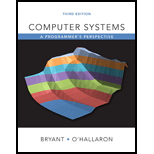
Concept explainers
A)
Given Information:
The given code is:
//variable declaration
//matrix
Int x[2][128];
int i;
//variable to store sum
Int sum=0;
//iterate through the matrix
for(i=0;i<128;i++)
{
//sum of matrix elements
sum+= x[0][i]*x[1][i];
}
Explanation:
- The given array is x[2][128]. The cache is initially empty and it begins to store data from “0x0” addresses.
- Here, size of (int) =4.
- Only memory accesses entries of array “x”.
- C arrays allocated in row-major order which means that each row is in their contiguous memory location.
B)
Given Information:
The given code is:
//variable declaration
//matrix
int x[2][128];
int i;
//variable to store sum
int sum=0;
//iterate through the matrix
for(i=0;i<128;i++)
{
//sum of matrix elements
sum+= x[0][i]*x[1][i];
}
Explanation:
- The given array is x[2][128]. The cache is initially empty and it begins to store data from “0x0” addresses.
- Here, size of (int) =4.
- Only memory accesses entries of array “x”.
- C arrays allocated in row-major order which means that each row is in their contiguous memory location.
C)
Given Information:
The given code is:
//variable declaration
//matrix
Int x[2][128];
int i;
//variable to store sum
Int sum=0;
//iterate through the matrix
for(i=0;i<128;i++)
{
//sum of matrix elements
sum+= x[0][i]*x[1][i];
}
Explanation:
- The given array is x[2][128]. The cache is initially empty and it begins to store data from “0x0” addresses.
- Here, size of (int) =4.
- Only memory accesses entries of array “x”.
- C arrays allocated in row-major order which means that each row is in their contiguous memory location.
D)
Given Information:
The given code is:
//variable declaration
//matrix
int x[2][128];
int i;
//variable to store sum
int sum=0;
//iterate through the matrix
for(i=0;i<128;i++)
{
//sum of matrix elements
sum+= x[0][i]*x[1][i];
}
- The given array is x[2][128]. The cache is initially empty and it begins to store data from “0x0” addresses.
- Here, size of (int) =4.
- Only memory accesses entries of array “x”.
- C arrays allocated in row-major order which means that each row is in their contiguous memory location.
E)
Given Information:
The given code is:
The given code is:
//variable declaration
//matrix
Int x[2][128];
int i;
//variable to store sum
Int sum=0;
//iterate through the matrix
for(i=0;i<128;i++)
{
//sum of matrix elements
sum+= x[0][i]*x[1][i];
}
Explanation:
- The given array is x[2][128]. The cache is initially empty and it begins to store data from “0x0” addresses.
- Here, size of (int) =4.
- Only memory accesses entries of array “x”.
- C arrays allocated in row-major order which means that each row is in their contiguous memory location.

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
- Quiz-1: Refer to Lecture-3 Module 1 swap (int* V, int k) { temp = V[k]; /* temp in $t0 */ V[k] = V[k+1]; V[k+1] = temp; } Write the swap() function using special registers required for accessing parameters passed to this function. Use “t” registers for any needed temporary registers.arrow_forwardwrite assembly x86-64 language File Types c, cpp, and asmarrow_forwardProgramming Language Pragmatics, 4th Editionarrow_forward
- subject: microprocessor and assembly language Data transmission systems and file subsystems often use a form of error detection that relies on calculating the parity (even or odd) of blocks of data. Your task is to create a procedure that returns True in the EAX register if the bytes in an array contain even parity, or False if the parity is odd. In other words, if you count all the bits in the entire array, their count will be even or odd. Preserve all other register values between calls to the procedure. Write a test program that calls your procedure twice, each time passing it a pointer to an array and the length of the array. The procedure’s return value in EAX should be 1 (True) or 0 (False). For test data, create two arrays containing at least 10 bytes, one having even parity, and another having odd parity.arrow_forwardLanguage: Java Write the function that produces the largest and smallest elements of an integer array sent to it, in accordance with themain function below. (Access to array elements should be done according to offset-address increment notation.)Sample Run:Biggest : 23Smallest : 0Sample Main................................/*prototip*/int main(){int array[5]={23, 4, 2, 0, 8};int biggest;int smallest;bigSmallFind(.............................)printf("Biggest : %d \n", biggest);printf("Smallest : %d \n", smallest);return 0;}arrow_forwardAssume that you have a A= 5x5 Matrix with one byte size elements.. Write an Assembly program that places its transpose A' just after A in 8086 physical memory and also places transpose of transpose (A) just after A' . The basic mechanism must use SI and DI registers.The whole codes must work, otherwise no points. WHEN YOU FINISHED THIS QUESTION ONLY, YOU HAVE TO SEND THE COPY OF THIS CODE to YOUR INSTRUCTOR as a TXT or WORD FILE THRU STIX AFTER EXAM ( IF YOU ARE SURE THE CODES ARE WORKING FINE!! OTHERWISE DON'T SEND !)arrow_forward
- Write a subroutine that finds the total number of elements in an array that are divisible by 8. The starting address of the array is passed in Z pointer and the array count is passed in r16. The count of elements that are divisible by 8 is returned in r22. Also write a test program to test this subroutine. The test program should define an array of 40 elements in program memory (immediately after the test program) to test this subroutine. use atmel studio pleasearrow_forwardsubject microprocessor and assembly language Create a procedure that fills an array of doublewords with N random integers, making sure the values fall within the range j...k, inclusive. When calling the procedure, pass a pointer to the array that will hold the data, pass N, and pass the values of j and k. Preserve all register values between calls to the procedure. Write a test program that calls the procedure twice, using different values for j and k. Verify your results using a debugger.arrow_forwardCache Memory Caches depend on the locality principle – if you access memory locations near to each other, then you will get better performance because the cache will pull in a bunch of nearby locations every time you access main memory. Assume that multi-dimensional arrays in C are stored in “row major order”, that is, the elements in each row are stored together Example: int test[3][5] = { {1, 2, 3, 4, 5}, {6, 7, 8, 9, 10}, {11, 12, 13, 14, 15} } Would be laid out in memory like: 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 Which of these two programs fragments (a or b) should have better cache performance? You need only answer “a” or “b” – no explanation needed. // begin fragment a int big[100,1000]; for (i=0, i<100, i++) { for (j=0, j<999, j++) { big[i,j] += big[i,j+1]; } } // end fragment a // begin fragment b int big[100,1000]; for (j=0, j<999, j++) { for (i=0, i<100, i++) { big[i,j] += big[i,j+1]; } } // end fragment barrow_forward
- Using C Language In this function, b has the address of a memory buffer that is num_bytes long. The function should repeatedly copy the 16 byte pattern that pattern16 points at into the memory buffer until num_bytes have been written. If num_bytes is not a multple of 16, the final write of the 16 byte pattern should be truncated to finish filling the buffer. void memset16(void *b, int num_bytes, void *pattern16) For example if the 16 bytes that pattern16 points at is 00 11 22 33 44 55 66 77 88 99 aa bb cc dd ee ff, then memset(b, 20, pattern16) should write to the buffer pointed at by p the 20 bytes 00 11 22 33 44 55 66 77 88 99 aa bb cc dd ee ff 00 11 22 33. Use SSE instructions to improve efficiency. Here's pseudocode. x = SSE unaligned load from pattern16while (num_bytes >= 16) SSE unaligned store x to p advance p by 16 bytes decrement num_bytes by 16while (num_bytes > 0) store 1 byte from pattern16 to p advance p by 1 byte advance pattern16 by 1 byte…arrow_forwardSystems Programming Purpose: The purpose of this assignment is to practice loop instructions and array iterations in M6800 assembly language programming. Write an assembly language program which implements the following C code that is a find minimum problem solution implementation: int A[10] = {8, 14, 6, 16, 5, 5, 10, 9, 4, 11}; int i = 0; int min = 255; // This is the largest number 8-bit accumulators can hold. while (i < 10) { if (A[i] < min) min = A[i]; } Your solution should be able to handle all possible array elements and orderings for any value an accumulator can hold. You should treat variable į as XR in assembler (index register) and store variable min in the address 60H. 0010: 00 00 00 00 00 00 00 00 0O 00 00 00 00 00 00 00 8628: 00 060 00 00 00 600 00 00 00 08 08 00 6 00 00 00 0030: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 0040: 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 0050: 00 G0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 006 0: 00 00 00 00 00 00 00 00 00 08 00…arrow_forwardProvide simple explanationarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
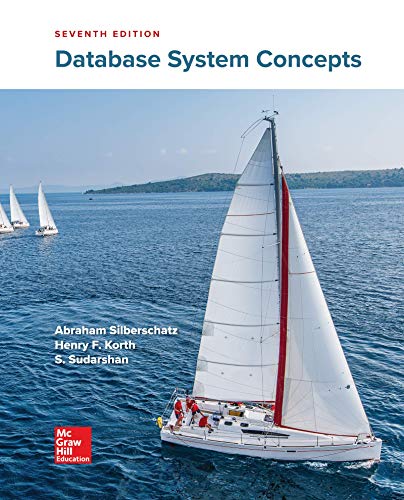
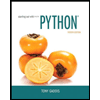
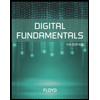
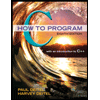
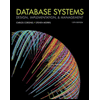
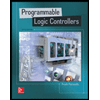