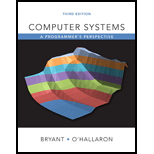
Computer Systems: A Programmer's Perspective (3rd Edition)
3rd Edition
ISBN: 9780134092669
Author: Bryant, Randal E. Bryant, David R. O'Hallaron, David R., Randal E.; O'Hallaron, Bryant/O'hallaron
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 6, Problem 6.35HW
Program Plan Intro
Given Information:
The code for matrix transpose routine is given:
//typedef 2-D array declaration
typedef int array[4][4];
//function to perform transpose of matrix
void transpose2(array dst, array src)
{
//variable declaration
int i,j;
//traverse through the rows
for(i=0;i<4;i++) {
//traverse through the elements
for(j=0;j<4;j++) {
//transpose of a matrix
dst[j][i]=src[i][j];
}
}
}
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
For a direct-mapped cache design with 64-bit addresses, the following bits of the address are used to access the cache:
Tag
Index
Offset
63-13
12-4
3-0
a. What is the cache block size (in bytes)?b. What is the cache size (in bytes)?c. What is the total number of bits (including valid bit, tag bits and data array bits) to implement this cache?d. For the same block and cache sizes, you want to implement a 4-way set-associative cache, what is the number of index bit and the number of tag bits?
3.
For a direct-mapped cache design with a 32-bit address, the following bits of the address are used to access the cache. We assume that each word has 4 bytes.
How many words of data are included in one cache line?
Chapter 6 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
Ch. 6.1 - Prob. 6.1PPCh. 6.1 - Prob. 6.2PPCh. 6.1 - Prob. 6.3PPCh. 6.1 - Prob. 6.4PPCh. 6.1 - Prob. 6.5PPCh. 6.1 - Prob. 6.6PPCh. 6.2 - Prob. 6.7PPCh. 6.2 - Prob. 6.8PPCh. 6.4 - Prob. 6.9PPCh. 6.4 - Prob. 6.10PP
Ch. 6.4 - Prob. 6.11PPCh. 6.4 - Prob. 6.12PPCh. 6.4 - Prob. 6.13PPCh. 6.4 - Prob. 6.14PPCh. 6.4 - Prob. 6.15PPCh. 6.4 - Prob. 6.16PPCh. 6.5 - Prob. 6.17PPCh. 6.5 - Prob. 6.18PPCh. 6.5 - Prob. 6.19PPCh. 6.5 - Prob. 6.20PPCh. 6.6 - Prob. 6.21PPCh. 6 - Prob. 6.22HWCh. 6 - Prob. 6.23HWCh. 6 - Suppose that a 2 MB file consisting of 512-byte...Ch. 6 - The following table gives the parameters for a...Ch. 6 - The following table gives the parameters for a...Ch. 6 - Prob. 6.27HWCh. 6 - This problem concerns the cache in Practice...Ch. 6 - Suppose we have a system with the following...Ch. 6 - Suppose we have a system with following...Ch. 6 - Suppose that a program using the cache in Problem...Ch. 6 - Repeat Problem 6.31 for memory address0x16E8 A....Ch. 6 - Prob. 6.33HWCh. 6 - Prob. 6.34HWCh. 6 - Prob. 6.35HWCh. 6 - Prob. 6.36HWCh. 6 - Prob. 6.37HWCh. 6 - Prob. 6.38HWCh. 6 - Prob. 6.39HWCh. 6 - Given the assumptions in Problem 6.38, determine...Ch. 6 - You are writing a new 3D game that you hope will...Ch. 6 - Prob. 6.42HWCh. 6 - Prob. 6.43HWCh. 6 - Prob. 6.45HWCh. 6 - Prob. 6.46HW
Knowledge Booster
Similar questions
- For a direct-mapped cache design with a 32-bit address, the following bits of the address are used to access the cache. a. What is the cache block size in words? b. How many entries does the cache have? Tag 31-13 Index 12-6 Offset 5-0arrow_forward6. For a direct mapped cache comprising 16 single word blocks answer the following questions. Assume address and word sizes are both 32 bits and that the memory is byte addressed (4 bytes per 32-bit word). Enter answers as numbers only. How many index bits are there? How many offset bits are there? How many tag bits are there?arrow_forwardFor a direct-mapped cache design with a 32-bit address, the following bits of the address are used to access the cache. We assume that each word has 4 bytes. How many entries does the cache have?arrow_forward
- 1. For a direct-mapped cache design with a 32-bit address, the following bits of address are used to access the cache. Tag Index Offset 31-14 13-7 6-0 a. What is the cache block size (in words)? b. How many entries does this cache have? c. What is the ratio between total bits required for such a cache implementation over the data storage bits?arrow_forward5.barrow_forwardIn a microprocessor of 32 bit addresses, the tag length will change if we design a two-way set-associate cache versus a four-way set-associative cache, with the same number of cache lines in each organization, and the same number of data bytes per set. a. True b. Falsearrow_forward
- For a direct-mapped cache design with a 32-bit address, the following bits of the address are used to access the cache. We assume that each word has 4 bytes. What is the ratio between total bits required for such a cache implementation over the data storage bits?arrow_forward3. The table below represents five lines from a cache that uses fully associative mapping with a block size of 8. Identify the address of the shaded data, 0xE6, first in binary and then in hexadecimal. The tag numbers and word id bits are in binary, but the content of the cache (the data) is in hexadecimal. Word id bits Tag 000 001 010 011 100 101 110 111 ------------------------------------------ 1011010 10 65 BA 0F C4 19 6E C3 1100101 21 76 CB 80 D5 2A 7F B5 0011011 32 87 DC 91 E6 3B F0 A6 1100000 43 98 ED A2 F7 4C E1 97 1111100 54 9A FE B3 08 5D D2 88arrow_forwardQuestion 4arrow_forward
- In a Direct Mapped Cache Memory Physical Address format the Cache line offset field size and word offset field size are same (with word size of one Byte). The number of tag bits in the Physical Address format is equal to the number of blocks in Cache Memory. If the Tag field Size is Mega words. 16 bits, the size of the physical Memory isarrow_forwardConsider a direct-mapped cache with 2 MB data 16-word block and 32-bit addressing. a Find the number of tag bits, index bits, and offset bits in a 32-bit that is byte addressed.arrow_forwardComputer Science Consider a direct-mapped cache with 8 lines, each holding 16 bytes of data. The cache is byte-addressable and the main memory consists of 64 KB, which is also byte-addressable. Assume that a program reads 16KB of memory sequentially. Answer the following questions:a) How many bits are required for the tag, index, and offset fields of a cache address?b) What is the cache size in bytes?c) What is the block size in bytes?d) What is the total number of blocks in main memory?e) How many cache hits and misses will occur for the program, assuming that the cache is initially empty?f) What is the hit ratio?g) Give an example virtual address (in BINARY) that will be placed in cache line 5.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
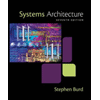
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning