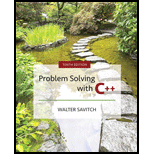
Write a function named outOfOrder that takes as Parameters an array of double s and an int parameter named size and returns a value of type int. This function will test this array for being out of order, meaning that the array violates the following condition:
a [0] <= a[1] <= a[2] <= ...
The function returns –1 if the elements are not out of order; otherwise. it will return the index of the first element of the array that is out of order. For example, consider the declaration
double a[10] = {1.2, 2.1, 3.3, 2.5, 4.5,
7.9, 5.4, 8.7, 9.9, 1.0);
In this array, a[2] and a[3] are the first pair out of order, and a[3] is the first element out of order, so the function returns 3. If the array were sorted, the function would return –1.

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Introduction to Programming Using Visual Basic (10th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Differential Equations: Computing and Modeling (5th Edition), Edwards, Penney & Calvis
C Programming Language
Starting Out with C++ from Control Structures to Objects (8th Edition)
Java: An Introduction to Problem Solving and Programming (7th Edition)
- in c++ In statistics, the mode of a set of values is the value that occurs most often or with the greatest frequency. Write a function that accepts as arguments the following: A) An array of integers B) An integer that indicates the number of elements in the array The function should determine the mode of the array. That is, it should determine which value in the array occurs most often. The mode is the value the function should return. If the array has no mode (none of the values occur more than once), the function should return −1. (Assume the array will always contain nonnegative values.) Demonstrate your pointer prowess by using pointer notation instead of array notation in this function.arrow_forwardin c++ language pleasssearrow_forwardFunctions with 2D Arrays in Java Write a function named displayElements that takes a two-dimensional array, the size of its rows and columns, then prints every element of a two-dimensional array. Separate every row by a new line and every column by a space. In the main function, call the displayElements function and pass in the required parameters. Output 1 2 3 4 5 6 7 8 9arrow_forward
- Create a function named "onlyOdd".The function should accept a parameter named "numberArray".Use the higher order function filter() to create a new array that only containsthe odd numbers. Return the new array.// Use this array to test your function:const testingArray = [1, 2, 4, 17, 19, 20, 21];arrow_forwardWrite a function name “computer Two” that accepts four parameters: A1: array of type integer A2: array of type integer S1: number of elements in A1 Array S2: number of elements in A2 Array The function must return true if the sum of A1 equals than the sum of A2 , otherwise it must return false .arrow_forwardIn java Develop a function that accepts an array and returns true if the array contains any duplicate values or false if none of the values are repeated. Develop a function that returns true if the elements are in decreasing order and false otherwise. A “peak” is a value in an array that is preceded and followed by a strictly lower value. For example, in the array {2, 12, 9, 8, 5, 7, 3, 9} the values 12 and 7 are peaks. Develop a function that returns the number of peaks in an array of integers. Note that the first element does not have a preceding element and the last element is not followed by anything, so neither the first nor last elements can be peaks. Develop a function that finds the starting index of the longest subsequence of values that is strictly increasing. For example, given the array {12, 3, 7, 5, 9, 8, 1, 4, 6}, the function would return 6, since the subsequence {1, 4, 6} is the longest that is strictly increasing. Develop a function that takes a string…arrow_forward
- When an array is passed as a parameter to a method, modifying the elements of the array from inside the function will result in a change to those array elements as seen after the method call is complete. O True O Falsearrow_forwardWrite a function named "SumPositive" that takes the following arguments: an array of integer values referred to as AArray; an integer Size that indicates the number of elements of AArray The function scans each position of the AArray[] and computes the sum of only the positive numbers. For example, if the array passed to the function looks like this: int AArray[] {1,-4,-2,2}; %3D SumPositive (AArray, 4); // the result returned is 3 int SumPositive(int AArray[], int Size) {arrow_forwardWrite a program that creates a 2D array of integers of size nXn initialized with test data.The program should have the following functions:● getTotal. This function should accept a 2D array as an argument and returnthe total of all the values in the array.● getAverage. This function should accept a 2D array as an argument and returnthe average of all the values in the array.● getRowTotal. This function should accept a 2D array as the first argument andan integer as its second argument. The second argument should be the subscriptof a row in the array. The function should return the total of the values in thespecified row.● getColumnTotal. This function should accept a 2D array as the first argumentand an integer as its second argument. The second argument should be thesubscript of a column in the array. The function should return the total of thevalues in the specified column.● getHighestInRow. This function should accept a 2D array as the firstargument and an integer as its second…arrow_forward
- In C++, define a “Conflict” function that accepts an array of Course object pointers. It will return two numbers:- the count of courses with conflicting days. If there is more than one course scheduled in the same day, it is considered a conflict. It will return if there is no conflict.- which day of the week has the most conflict. It will return 0 if there is none. Show how this function is being called and returning proper values. you may want to define a local integer array containing the count for each day of the week with the initial value of 0.Whenever you have a Course object with a specific day, you can increment that count for that corresponding index in the schedule array.arrow_forwardDefine a function named “getExamListInfo” that accepts an array of Exam objects and its size. It will return the following information to the caller: - The number of exams with perfect score - The number of exams with “Pass” status - The index of the Exam object in the array that has the largest score. For example, if you get the exam info for this array of Exam object Exam examList[] = { {"Midterm1 Exam", 90}, {"Midterm2 Exam", 80}, {"Final Exam", 50}, {"Extra Credit", 100}, {"Initial Test", 0}, {"Homework1", 69} } ; You will get Perfect Count: 1 Pass Count: 3 Index of the largest: 3 C++arrow_forwardin carrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
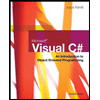