
for (int i = 0; i < len; i++)
{
//Loop for columns
for (int j = 0; j < 5; j++)
{
//Print the result
cout << a[i][j] << " ";
}
//Print statement
cout << endl;
}
}
Explanation of above function:
- The function “echo()” is defined with “void” return type.
- It has two arguments. One is a constant array variable with size of column and another one is size of the array.
- Using “for” loops, the array values are printed in screen.
Program with function definition:
/*Include the appropriate headers*/
#include<iostream>
using namespace std;
//Function definition
void echo(const int a[][5], int len)
{
//Print statement
cout<<"Array values:\n";
//Loop for rows
for (int i = 0; i <...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
How we can pass the function pointer as a parameter give example.
Find any errors in the following function declarations:
void sun(void, void);
float ACM (void); is non-void function with no parameters
Select one:
O True
O False
Chapter 7 Solutions
Problem Solving with C++ (10th Edition)
Ch. 7.1 - Prob. 1STECh. 7.1 - In the array declaration double score(5); state...Ch. 7.1 - Identity any errors in the following array...Ch. 7.1 - What is the output of the following code? char...Ch. 7.1 - What is the output of the following code? double a...Ch. 7.1 - What is the output of the following code? int i,...Ch. 7.1 - Prob. 7STECh. 7.1 - Suppose we expect the elements of the array a to...Ch. 7.1 - Prob. 9STECh. 7.1 - Suppose you have the following array declaration...
Ch. 7.2 - Consider the following function definition: void...Ch. 7.2 - Prob. 12STECh. 7.2 - Write a function definition for a function called...Ch. 7.2 - Consider the following function definition: void...Ch. 7.2 - Insert const before any of the following array...Ch. 7.2 - Write a function named outOfOrder that takes as...Ch. 7.3 - Write a program that will read up to ten...Ch. 7.3 - Write a program that will read up to ten letters...Ch. 7.3 - Following is the declaration for an alternative...Ch. 7.4 - Prob. 20STECh. 7.4 - Write code that will fill the array a (declared...Ch. 7.4 - Prob. 22STECh. 7 - Write a function named firstLast2 that takes as...Ch. 7 - Write a function named countNum2s that takes as...Ch. 7 - Write a function named swapFrontBack that takes as...Ch. 7 - The following code creates a small phone book. An...Ch. 7 - There are three versions of this project. Version...Ch. 7 - Hexadecimal numerals are integers written in base...Ch. 7 - Solution to Programming Project 7.3 Write a...Ch. 7 - Prob. 4PPCh. 7 - Write a program that reads in a list of integers...Ch. 7 - Prob. 6PPCh. 7 - An array can be used to store large integers one...Ch. 7 - Write a program that will read a line of text and...Ch. 7 - Write a program to score five-card poker hands...Ch. 7 - Write a program that will allow two users to play...Ch. 7 - Write a program to assign passengers seats in an...Ch. 7 - Prob. 12PPCh. 7 - The mathematician John Horton Conway invented the...Ch. 7 - Redo (or do for the first time) Programming...Ch. 7 - Redo (or do for the first time) Programming...Ch. 7 - A common memory matching game played by young...Ch. 7 - Your swim school has two swimming instructors,...Ch. 7 - Your swim school has two swimming instructors,...Ch. 7 - Prob. 19PPCh. 7 - The Social Security Administration maintains an...
Knowledge Booster
Similar questions
- Write a void function called 'qualify' that prints the message 'You qualify for the bonus' . Write the prototype for your function. Write a call for your functionarrow_forwardMark the following statements as true or false:arrow_forwardPlaying with Functions: Write a Javascript program to check if the argument passed to any function is a function type or not. I.e. Create a function check which takes a argument and check if that argument is a function or not.arrow_forward
- Create a program that accepts an integer N, and pass it to the function generatePattern. generatePattern() function which has the following description: Return type - void Parameter - integer n This function prints a right triangular pattern of letter 'T' based on the value of n. The top of the triangle starts with 1 and increments by one down on the next line until the integer n. For each row of in printing the right triangle, print "T" for n times. In the main function, call the generatePattern() function. Input 1. One line containing an integer Output Enter N: 4 T TT TTT TTTTarrow_forwardTrue or false? A call to a function with a void return type is always a statement itself, but a call to a value-returning function cannot be a statement by itself.arrow_forwardFor this question you will design and implement a function that takes 5 arguments and produces 5 return values. This question is internally recognized as variant 0371. The 5 arguments must be of types int, int, bool, bool, and char (in that order), and they must be stored in parameter variables 'ham', 'bar', 'xyz', 'gzk', and 'foo', respectively. As a clarifying example, the second parameter - 'bar' - will be of type int. The function you create must perform the operations list below, in the specified order and exactly as they are described here. You are NOT permitted to simplify the operations. 1. create a new variable named 'ukr' that stores the result of increasing the 'ham' parameter by 42 2. if the conjunction of the 'xyz' parameter and the 'gzk' parameter has a value of True, multiply the value of the 'ham' parameter by a value of 29 and store the result back in 'ham' 3. negate the value of the 'xyz' parameter and store the result back in 'xyz' 4. decrease the value of the 'ham'…arrow_forward
- Write the function prototype of the void function named G() that takes an int reference parameter and two char parameters respectively Your answerarrow_forwardUse Clojure to complete these questions: Please note that this is the beginning of the course so please use simple methods possible, thanks a. The built-in function type is useful for checking what kind of object an expression evaluates to. Write a function both-same-type? that takes two arguments, and returns true when they both have the same type, and false otherwise. b. Write a function list-longer-than? which takes two arguments: an integer n, and a list lst and returns true if lst has more than n elements, and false otherwise. For example, (list-longer-than? 3 '(1 2 3)) should return false, and (list-longer-than? 2 '(1 2 3)) should return true. c. See attached picture.arrow_forwardUSE C++programming languagearrow_forward
- PROGRAMMING LANGUAGE: C++arrow_forwardWrite a statement that declares a prototype for a function divide that takes four arguments and returns no value. The first two arguments are of type int. The last two arguments arguments are pointers to int that are set by the function to the quotient and remainder of dividing the first argument by the second argument. The function does not return a value.arrow_forwards buipeay E Write a well-documented C++ program consisting of the following two functions. • Write a function that accepts an array of integer elements and its size as parameters, swaps the two halves of the array as shown below. If the array contains the following: 5 9 2 12 8 4 Then your function should swap the two halves of the given array so as it becomes as follows: 12 8 4 5 9 • Write a function main () that defines an array consisting of 13 integer elements and performs the following tasks in order: • fills the array by prompting the user to enter 13 integer values from the keyboard; • displays all array elements separated by two spaces. • swaps the two halves of the array by calling the above-defined function. • displays all array elements (after swapping) separated by tabs. Hints 1. Develop and check the correctness of the tasks in a and b parts before starting parts c and d. 2. Try and practice the following program structures: o your program, write the main () function after…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
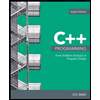
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
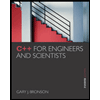
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr