Lab 5 : Arrays Please the attachment which is been given to Develop a C code that asks for a class size (maximum can be 20). Then it asks the user to enter all the marks and saves them in an array. Then it generates a report about the class status that includes the following 1- Class average 2- Best mark and worst mark 3- number of failed students 4- Number of students with a grade of A and higher (80 or above) 5- number of students who got a mark above average
Lab 5 : Arrays
Please the attachment which is been given to Develop a C code that asks for a class size (maximum can be 20).
Then it asks the user to enter all the marks and saves them in an array.
Then it generates a report about the class status that includes the following
1- Class average
2- Best mark and worst mark
3- number of failed students
4- Number of students with a grade of A and higher (80 or above)
5- number of students who got a mark above average

- The program asks the user to input number of students in the class.
- To generate an array for number of students, malloc() is used.
- The user is then prompted to input marks for the students.
- To input marks for loop is used. Sum is calculated at the time of entry of marks.
- At the end of loop average is calculated.
- Another loop is used to calculate maximum marks, minimum marks, number of students who failed, number of students
with marks above 80 and number of students who scored above class average. - The passing limit is set to 33.
- The allocated memory is freed using free().
#include <stdio.h>
#include <stdlib.h>
int main()
{
int n, *marks, sum = 0;
int max, min;
float avg = 0;
int pass = 33;
int fail = 0;
int above_avg = 0;
int above_A = 0;
printf("Enter number of students: ");
scanf("%d", &n);
if (n > 20)
{
printf("Invalid input, exiting the program.");
exit(0);
}
marks = (int*) malloc(n * sizeof(int));
// if memory cannot be allocated
if(marks == NULL)
{
printf("Error! memory not allocated. Exiting program");
exit(0);
}
printf("Enter marks for %d students: \n", n);
for(int i = 0; i < n; ++i)
{
scanf("%d", marks + i);
sum += *(marks + i);
}
// Class average
avg = (float)sum / n;
max = min = marks[0];
/*
* Loop for finding maximum marks, minimum marks,
* number of students who failed, number of students
* with marks above 80 and number of students who scored
* above class average
*/
for (int i = 0; i < n; ++i)
{
if (max < marks[i])
max = marks[i];
if (min > marks[i])
min = marks[i];
if (marks[i] < pass)
fail += 1;
if (marks[i] > avg)
above_avg += 1;
if (marks[i] > 80)
above_A += 1;
}
printf ("Class Average: %f \n", avg);
printf ("Best marks: %d \n", max);
printf ("Worst marks: %d \n", min);
printf ("Number of students failed: %d \n", fail);
printf ("Number of students with marks above 80: %d \n", above_A);
printf ("Number of students above average: %d \n", above_avg);
free(marks);
return 0;
}
Step by step
Solved in 3 steps with 1 images

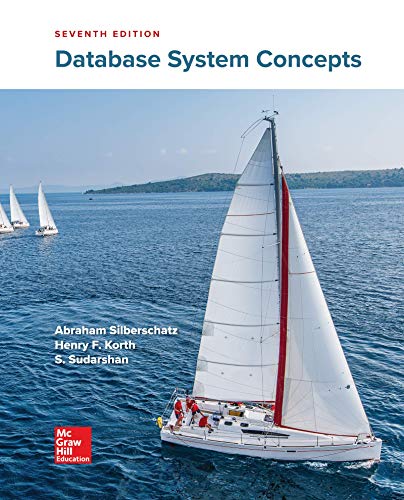
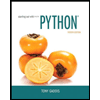
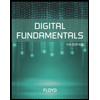
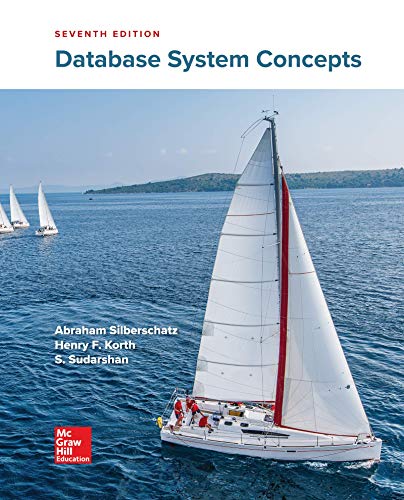
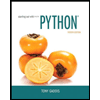
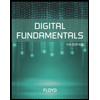
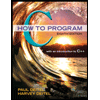
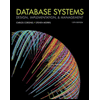
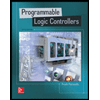