This program will store roster and rating information for a soccer team. Coaches rate players during tryouts to ensure a balanced team. (1) Prompt the user to input five pairs of numbers: A player's jersey number (0 - 99) and the player's rating (1 - 9). Store the jersey numbers in one int array and the ratings in another int array. Output these arrays (i.e., output the roster). Ex: Enter player 1's jersey number: 84 Enter player 1's rating: 7 Enter player 2's jersey number: 23 Enter player 2's rating: 4 Enter player 3's jersey number: 4 Enter player 3's rating: 5 Enter player 4's jersey number: 30 Enter player 4's rating: 2 Enter player 5's jersey number: 66 Enter player 5's rating: 9 ROSTER Player 1 -- Jersey number: 84,Rating: 7 Player 2 -- Jersey number: 23, Rating: 4 ... (2) Implement a menu of options for a user to modify the roster. Each option is represented by a single character. The program initially outputs the menu, and outputs the menu after a user chooses an option. The program ends when the user chooses the option to Quit. For this step, the other options do nothing. Ex: MENU u - Update player rating a - Output players above a rating r - Replace player o - Output roster q - Quit Choose an option: (3) Implement the "Output roster" menu option. Ex: ROSTER Player 1 -- Jersey number: 84, Rating: 7 Player 2 -- Jersey number: 23, Rating: 4 ... (4) Implement the "Update player rating" menu option. Prompt the user for a player's jersey number. Prompt again for a new rating for the player, and then change that player's rating. Ex: Enter a jersey number: 23 Enter a new rating for player: 6 ... (5) Implement the "Output players above a rating" menu option. Prompt the user for a rating. Print the jersey number and rating for all players with ratings above the entered value. Ex: Enter a rating: 5 ABOVE 5 Player 1 -- Jersey number: 84, Rating: 7 ... (6) Implement the "Replace player" menu option. Prompt the user for the jersey number of the player to replace. If the player is in the roster, then prompt again for a new jersey number and rating. Update the replaced player's jersey number and rating. Ex: Enter a jersey number: 4 Enter a new jersey number: 12 Enter a rating for the new player: 8
This program will store roster and rating information for a soccer team. Coaches rate players during tryouts to ensure a balanced team.
(1) Prompt the user to input five pairs of numbers: A player's jersey number (0 - 99) and the player's rating (1 - 9). Store the jersey numbers in one int array and the ratings in another int array. Output these arrays (i.e., output the roster).
Ex:
Enter player 1's jersey number:
84
Enter player 1's rating:
7
Enter player 2's jersey number:
23
Enter player 2's rating:
4
Enter player 3's jersey number:
4
Enter player 3's rating:
5
Enter player 4's jersey number:
30
Enter player 4's rating:
2
Enter player 5's jersey number:
66
Enter player 5's rating:
9
ROSTER
Player 1 -- Jersey number: 84,Rating: 7
Player 2 -- Jersey number: 23, Rating: 4 ...
(2) Implement a menu of options for a user to modify the roster. Each option is represented by a single character. The program initially outputs the menu, and outputs the menu after a user chooses an option. The program ends when the user chooses the option to Quit. For this step, the other options do nothing.
Ex:
MENU
u - Update player rating
a - Output players above a rating
r - Replace player
o - Output roster
q - Quit Choose an option:
(3) Implement the "Output roster" menu option.
Ex:
ROSTER
Player 1 -- Jersey number: 84, Rating: 7
Player 2 -- Jersey number: 23, Rating: 4 ...
(4) Implement the "Update player rating" menu option. Prompt the user for a player's jersey number. Prompt again for a new rating for the player, and then change that player's rating.
Ex:
Enter a jersey number:
23
Enter a new rating for player:
6
...
(5) Implement the "Output players above a rating" menu option. Prompt the user for a rating. Print the jersey number and rating for all players with ratings above the entered value.
Ex:
Enter a rating:
5
ABOVE 5
Player 1 -- Jersey number: 84, Rating: 7
...
(6) Implement the "Replace player" menu option. Prompt the user for the jersey number of the player to replace. If the player is in the roster, then prompt again for a new jersey number and rating. Update the replaced player's jersey number and rating.
Ex:
Enter a jersey number:
4
Enter a new jersey number:
12
Enter a rating for the new player:
8
![LAB
ACTIVITY
1 import java.util.Scanner;
3 public class PlayerRoster {
INM46809
2
5
7
9}
10
30.10.1: LAB*: Program: Soccer team roster
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
/* Type your code here. */
}
PlayerRoster.java](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9e6fa127-a003-469d-9a51-b1d4610918ed%2F4bdd6170-3f50-4c1a-b18a-5036fed244c0%2Fw726sz_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

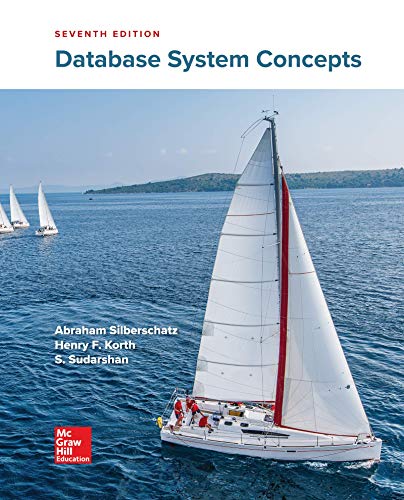
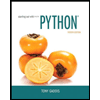
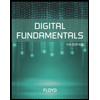
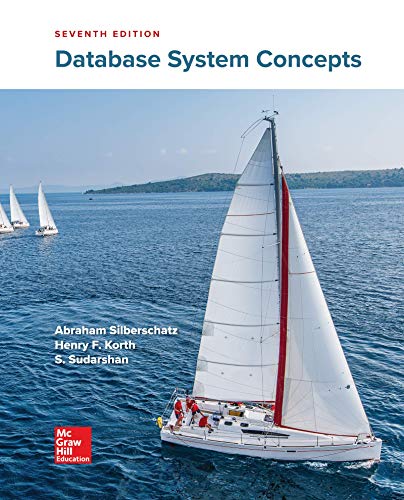
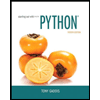
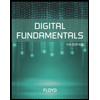
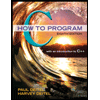
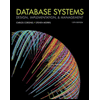
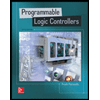