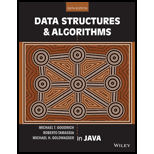
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781118771334
Author: Michael T. Goodrich
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Question
Chapter 1, Problem 12R
Program Plan Intro
Processing a negative payment amount
Program plan:
- Import the required packages.
- Create a class CreditCard to modify the class by ignoring any request to process the negative payment amount.
- Declare required variables.
- The parameterized constructor CreditCard() takes the input parameters of “cust”, “bk”, “acnt”, “lim”, and “initialBal” to initialize the values.
- The parameterized constructor CreditCard() takes the input parameters of “cust”, “bk”, “acnt”, and “lim” to initializes the values.
- The accessor method getCustomer() is to get the customer name.
- The accessor method getBank() is to get the bank name.
- The accessor method getAccount() is to get the account number.
- The accessor method getLimit() is to get the credit limit.
- The accessor method getBalance() is to get the credit balance.
- The method charge() is to update the balance.
- The method makePayment() is to make a payment when amount is not a negative payment.
- The utility method printSummary() is to display the credit card’s information.
- In main() function.
- Create an instance for CreditCard class.
- Initialize the value for credit card details by invoking the parameterized constructors using wallet object.
- Call the charge() method is to update the balance using wallet object for 3 arrays.
- Loop executes until the wallet[] object.
- Call the printsummary() method is to display the credit card details.
- Loop executes until the getBalance() method value is greater than 200. If yes,
- Call to makePayment() method to make a payment, then display the new balance.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
53. Write a class with two instance variables, representing an old password and
a new password. Write a recursive method that returns the number of places
where the two passwords have different characters. The passwords can have
different lengths. Write another, nonrecursive method returning whether the
two passwords are sufficiently different. The method takes an int parameter
indicating the minimum number of differences that qualify the passwords as
being sufficiently different. Your program should include a client class to test
your class.
function in Python called PassFail(), that returns the number of points required on the last exam in order to pass this class based on the first
four exam points and the 55% exam standard mentioned in the syllabus.
Note: there are a total of five exams. The first four exams are worth 100 points each.
The fifth and last exam is worth 200 points.
1. Implement the above system using inheritance in the best possible way. Keep every object size as small as possible. Implement all methods (setter/getter/constructors and destructors) Note that the region area is 0 while the city is len*width and the country is the sum of their cities. 2. Create array of countries called Arab of 22 countries, Write a function fill that fills the arrav Arab 3. Write a method that finds the city that has the max area in a country 4. Write a method that sorts the cities in a country from the largest to the smallest area 5. Write a function that retums array of countries of the same area of Arab 6. Write a function that compares between two countries. It returns true if countryl area greater than country2 area. 7. Write a function to move a city from one country to another.
Chapter 1 Solutions
Data Structures and Algorithms in Java
Ch. 1 - Prob. 1RCh. 1 - Suppose that we create an array A of GameEntry...Ch. 1 - Write a short Java method, isMultiple, that takes...Ch. 1 - Write a short Java method, isEven, that takes an...Ch. 1 - Write a short Java method that takes an integer n...Ch. 1 - Write a short Java method that takes an integer n...Ch. 1 - Write a short Java method that takes an integer n...Ch. 1 - Write a short Java method that counts the number...Ch. 1 - Prob. 9RCh. 1 - Prob. 10R
Ch. 1 - Modify the CreditCard class from Code Fragment 1.5...Ch. 1 - Prob. 12RCh. 1 - Modify the declaration of the first for loop in...Ch. 1 - Prob. 14CCh. 1 - Write a pseudocode description of a method for...Ch. 1 - Write a short program that takes as input three...Ch. 1 - Write a short Java method that takes an array of...Ch. 1 - Prob. 18CCh. 1 - Write a Java program that can take a positive...Ch. 1 - Write a Java method that takes an array of float...Ch. 1 - Write a Java method that takes an array containing...Ch. 1 - Prob. 22CCh. 1 - Write a short Java program that takes two arrays a...Ch. 1 - Modify the CreditCard class from Code Fragment 1.5...Ch. 1 - Modify the CreditCard class to add a toString()...Ch. 1 - Write a short Java program that takes all the...Ch. 1 - Write a Java program that can simulate a simple...Ch. 1 - A common punishment for school children is to...Ch. 1 - The birthday paradox says that the probability...Ch. 1 - (For those who know Java graphical user interface...
Knowledge Booster
Similar questions
- Write code for the InternetThing class to complete: a. A constructor that accepts a manufacturer and a serial number as its parameter. This constructor performs the following actions: i Store the serial number as a string ii Set a unique id number as an integer(hint- use the nextId). iii Set the IP address to the value “192.168.0.”+idnumber iv Set a local representation of a the power used in mW to the integer 1. v Set the password to “admin”. vi Prints the value “Created ”+ the result of the toString() method after assigning all instance data. vii Increment the number of things on record. b. Accessors for all private variables – Ensure the accessors for id, password and powerUse are named getId(), getPassword() and getPowerUse() c. A method setPassword that accepts a string argument and sets the instance password to the argument. All instance variables, except the password, should be private. The password should be protected. In class SmartHome, method addThing, • Create a…arrow_forwardJava Question.arrow_forwardUsing Python Languagearrow_forward
- *using java* Create a class AnimalCreate a class Cat, and a class Dog, and a class Bearded Dragon which extend Animal. Add to your Animal class: Member: name Methods: Public Animal(String name) //constructor public void makesSound()with the implementation printing out a generic animal sound. Next, override (add) the makesSound()method to your Cat and Dog class, with the implementation specific to each animal (i.e, cat says purr..). Do not override the makesSound() for your Dragon (bearded dragons don’t make sounds!)Note, you’ll also need a constructor in each of your subclasses which calls super(name) to initialize the common ‘name’ member of Animal. --- Next (in your test harness) create a List of different Animals ( a couple cats, a dog, a dragon... ) and add these Animals to your list. Iterate through your list & call makeSound on each. ( you should observe the implementation of the makeSound() method called will be: cat -> from Cat class, dog-> from Dog class,…arrow_forwardUsing JAVA write a code to: Implement a class Person with a name and a year of birth. Add a class Student that inherits from Person and has a major. Add a class Instructor that inherits from Person and has a salary. For each class, write the needed constructors, getters, setters, and a toString method that stringifies the instance variables in a readable format. Supply a test program that instantiates an instance of each class and exercises each method defined for the class.arrow_forward1. Suppose you are a student of East West University. You have to design a multithreadedsystem in java for your CSE110 course. So, you are assigned a job with followingdescriptions. You have to design a class NumberComputator which has a functionprintComposite(int n) that can take a parameter N and print the composite numberfrom 1 to nth term. Composite numbers are the number that are not prime number.Again, create two thread class (ThreadOne, ThreadTwo) by extending the Threadclass so that all of the thread should call the method “printComposite(int n)” of theNumberComputator class. Again, write a main java class named“MultiProgrammingDemo” with a main method that instantiate two objects of twothreads(ThreadOne, ThreadTwo).Your task is to process and run two threads simultaneously in the main method byconsidering the fact that when one thread printing with the printComposite(n)function the other threads must be wait for the completion of the work the initial thread.Design a JAVA…arrow_forward
- Write a simple C++ code as I am a beginner.arrow_forwardModify the above code to use the alternative method for multithreading (i.e., implementing the Runnable interface). Name your main class RunnableHelloBye and your subsidiary classes Hello and Goodbye respectively. The fi rst should display the message ‘Hello!’ ten times (with a random delay of 0–2 s between consecutive displays), while the second should do the same with the message ‘Goodbye!’. Note that it will NOT be the main class that implements the Runnable interface, but each of the two subsidiary classes.arrow_forwardUOWD Library is asking you to write a Java program that manages all the items in the Library. The library has books, journals, and media (DVD for example). All items have a name, author(s), and year of publication. A journal also has a volume number, while a media has a type (audio/video/interactive). The user of your program should be able to add an item, delete an item, change information of an item, list all items in a specific category (book, journal, or media), and print all items (from all categories). A menu asks the user which operation s/he wants to perform. Important: make use of collections, inheritance, interfaces, and exception handling wherever appropriate.arrow_forward
- Implement the below-given scenario using a Circular Link List of data structure in C++ Programming. Use classes and apply the encapsulation rules also. In an online shopping cart, the system must maintain a list of items and must calculate the total bill by adding the amount of all the items in the cart. Do Following: First create a class Item having id, name, price, and quantity provide appropriate methods and then Create Cart/List class which holds an items object to represent total items in the cart and next pointer Implement the method to add items in the array, remove an item and display all items. Now in the main do the following Insert Items in the list Display all items. Traverse the link list so that each item's bill gets calculated (by multiplying quantity with price) and also calculate and display the Total bill in the end. Delete an item if the user wants to remove an item from the cart.arrow_forward3.25 Fixed Sized Deque Your task is to create an implementation of the Java Deque interface that can only hold N items where N is a number passed into the constructor. Note that most (if not all) of the unit tests rely on the method object[] toArray() inherited from Collection. Therefore, you must make sure you implement that method correctly in order to get most of the points. 301664.1524810.gpazay7 LAB АCTIVITY 3.25.1: Fixed Sized Deque 0/ 70 Submission Instructions Compile command javac FixedsizedDeque.java -xlint:all -encoding utf-8 We will use this command to compile your code Upload your files below by dragging and dropping into the area or choosing a file on your hard drive. FixedSizedDeque.java Drag file here or Choose on hard drive.arrow_forward5. Create an interface MessageDecoder that has a single abstract method decode (cipherText), where cipher Text is the message to be decoded. The method will return the decoded message. Modify the classes 8.5 Graphics Supplement SubstitutionCipher and ShuffleCipher, as described in Exercises 16 and 17, so that they implement Message Decoder as well as the interface MessageEncoder that Exercise 15 describes. Finally, write a program that allows a user to encode and decode messages entered on the keyboard. 703arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
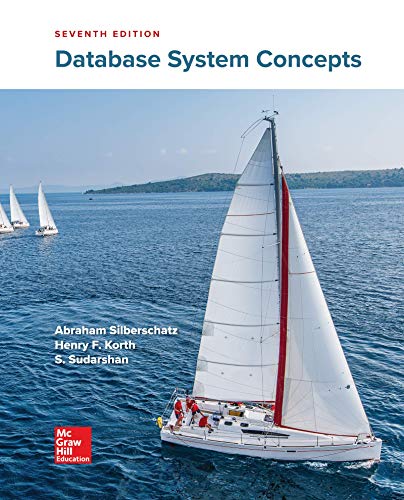
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
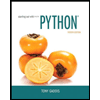
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
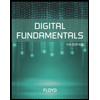
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
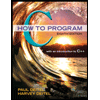
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
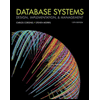
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
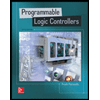
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education