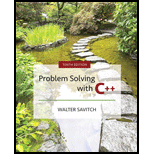
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 7.2, Problem 12STE
Program Plan Intro
Purpose of given code:
The given code is initializes the array “b[]” and passes each value by calling the function “tripler()” using “for” loop.
Given code:
/*Function definition with argument*/
void tripler(int& n)
{
/*Calculation of "n" value*/
n=3*n;
}
/*Declaration and initialization of variable*/
int b[5]={1,2,3,4,5};
//Error
for(int i=1;i<=5;i++)
/*Call the function with argument value*/
tripler(b[i]);
Explanation of given code:
- The code defines the function “tripler()” which takes the address of an argument.
- The value of passed by argument is multiplied by “3” and stored into the variable “n”.
- The array variable “b[]” was initialized in type of integer.
- Using “for” loop, call the function with each value of array.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
using r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.
using r language
using r language
Chapter 7 Solutions
Problem Solving with C++ (10th Edition)
Ch. 7.1 - Prob. 1STECh. 7.1 - In the array declaration double score(5); state...Ch. 7.1 - Identity any errors in the following array...Ch. 7.1 - What is the output of the following code? char...Ch. 7.1 - What is the output of the following code? double a...Ch. 7.1 - What is the output of the following code? int i,...Ch. 7.1 - Prob. 7STECh. 7.1 - Suppose we expect the elements of the array a to...Ch. 7.1 - Prob. 9STECh. 7.1 - Suppose you have the following array declaration...
Ch. 7.2 - Consider the following function definition: void...Ch. 7.2 - Prob. 12STECh. 7.2 - Write a function definition for a function called...Ch. 7.2 - Consider the following function definition: void...Ch. 7.2 - Insert const before any of the following array...Ch. 7.2 - Write a function named outOfOrder that takes as...Ch. 7.3 - Write a program that will read up to ten...Ch. 7.3 - Write a program that will read up to ten letters...Ch. 7.3 - Following is the declaration for an alternative...Ch. 7.4 - Prob. 20STECh. 7.4 - Write code that will fill the array a (declared...Ch. 7.4 - Prob. 22STECh. 7 - Write a function named firstLast2 that takes as...Ch. 7 - Write a function named countNum2s that takes as...Ch. 7 - Write a function named swapFrontBack that takes as...Ch. 7 - The following code creates a small phone book. An...Ch. 7 - There are three versions of this project. Version...Ch. 7 - Hexadecimal numerals are integers written in base...Ch. 7 - Solution to Programming Project 7.3 Write a...Ch. 7 - Prob. 4PPCh. 7 - Write a program that reads in a list of integers...Ch. 7 - Prob. 6PPCh. 7 - An array can be used to store large integers one...Ch. 7 - Write a program that will read a line of text and...Ch. 7 - Write a program to score five-card poker hands...Ch. 7 - Write a program that will allow two users to play...Ch. 7 - Write a program to assign passengers seats in an...Ch. 7 - Prob. 12PPCh. 7 - The mathematician John Horton Conway invented the...Ch. 7 - Redo (or do for the first time) Programming...Ch. 7 - Redo (or do for the first time) Programming...Ch. 7 - A common memory matching game played by young...Ch. 7 - Your swim school has two swimming instructors,...Ch. 7 - Your swim school has two swimming instructors,...Ch. 7 - Prob. 19PPCh. 7 - The Social Security Administration maintains an...
Knowledge Booster
Similar questions
- using r language Obtain a bootstrap t confidence interval estimate for the correlation statistic in Example 8.2 (law data in bootstrap).arrow_forwardusing r language Compute a jackknife estimate of the bias and the standard error of the correlation statistic in Example 8.2.arrow_forwardusing r languagearrow_forward
- using r languagearrow_forwardThe assignment here is to write an app using a database named CIT321 with a collection named students; we will provide a CSV file of the data. You need to use Vue.js to display 2 pages. You should know that this assignment is similar, all too similar in fact, to the cars4sale2 example in the lecture notes for Vue.js 2. You should study that program first. If you figure out cars4sale2, then program 6 will be extremely straightforward. It is not my intent do drop a ton of new material here in the last few days of class. The database contains 51 documents. The first rows of the CSV file look like this: sid last_name 1 Astaire first_name Humphrey CIT major hrs_attempted gpa_points 10 34 2 Bacall Katharine EET 40 128 3 Bergman Bette EET 42 97 4 Bogart Cary CIT 11 33 5 Brando James WEB 59 183 6 Cagney Marlon CIT 13 40 GPA is calculated as gpa_points divided by hrs_attempted. GPA points would have been arrived at by adding 4 points for each credit hour of A, 3 points for each credit hour of…arrow_forwardI need help to solve the following case, thank youarrow_forward
- You will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forwardShow all the workarrow_forwardConstruct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
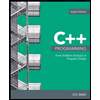
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
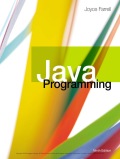
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
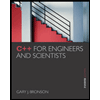
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
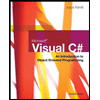
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,