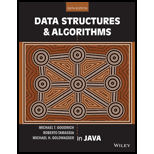
Input All Base Types
Program plan:
- Create a class InputBaseTypes to read different base types of input into standard output device.
- In main() function.
- Invoke the function inputAllBaseTypes() and write the different types of input to output device.
- In the method inputAllBaseTypes(),
- Read the different input values such as integer, decimal, big integer, big decimal, float, Boolean, long, and long integer from user and checks each base type of input value then it prints back to standard output device.
- Use the while loop and predefined function hasNextInt() to check whether the given input is integer or not..
- Use the while loop and predefined function hasNextBigDecimal() to check whether the given input is big decimal or not.
- Use the while loop and predefined function hasNextLong () to check whether the given input is long or not.
- Use the while loop and predefined function hasNextDouble() to check whether the given input is integer or not.
- Use the while loop and predefined function hasNextBoolean() to check whether the given input is Boolean or not.
- Use the while loop and predefined function hasNextBigInteger () to check whether the given input is big integer or not.
- Use the while loop and predefined function hasNextByte () to check whether the given input is byte or not.
- Use the while loop and predefined function hasNextFloat () to check whether the given input is float or not.
- In main() function.

Program to implement the method inputAllBaseTypes() that reads different base type input from input device and print the input back to standard output device.
Explanation of Solution
Program:
//Import the required java package
import java.util.Scanner;
Create a class InputBaseTypes to read different base types of input into standard output device.
//Main class definition
public class InputBaseTypes
{
The main() function prints all types of input into a standard output device.
//Main function
public static void main(String[] args)
{
//Display the header
System.out.println( "Basic input types." );
Invoke the function inputAllBaseTypes() and write the different types of input to output device.
//Call the function inputAllBaseTypes()
System.out.println(inputAllBaseTypes() );
}
Create the method inputAllBaseTypes() that reads the input value from user and checks each base type of input value then it prints back to standard output device.
//Create the method
public static int inputAllBaseTypes()
{
//Read the input from user
Scanner input = new Scanner(System.in);
Get the integer value from user.
//Read the integer value
System.out.print("Enter an integer: ");
Use the while loop and predefined function hasNextInt() to check whether the given input is integer or not. If not, skip the current input and display the error message. If the input is integer, then return back the input to display in standard output device.
/*Call the function hasNextInt() to check the given input is an integer*/
while( !input.hasNextInt() )
{
//Return the skipped input
input.nextLine();
//Display the error message
System.out.print("Not an integer, try again: ");
}
/*Display the entered big decimal into output device.*/
System.out.println( "You entered: " + input.nextInt() );
Get the big decimal from user.
//Read the integer value
System.out.print("Enter a BigDecimal: ");
Use the while loop and predefined function hasNextBigDecimal() to check whether the given input is big decimal or not. If not, skip the current input and display the error message. If the input is big decimal, then return back the input to display in standard output device.
/*Call the function hasNextBigDecimal () to check the given input is an big decimal*/
while( !input.hasNextBigDecimal() )
{
//Return the skipped input
input.nextLine();
//Display the error message
System.out.print("Not a BigDecimal, try again: ");
}
/*Display the entered big decimal into output device*/
System.out.println( "You entered: " + input.nextBigDecimal() );
Get the long value from user.
//Read the long value
System.out.println( "Enter a long: " );
Use the while loop and predefined function hasNextLong() to check whether the given input is long or not. If not, skip the current input and display the error message. If the input is long, then return back the input to display in standard output device.
/*Call the function hasNextLong() to check the given input is a long*/
while( !input.hasNextLong() )
{
//Return the skipped input
input.nextLine();
//Display the error message
System.out.println( "Not a long, try again" );
}
/*Display the entered long value into output device*/
System.out.println( "You entered: " + input.nextLong() );
Get the double value from user.
//Read the double value
System.out.println( "Enter a double: " );
Use the while loop and predefined function hasNextDouble() to check whether the given input is integer or not. If not, skip the current input and display the error message. If the input is integer, then return back the input to display in standard output device.
/*Call the function hasNextDouble() to check the given input is an double*/
while( !input.hasNextDouble() )
{
//Return the skipped input
input.nextLine();
//Display the error message
System.out.println( "Not a double, try again" );
}
/*Display the entered double value into output device.*/
System.out.println( "You entered: " + input.nextDouble() );
Get the Boolean value from user.
//Read the Boolean value
System.out.println( "Enter a boolean: " );
Use the while loop and predefined function hasNextBoolean() to check whether the given input is Boolean or not. If not, skip the current input and display the error message. If the input is Boolean, then return back the input to display in standard output device.
/*Call the function hasNextBoolean () to check the given input is an big decimal*/
while( !input.hasNextBoolean() )
{
//Return the skipped input
input.nextLine();
//Display the error message
System.out.println( "Not a boolean, try again" );
}
//Display the entered boolean into output device
System.out.println( "You entered: " + input.nextBoolean() );
Get the big integer value from user.
//Read the big integer value
System.out.println( "Enter a BigInteger: " );
Use the while loop and predefined function hasNextBigInteger() to check whether the given input is big integer or not. If not, skip the current input and display the error message. If the input is big integer, then return back the input to display in standard output device.
/*Call the function hasNextBigInteger() to check the given input is a long*/
while( !input.hasNextBigInteger() )
{
//Return the skipped input
input.nextLine();
//Display the error message
System.out.println( "Not a BigInteger, try again" );
}
/*Display the entered big integer into output device*/
System.out.println( "You entered: " + input.nextBigInteger() );
Get the input byte from user.
//Read the byte value
System.out.println( "Enter a Byte: " );
Use the while loop and predefined function hasNextByte () to check whether the given input is byte or not. If not, skip the current input and display the error message. If the input is byte, then return back the input to display in standard output device.
/*Call the function hasNextByte() to check the given input is a long*/
while( !input.hasNextByte() )
{
//Return the skipped input
input.nextLine();
//Display the error message
System.out.println( "Not a Byte, try again" );
}
//Display the entered byte into output device
System.out.println( "You entered: " + input.nextByte() );
Get the float value from user.
//Read the float value
System.out.println( "Enter a Float: " );
Use the while loop and predefined function hasNextFloat () to check whether the given input is float or not. If not, skip the current input and display the error message. If the input is float, then return back the input to display in standard output device.
/*Call the function hasNextFloat() to check the given input is a long*/
while( !input.hasNextFloat() )
{
//Return the skipped input
input.nextLine();
//Display the error message
System.out.println( "Not a Float, try again" );
}
//Display the entered float into output device
System.out.println( "You entered: " + input.nextFloat() );
//Return the value
return 0;
}
}
Output:
Basic input types.
Enter an integer: 1234
You entered: 1234
Enter a BigDecimal: 15
You entered: 15
Enter a long:
5
You entered: 5
Enter a double:
2
You entered: 2.0
Enter a boolean:
g
Not a boolean, try again
true
You entered: true
Enter a BigInteger:
89076
You entered: 89076
Enter a Byte:
u
Not a Byte, try again
8
You entered: 8
Enter a Float:
25
You entered: 25.0
0
Want to see more full solutions like this?
Chapter 1 Solutions
Data Structures and Algorithms in Java
- 1. Complete the routing table for R2 as per the table shown below when implementing RIP routing Protocol? (14 marks) 195.2.4.0 130.10.0.0 195.2.4.1 m1 130.10.0.2 mo R2 R3 130.10.0.1 195.2.5.1 195.2.5.0 195.2.5.2 195.2.6.1 195.2.6.0 m2 130.11.0.0 130.11.0.2 205.5.5.0 205.5.5.1 R4 130.11.0.1 205.5.6.1 205.5.6.0arrow_forwardAnalyze the charts and introduce each charts by describing each. Identify the patterns in the given data. And determine how are the data points are related. Refer to the raw data (table):arrow_forward3A) Generate a hash table for the following values: 11, 9, 6, 28, 19, 46, 34, 14. Assume the table size is 9 and the primary hash function is h(k) = k % 9. i) Hash table using quadratic probing ii) Hash table with a secondary hash function of h2(k) = 7- (k%7) 3B) Demonstrate with a suitable example, any three possible ways to remove the keys and yet maintaining the properties of a B-Tree. 3C) Differentiate between Greedy and Dynamic Programming.arrow_forward
- What are the charts (with their title name) that could be use to illustrate the data? Please give picture examples.arrow_forwardA design for a synchronous divide-by-six Gray counter isrequired which meets the following specification.The system has 2 inputs, PAUSE and SKIP:• While PAUSE and SKIP are not asserted (logic 0), thecounter continually loops through the Gray coded binarysequence {0002, 0012, 0112, 0102, 1102, 1112}.• If PAUSE is asserted (logic 1) when the counter is onnumber 0102, it stays here until it becomes unasserted (atwhich point it continues counting as before).• While SKIP is asserted (logic 1), the counter misses outodd numbers, i.e. it loops through the sequence {0002,0112, 1102}.The system has 4 outputs, BIT3, BIT2, BIT1, and WAITING:• BIT3, BIT2, and BIT1 are unconditional outputsrepresenting the current number, where BIT3 is the mostsignificant-bit and BIT1 is the least-significant-bit.• An active-high conditional output WAITING should beasserted (logic 1) whenever the counter is paused at 0102.(a) Draw an ASM chart for a synchronous system to providethe functionality described above.(b)…arrow_forwardS A B D FL I C J E G H T K L Figure 1: Search tree 1. Uninformed search algorithms (6 points) Based on the search tree in Figure 1, provide the trace to find a path from the start node S to a goal node T for the following three uninformed search algorithms. When a node has multiple successors, use the left-to-right convention. a. Depth first search (2 points) b. Breadth first search (2 points) c. Iterative deepening search (2 points)arrow_forward
- We want to get an idea of how many tickets we have and what our issues are. Print the ticket ID number, ticket description, ticket priority, ticket status, and, if the information is available, employee first name assigned to it for our records. Include all tickets regardless of whether they have been assigned to an employee or not. Sort it alphabetically by ticket status, and then numerically by ticket ID, with the lower ticket IDs on top.arrow_forwardFigure 1 shows an ASM chart representing the operation of a controller. Stateassignments for each state are indicated in square brackets for [Q1, Q0].Using the ASM design technique:(a) Produce a State Transition Table from the ASM Chart in Figure 1.(b) Extract minimised Boolean expressions from your state transition tablefor Q1, Q0, DISPATCH and REJECT. Show all your working.(c) Implement your design using AND/OR/NOT logic gates and risingedgetriggered D-type Flip Flops. Your answer should include a circuitschematic.arrow_forwardA controller is required for a home security alarm, providing the followingfunctionality. The alarm does nothing while it is disarmed (‘switched off’). It canbe armed (‘switched on’) by entering a PIN on the keypad. Whenever thealarm is armed, it can be disarmed by entering the PIN on the keypad.If motion is detected while the alarm is armed, the siren should sound AND asingle SMS message sent to the police to notify them. Further motion shouldnot result in more messages being sent. If the siren is sounding, it can only bedisarmed by entering the PIN on the keypad. Once the alarm is disarmed, asingle SMS should be sent to the police to notify them.Two (active-high) input signals are provided to the controller:MOTION: Asserted while motion is detected inside the home.PIN: Asserted for a single clock cycle whenever the PIN has beencorrectly entered on the keypad.The controller must provide two (active-high) outputs:SIREN: The siren sounds while this output is asserted.POLICE: One SMS…arrow_forward
- 4G+ Vo) % 1.1. LTE1 : Q B NIS شوز طبي ۱:۱۷ کا A X حاز هذا على إعجاب Mohamed Bashar. MEDICAL SHOE شوز طبي ممول . اقوى عرض بالعراق بلاش سعر القطعة ١٥ الف سعر القطعتين ٢٥ الف سعر 3 قطع ٣٥ الف القياسات : 40-41-42-43-44- افحص وكدر ثم ادفع خدمة التوصيل 5 الف لكافة محافظات العراق ופרסם BNI SH ופרסם DON JU WORLD DON JU MORISO DON JU إرسال رسالة III Messenger التواصل مع شوز طبي تعليق باسم اواب حمیدarrow_forwardA manipulator is identified by the following table of parameters and variables:a. Obtain the transformation matrices between adjacent coordinate frames and calculate the global transformation matrix.arrow_forwardWhich tool takes the 2 provided input datasets and produces the following output dataset? Input 1: Record First Last Output: 1 Enzo Cordova Record 2 Maggie Freelund Input 2: Record Frist Last MI ? First 1 Enzo Last MI Cordova [Null] 2 Maggie Freelund [Null] 3 Jason Wayans T. 4 Ruby Landry [Null] 1 Jason Wayans T. 5 Devonn Unger [Null] 2 Ruby Landry [Null] 6 Bradley Freelund [Null] 3 Devonn Unger [Null] 4 Bradley Freelund [Null] OA. Append Fields O B. Union OC. Join OD. Find Replace Clear selectionarrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
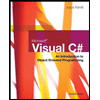
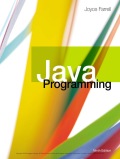
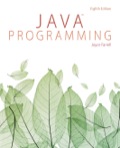
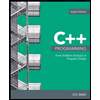
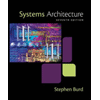