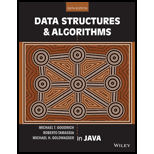
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781118771334
Author: Michael T. Goodrich
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Textbook Question
thumb_up100%
Chapter 1, Problem 28P
A common punishment for school children is to write out a sentence multiple times. Write a Java stand-alone program that will write out the following sentence one hundred times: “I will never spam my friends again.” Your program should number each of the sentences and it should make eight different random-looking typos.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
WRITE IN JAVA USING JOptionPane.showInputDialog
Write a program that reads a sentence as input and converts each word to “Pig Latin”. In one version of Pig Latin, you convert a word by removing the first letter, placing that letter at the end of the word, and then appending “ay” to the word. Here is an Example:
English: I SLEPT MOST OF THE NIGHT
Pig Latin: IAY LEPTSAY OSTMAY FOAY HETAY IGHTNAY
EXAMPLE:
Write a code that generates a random lowercase letter. In java programming.
Note: Not a string of letters just one letter is generated every time the program is run. meaning the output should not be something like this:
a
c
t
c
-that's a random string, it should only be one random letter generated randomly.
Write a Java program that reads two positive integers num1 and num2 (assume num1 < num2). Then, the program
will display all perfect numbers between num1 and num2. A positive integer is called a perfect number if it is equäl to
the sum of all of its positive divisors, excluding itself. For example, 6 is the first perfect number because 6 = 3 + 2 + 1.
The next is 28 = 14 + 7 + 4 + 2 + 1. Hint: use modulus (%) to find the divisor of a number.
Sample Input/Output
Enter two numbers: 6 1001
6 is a perfect number
28 is a perfect number
496 is a perfect number
Note: Your answer should follow the given sample input / output.
Chapter 1 Solutions
Data Structures and Algorithms in Java
Ch. 1 - Prob. 1RCh. 1 - Suppose that we create an array A of GameEntry...Ch. 1 - Write a short Java method, isMultiple, that takes...Ch. 1 - Write a short Java method, isEven, that takes an...Ch. 1 - Write a short Java method that takes an integer n...Ch. 1 - Write a short Java method that takes an integer n...Ch. 1 - Write a short Java method that takes an integer n...Ch. 1 - Write a short Java method that counts the number...Ch. 1 - Prob. 9RCh. 1 - Prob. 10R
Ch. 1 - Modify the CreditCard class from Code Fragment 1.5...Ch. 1 - Prob. 12RCh. 1 - Modify the declaration of the first for loop in...Ch. 1 - Prob. 14CCh. 1 - Write a pseudocode description of a method for...Ch. 1 - Write a short program that takes as input three...Ch. 1 - Write a short Java method that takes an array of...Ch. 1 - Prob. 18CCh. 1 - Write a Java program that can take a positive...Ch. 1 - Write a Java method that takes an array of float...Ch. 1 - Write a Java method that takes an array containing...Ch. 1 - Prob. 22CCh. 1 - Write a short Java program that takes two arrays a...Ch. 1 - Modify the CreditCard class from Code Fragment 1.5...Ch. 1 - Modify the CreditCard class to add a toString()...Ch. 1 - Write a short Java program that takes all the...Ch. 1 - Write a Java program that can simulate a simple...Ch. 1 - A common punishment for school children is to...Ch. 1 - The birthday paradox says that the probability...Ch. 1 - (For those who know Java graphical user interface...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Complete the following program so that it defines an array of 10 Yard objects. The program should use a loop to...
Starting Out with C++: Early Objects (9th Edition)
Describe the primary differences between the conceptual and logical data models.
Modern Database Management
a. Suppose you XOR the first 2 bits of a string of bits and then continue down the string by successively XORin...
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
What is the importance of modeling in engineering? How are the mathematical models for engineering processes pr...
HEAT+MASS TRANSFER:FUND.+APPL.
Account Balance A program that calculates the current balance in a savings account must ask the user for the fo...
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
When displaying a Java applet, the browser invokes the _____ to interpret the bytecode into the appropriate mac...
Web Development and Design Foundations with HTML5 (8th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a java programarrow_forwardWrite a Java program that asks the user to enter a string and counts how many times the letter "s" or "S" appears in the string. Display that number and then display the string that many times. Requirements: Call a method that gets the string from the user. Use recursion to ensure the string has at least 5 characters. Call a method that returns the number of times the letter "s" or "S" appears in the string. This method should use recursion. Call a method that takes the number of times the letter"s" or "S" appeared in the string and display the original string that was obtained from the user that many times using recursion. Call a method that sorts the letters in the string and searches for the letter "m". Display Found or Not Found.arrow_forwardWrite a JAVA program that checks phrases to determine if they are palindromes. A palindrome is a word, phrase, or sentence that reads left-to-right the same way it reads right-to-left, ignoring all punctuation and capitalization. For example, the statement “Madam, I’m Adam” is a palindrome as the response of “Eve.” Use a method to determine if a String is a palindrome. Test a single word non-palindrome, a single word palindrome, a phrase that is not a palindrome, and a phrase that is a palindrome. Print the phrase and whether it is a palindrome or not. Some simple palindromes are “racecar,” “taco cat,” and “A man, a plan, a canal, Panama!” There are a few different approaches for the test; choose one of your liking. Hint: extract all letters, change the remaining letters to lowercase, then test if the phrase is a palindrome.arrow_forward
- Write a Java program that asks a user to enter his/her age. If the user's age is in the range of [18, 150] (18 ≤ age ≤ 150), program prints “Old enough!”, when the given age is smaller than the given range it must print “Too young”, and otherwise prints "Incorrect age."arrow_forwardWrite a program in Javaarrow_forwardWrite a Java program using at least one for loop that prints pictures of piles of counters of different sizes using ‘=‘ characters as shown below. Each counter should be an odd number of characters wide and be two characters longer than the counter above it. The user should, when asked, type in the size of the top and bottom counters. For example, if the user enters 3 for the top counter and 7 for the bottom counter then the image below should be printed. your program must make use of a counter-controlled for loop be in procedural programming style (not OOP) your program should also be logically correct, solving the core problem, be elegantly written following good style, and be broken into appropriate methods that take arguments and/or return results.arrow_forward
- I need help with this Java problem as it's explained in the image below: Palindrome (Deque) A palindrome is a string that reads the same backwards and forwards. Use a deque to implement a program that tests whether a line of text is a palindrome. The program reads a line, then outputs whether the input is a palindrome or not. Ex: If the input is: senile felines! the output is: Yes, "senile felines!" is a palindrome. Ex: If the input is: rotostor the output is: No, "rotostor" is not a palindrome. Ignore punctuation and spacing. Assume all alphabetic characters will be lowercase. Special case: A one-character string is a palindrome. Hint: The deque must be a Deque of Characters, but ordinary chars will be automatically converted to Characters when added to the deque. Java Code: import java.util.Scanner; import java.util.LinkedList; import java.util.Deque; public class LabProgram { public static void main(String[] args) { Scanner scnr = new Scanner(System.in);…arrow_forwardI need help with this Java problem as it's explained in the image below: Palindrome (Deque) A palindrome is a string that reads the same backwards and forwards. Use a deque to implement a program that tests whether a line of text is a palindrome. The program reads a line, then outputs whether the input is a palindrome or not. Ex: If the input is: senile felines! the output is: Yes, "senile felines!" is a palindrome. Ex: If the input is: rotostor the output is: No, "rotostor" is not a palindrome. Ignore punctuation and spacing. Assume all alphabetic characters will be lowercase. Special case: A one-character string is a palindrome. Hint: The deque must be a Deque of Characters, but ordinary chars will be automatically converted to Characters when added to the deque. Java Code: import java.util.Scanner; import java.util.LinkedList; import java.util.Deque; public class LabProgram { public static void main(String[] args) { Scanner scnr = new…arrow_forwardWrite the complete java program.arrow_forward
- Write in Java and use JOptionPane.showInputDialog Write a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows. When the program begins, a random number is ranged of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, then the computer has chosen paper, If the number is 3, then the computer has chosen scissors. (Don’t display the computer’s choice yet.) The user enters his or her choice of “rock”, “paper”, or “scissors” at the key-board. (You can use a menu if you prefer.) The computer’s choice is displayed. A winner is selected according to the following rules: If one player chooses rock and the other player chooses scissors, then rock wins. (The rock smashes the scissors.) If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.) If one player chooses paper and the other player chooses rock, then paper wins.…arrow_forwardWrite an application that reads an integer value from the user and prints the sum ofall even integers between 2 and the input value, inclusive. Print an error message ifthe input value is less than 2. Use Java. Sample Run Examples: Example 1:the user enters 14Output: Sum: 56Example 2:the user enters 1Output: Error number must be greater than 1arrow_forwardJAVAarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
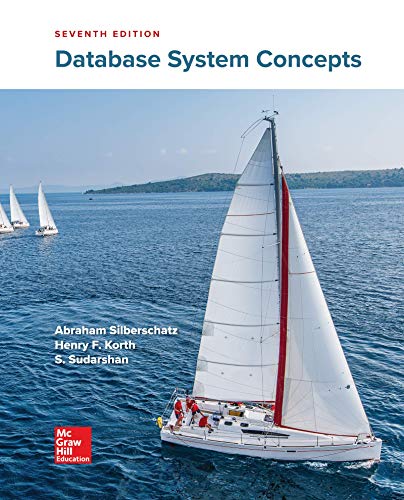
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
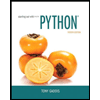
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
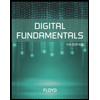
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
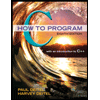
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
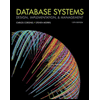
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
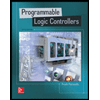
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Java random numbers; Author: Bro code;https://www.youtube.com/watch?v=VMZLPl16P5c;License: Standard YouTube License, CC-BY