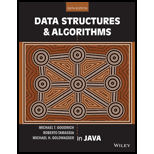
Explanation of Solution
Method to find the middle node of doubly linked list:
This method moves right from head is assigned to middle node and at the same time moves left from tail is assigned to partner node.
- If the middle and partner node is equal, then return the middle node.
- If middle node is just next node of partner node, then it even number of elements. So, return the previous node of middle node.
Program:
//Define the middle() method
private Node<E> middle()
{
//Check whether the list is empty
if (size == 0)
//Throw an exception
throw new IllegalStateException("List must not be empty");
//Assign the next node of head to middle Node
Node<E> middleNode = head−>next;
//Assign the previous node of tail to partner Node
Node<E> partnerNode = tail−>prev;
/*Loop executes until the middle node and partner node is not equal and next node of middle node and partner node is not equal. */
while (middleNode != partnerNode && middleNode−>next != partnerNode)
{
//Assign next node of middle node to middle node
middleNode = middleNode.getNext();
/*Assign previous node of partner node to partner node...

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Data Structures and Algorithms in Java
- Develop a procedure that will allow you to remove from a linked list all of the nodes that share a key?arrow_forwardFor linked lists, use a natural mergesort. Since it doesn't take up extra space and is certain to be linearithmic, this method is the one used to sort linked lists.For linked lists, use a natural mergesort. Since it doesn't take up extra space and is certain to be linearithmic, this method is the one used to sort linked lists.For linked lists, use a natural mergesort. Since it doesn't take up extra space and is certain to be linearithmic, this method is the one used to sort linked lists.For linked lists, use a natural mergesort. Since it doesn't take up extra space and is certain to be linearithmic, this method is the one used to sort linked lists.For linked lists, use a natural mergesort. Since it doesn't take up extra space and is certain to be linearithmic, this method is the one used to sort linked lists.For linked lists, use a natural mergesort. Since it doesn't take up extra space and is certain to be linearithmic, this method is the one used to sort linked lists.For linked…arrow_forwardJava Programming language Help please.arrow_forward
- How many references must you change to insert/delete a node in a single/double linked list? What are the time complexities of finding 10000th element from beginning of a linked list of size n? Understand the method such as AppentToHead, AppendToTail, Delete,...etc and be able to answer questions based on certain scenario?arrow_forwardThe strongest linkedlist is made up of an unknown number of nodes. Is there one in particular that stands out as being particularly lengthy?arrow_forwardThe strongest linkedlist is made up of an unknown number of nodes.Is there one in particular that stands out as being particularly lengthy?arrow_forward
- Java Programming language Please help me with this. Thanks in advance.arrow_forwardQuestion Given a singly linked list, you need to do two tasks. Swap the first node with the last node. Then count the number of nodes and if the number of nodes is an odd number then delete the first node, otherwise delete the last node of the linked list. For example, if the given linked list is 1->2->3->4->5 then the linked list should be modified to 2->3->4->1. Because 1 and 5 will be swapped and 5 will be deleted as the number of nodes in this linked list is 5 which is an odd number, that means the first node which contains 5 has been deleted. If the input linked list is NULL, then it should remain NULL. If the input linked list has 1 node, then this node should be deleted and a new head should be returned. Sample 1: Input: NULL output: NULL. Sample 2: Input: 1 output: NULL Sample 3: Input: 1->2 output: 2 Sample 4: Input: 1->2->3 output: 2->1 Sample 5: Input: 1->2->3->4 _output: 4->2->3 Sample 6: Input: 1->2->3->4->5->6 output: 6->2->3->4->5. Input: The function takes one argument…arrow_forwardDevelop a procedure for removing duplicates from a linked list by identifying and removing all occurrences of a given key.arrow_forward
- In a circular linked list, how can you determine if a given node is the last node in the list? Describe the approach you would take to solve this problem.arrow_forwardPlease give me correct solution and explain.arrow_forwardThe nodes of the strongest linkedlist are undetermined.Is one especially lengthy?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
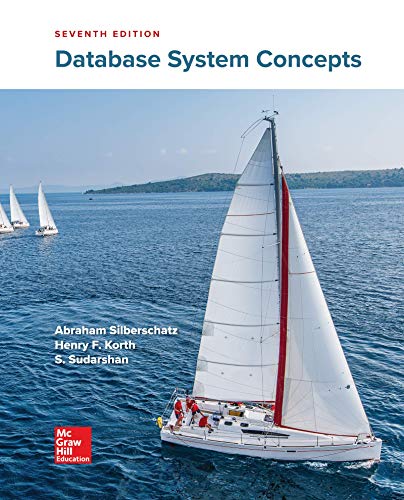
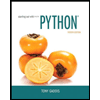
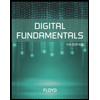
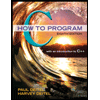
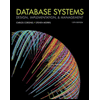
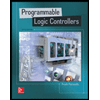