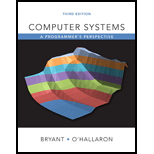
Given Information:
Three different functions are given:
// declare 2-D array
typedef int array_t[N][N];
// function to add values of 2-D array
int sumA(array_t a) {
// variable declaration
int i, j;
int sum = 0;
// traverse through rows
for (i = 0; i < N; i++)
// traverse through elements
for (j = 0; j < N; j++) {
// sum of elements
sum = sum + a[i][j];
}
// return sum
return sum;
}
// function to add values of 2-D array
int sumB(array_t a) {
// variable declaration
int i, j;
int sum = 0;
// traverse through columns
for (j = 0; j < N; j++)
// traverse through rows
for (i = 0; i < N; i++) {
// sum of elements
sum = sum + a[i][j];
}
// return sum
return sum;
}
// function to add values of 2-D array
int sumC(array_t a)
{
//variable declaration
int i,j;
int sum=0;
//traverse through columns
for(j=0;j<N;j+=2)
//traverse through rows
for(i=0;i<N;i+=2)
{
//sum of elements
sum+=(a[i][j]+a[i+1][j]+ a[i][j+1]+a[i+1][j+1]);
}
//return sum
return sum;
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
- instruction is in the first picture cacheSim.h #include<stdlib.h>#include<stdio.h>#define DRAM_SIZE 1048576typedef struct cb_struct {unsigned char data[16]; // One cache block is 16 bytes.u_int32_t tag;u_int32_t timeStamp; /// This is used to determine what to evict. You can update the timestamp using cycles.}cacheBlock;typedef struct access {int readWrite; // 0 for read, 1 for writeu_int32_t address;u_int32_t data; // If this is a read access, value here is 0}cacheAccess;// This is our dummy DRAM. You can initialize this in anyway you want to test.unsigned char * DRAM;cacheBlock L1_cache[2][2]; // Our 2-way, 64 byte cachecacheBlock L2_cache[4][4]; // Our 4-way, 256 byte cache// Trace points to a series of cache accesses.FILE *trace;long cycles;void init_DRAM();// This function print the content of the cache in the following format for an N-way cache with M Sets// Set 0 : CB1 | CB2 | CB 3 | ... | CB N// Set 1 : CB1 | CB2 | CB 3 | ... | CB N// ...// Set M-1 : CB1 | CB2 | CB…arrow_forwardDo pleasearrow_forward1)Encode the binary data 1010101010 into an even parity Hamming code. 2)A direct mapped cache consists of 256 slots. Main memory contains 64K blocks of 16 words each. Access time of the cache is 15 ns, and that for the main memory is 120 ns. Assume that main memory is accessed in parallel with cache look up. Initially, the cache is empty. (a) Give the format of the memory address. (b) Compute the effective access time, if suppose the hit ratio for read is 90%.arrow_forward
- Problem: A 1024 x 1024 array of 32-bit numbers is to be normalized as follows. For each column, the largest element is found and all elements of the column are divided by the value of this element. Assume that each page in the virtual memory consists of 4K bytes, and that 1M bytes of the main memory are allocated for storing array data during this computation. Assume that it takes 10 ms to load a page from the disk into the main memory when a page fault occurs. (a) Assume that the array is processed one column at a time. How many page faults would occur and how long does it take to complete the normalization process if the elements of the array are stored in column order in the virtual memory? (b) Repeat part (a) assuming the elements are stored in row order?arrow_forwardThe memory access time is I nanosecond for read operation with a hit in cache. * nanoseconds for a read operation with a miss in cache, 2 nanoseconds for a write operation with a hit in cache and 10 anoseconds for a write operation with a miss in cache. Execution of a sequence of instructions involves 100 instruction fetch operations, 60 memory operand read operations and 40 memory operand write operations. The cache hit-ratio is 0.9. The average memory access time (in nanoseconds) in executing the sequence of instructions isarrow_forwardQuiz-1: Refer to Lecture-3 Module 1 swap (int* V, int k) { temp = V[k]; /* temp in $t0 */ V[k] = V[k+1]; V[k+1] = temp; } Write the swap() function using special registers required for accessing parameters passed to this function. Use “t” registers for any needed temporary registers.arrow_forward
- 2-Caches are important to providing a high-performance memory hierarchy to processors. Below is a list of 32-bit memory address references, given as word addresses. a. For each of these references, identify the binary address, the tag, and the index given a direct-mapped cache with two-word blocks and a total size of 4 blocks. Also list if each reference is a hit or a miss, assuming the cache is initially empty. 42, 180, 46, 185, 189, 3, 181, 43, 6, 189, 65, 190 b. For each of these references, identify the binary address, the tag, and the index given a direct-mapped cache with four-word blocks and a total size of 4 blocks. Also list if each reference is a hit or a miss, assuming the cache is initially empty. c. For each of these references, identify the binary address, the tag, and the index given a two way associative cache with two-word blocks and a total size of 4 blocks. Also list if each reference is a hit or a miss, assuming the cache is initially empty d. For each of these…arrow_forwardCaches are important to providing a high-performance memory hierarchy toprocessors. Below is a list of 32-bit memory address references, given as word addresses.4, 180, 43, 2, 191, 88, 190, 14, 181, 44, 186, 253 a. For each of these references, identify the binary address, the tag, and the indexgiven a direct-mapped cache with 16 one-word blocks. Also list if each reference is a hitor a miss, assuming the cache is initially empty.b. For each of these references, identify the binary address, the tag, and the indexgiven a direct-mapped cache with two-word blocks and a total size of 8 blocks. Also listif each reference is a hit or a miss, assuming the cache is initially empty.arrow_forwardALSO: What is the hit ratio for the memory accesses (Round to the nearest percent.)arrow_forward
- c) On a machine with 32-bit words and 32-bit addresses, suppose the cache is an 16MB 8-way set-associative cache, with 8-word cache lines. Indicate how an address would be partitioned into fields to allow a single word to be fetched from the cache by the CPU (just indicate what the fields are, the order of the flelds in the address, and how each field is). Show many bits your work.arrow_forwardFor a direct-mapped cache design with 64-bit addresses, the following bits of the address are used to access the cache: Tag Index Offset 63-13 12-4 3-0 a. What is the cache block size (in bytes)?b. What is the cache size (in bytes)?c. What is the total number of bits (including valid bit, tag bits and data array bits) to implement this cache?d. For the same block and cache sizes, you want to implement a 4-way set-associative cache, what is the number of index bit and the number of tag bits?arrow_forward1. Write a program to store 5 consecutive bytes of data into the data segment. The first data element should be 1 and the data should be stored starting from offset position 10H. 2. Write program to exchange the contents of AL and BL using the stacks. The initial values of AL should have 4CH and BL should have 55H. 3. In the above programs, write the physical addresses of all the segment registers, as well as the logical addresses.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
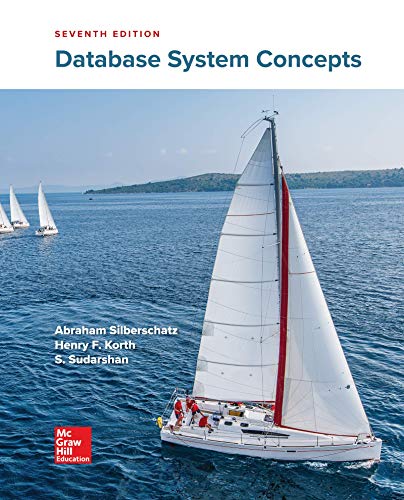
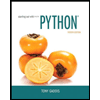
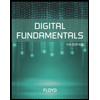
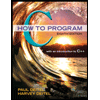
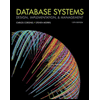
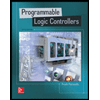