python: numpy def purchases(transactions): """ QUESTION 7 - A high-end store is trying to evaluate the total amount that customer's spend per transaction. They want customers to spend anywhere between $130 and $150 on average. - You need to determine whether the average number spent on each transaction per month is above, between, or below the desired amount. - Transactions is a numpy array containing a date, total amount earned each month, and total number of transactions each month. - Above: month's average amount spent per transaction > 150 - Within Range: 150 >= month's average amount spent per transaction >= 130 - Below: month's average amount spent per transaction < 130 - Return a numpy array with "Above", "Within Range" and "Below" for the average amount spent per transaction per month - THIS MUST BE DONE IN ONE LINE HINT: use np.where() and convert the type of each column to float Args: transactions (np.array) Returns: np.array >> transactions = np.array([['01-31-2022', 5462101, 24752], ['02-28-2022', 7081547.30, 34615], ['03-31-2022', 3287654.57, 16588], ['04-30-2022', 8725851.81, 45621], ['05-31-2022', 6730748.72, 26741], ['06-30-2022', 9562745.43, 76436], ['07-31-2022', 8641735.21, 61448], ['08-31-2022', 7641748.57, 52846], ['09-30-2022', 7645277.02, 65457], ['10-31-2022', 9416274.67, 65109], ['11-30-2022', 9841378.97, 57254], ['12-31-2022', 10654298.18, 98651]]) >> purchases(transactions) ['Above' 'Above' 'Above' 'Above' 'Above' 'Below' 'Within Range' 'Within Range' 'Below' 'Within Range' 'Above' 'Below'] """ # transactions = np.array([['01-31-2022', 5462101, 24752], # ['02-28-2022', 7081547.30, 34615], # ['03-31-2022', 3287654.57, 16588], # ['04-30-2022', 8725851.81, 45621], # ['05-31-2022', 6730748.72, 26741], # ['06-30-2022', 9562745.43, 76436], # ['07-31-2022', 8641735.21, 61448], # ['08-31-2022', 7641748.57, 52846], # ['09-30-2022', 7645277.02, 65457], # ['10-31-2022', 9416274.67, 65109], # ['11-30-2022', 9841378.97, 57254], # ['12-31-2022', 10654298.18, 98651]]) # print(purchases(transactions))
python: numpy
def purchases(transactions):
"""
QUESTION 7
- A high-end store is trying to evaluate the total amount that customer's spend per transaction. They
want customers to spend anywhere between $130 and $150 on average.
- You need to determine whether the average number spent on each transaction per month is above, between, or
below the desired amount.
- Transactions is a numpy array containing a date, total amount earned each month, and total
number of transactions each month.
- Above: month's average amount spent per transaction > 150
- Within Range: 150 >= month's average amount spent per transaction >= 130
- Below: month's average amount spent per transaction < 130
- Return a numpy array with "Above", "Within Range" and "Below" for the average amount spent per transaction per month
- THIS MUST BE DONE IN ONE LINE
HINT: use np.where() and convert the type of each column to float
Args:
transactions (np.array)
Returns:
np.array
>> transactions = np.array([['01-31-2022', 5462101, 24752],
['02-28-2022', 7081547.30, 34615],
['03-31-2022', 3287654.57, 16588],
['04-30-2022', 8725851.81, 45621],
['05-31-2022', 6730748.72, 26741],
['06-30-2022', 9562745.43, 76436],
['07-31-2022', 8641735.21, 61448],
['08-31-2022', 7641748.57, 52846],
['09-30-2022', 7645277.02, 65457],
['10-31-2022', 9416274.67, 65109],
['11-30-2022', 9841378.97, 57254],
['12-31-2022', 10654298.18, 98651]])
>> purchases(transactions)
['Above' 'Above' 'Above' 'Above' 'Above' 'Below' 'Within Range'
'Within Range' 'Below' 'Within Range' 'Above' 'Below']
"""
# transactions = np.array([['01-31-2022', 5462101, 24752],
# ['02-28-2022', 7081547.30, 34615],
# ['03-31-2022', 3287654.57, 16588],
# ['04-30-2022', 8725851.81, 45621],
# ['05-31-2022', 6730748.72, 26741],
# ['06-30-2022', 9562745.43, 76436],
# ['07-31-2022', 8641735.21, 61448],
# ['08-31-2022', 7641748.57, 52846],
# ['09-30-2022', 7645277.02, 65457],
# ['10-31-2022', 9416274.67, 65109],
# ['11-30-2022', 9841378.97, 57254],
# ['12-31-2022', 10654298.18, 98651]])
# print(purchases(transactions))

- Define a function "purchases" that takes in a numpy array "transactions" as input.
- Use np.where() to determine whether the average amount spent per transaction per month is "Above", "Within Range", or "Below".
- Convert the columns of the numpy array "transactions" to float using the astype() method.
- Calculate the average amount spent per transaction per month by dividing the total amount earned each month by the total number of transactions each month.
- Use np.where() to determine whether the average amount spent per transaction per month is "Above", "Within Range", or "Below" based on the desired range of $130 to $150.
- Return a numpy array with the results.
Step by step
Solved in 4 steps with 2 images

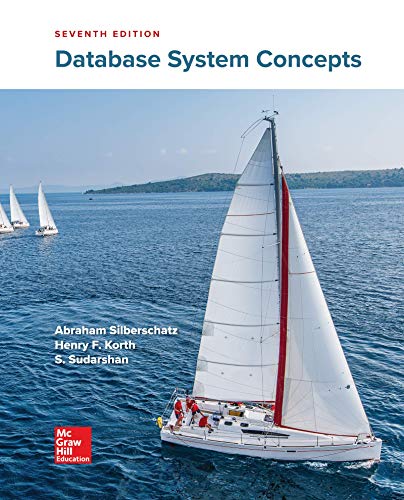
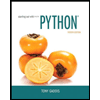
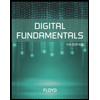
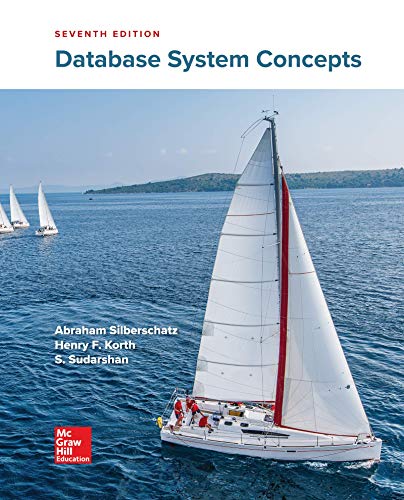
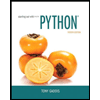
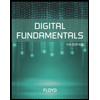
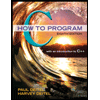
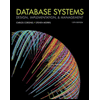
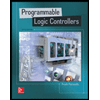