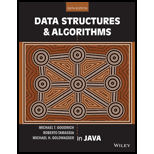
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781118771334
Author: Michael T. Goodrich
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Question
Chapter 1, Problem 10R
Program Plan Intro
Flowers
Program plan:
- Create a class Flower to display the name, number of petals and price of flower.
- Declare the required variables.
- In main() function,
- Create the objects for the class.
- Use the instance flower to set and return the name, petals and price values of flower.
- Call the function display() using the instance flower and display the flower name, pedals and price.
- Create the default constructor with no arguments.
- Create the parameterized constructor with the arguments “n”, “p”, and “d” to assign the name, pedals and price value respectively.
- In the method setName(),
- It passes the argument “n” to set the name of the flower.
- In the method setPetals(),
- It passes the argument “p” to set the number of petals in the flower.
- In the method setPrice(),
- It passes the argument “p” to set the price of the flower.
- In the method getName(),
- Return the name of the flower.
- In the method getPetals(),
- Return the petals of the flower.
- In the method getPrice(),
- Return the price of the flower.
- In the method display (),
- Print the details of the flower.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write a class in Java , named Patient that has fields for the following data: First name, middle name, and last name Address, city, state, and ZIP code Phone number Name and phone number of emergency contact The patient class should have a constructor, accessor, and mutator that accept an argument for each field.
in java A class called Author (as shown in the class diagram) is designed to model a book's author. It contains:
Three private instance variables: name (String), email (String), and gender (char of either 'm' or 'f');
One constructor to initialize the name, email and gender with the given values;
public Author (String name, String email, char gender) {......}
(There is no default constructor for Author, as there are no defaults for name, email and gender.)
public getters/setters: getName(), getEmail(), setEmail(), and getGender();(There are no setters for name and gender, as these attributes cannot be changed.)
A toString() method that returns string representation of author.
A class called Book is designed (as shown in the class diagram) to model a book written by one author. It contains:
Four private instance variables: name (String), author (of the class Author you have just created, assume that a book has one and only one author), price (double), and qty (int);
Two…
Design a class named Person with properties for holding a person’s name, address, and telephone number. Next, design a class named Customer, which is derived from the Person class. The Customer class should have a property for a customer number and a Boolean property indicating whether the customer wishes to be on a mailing list. Demonstrate an object of the Customer class in a simple application.
Chapter 1 Solutions
Data Structures and Algorithms in Java
Ch. 1 - Prob. 1RCh. 1 - Suppose that we create an array A of GameEntry...Ch. 1 - Write a short Java method, isMultiple, that takes...Ch. 1 - Write a short Java method, isEven, that takes an...Ch. 1 - Write a short Java method that takes an integer n...Ch. 1 - Write a short Java method that takes an integer n...Ch. 1 - Write a short Java method that takes an integer n...Ch. 1 - Write a short Java method that counts the number...Ch. 1 - Prob. 9RCh. 1 - Prob. 10R
Ch. 1 - Modify the CreditCard class from Code Fragment 1.5...Ch. 1 - Prob. 12RCh. 1 - Modify the declaration of the first for loop in...Ch. 1 - Prob. 14CCh. 1 - Write a pseudocode description of a method for...Ch. 1 - Write a short program that takes as input three...Ch. 1 - Write a short Java method that takes an array of...Ch. 1 - Prob. 18CCh. 1 - Write a Java program that can take a positive...Ch. 1 - Write a Java method that takes an array of float...Ch. 1 - Write a Java method that takes an array containing...Ch. 1 - Prob. 22CCh. 1 - Write a short Java program that takes two arrays a...Ch. 1 - Modify the CreditCard class from Code Fragment 1.5...Ch. 1 - Modify the CreditCard class to add a toString()...Ch. 1 - Write a short Java program that takes all the...Ch. 1 - Write a Java program that can simulate a simple...Ch. 1 - A common punishment for school children is to...Ch. 1 - The birthday paradox says that the probability...Ch. 1 - (For those who know Java graphical user interface...
Knowledge Booster
Similar questions
- Writing a java program that contains a class representing the vehicle And it contains basic specifications such as safe speed It is pre-installed and another variable for velocity is an amount The speed of the car and can be increased or decreased, and others Other specifications as desired by the student, as well L-car functions such as movement, stop and reverse, among others Of the jobs, according to the request of the student, so that the car is built By moving at a certain speed and when increasing and equaling the speed For safe speed, it will alert you when the speed is exceeded Safety the car stops permanently and speeds up Become zero. Note: Not all concepts are used In object-oriented programmingarrow_forwardNote: Write a Java program given below Question # 1: Define a class for Students with field name, age, marks. Define getter and setter for all the fields. In the setter method, if age is less than 0, print that Age is less than zero. Similarly, if marks is less than zero or greater than 100, print in correct marks and don’t assign marks to member variable. In the Test class, create student’s object and assign the marks and age to appropriate values. Try assigning incorrect values to check if getter and setter methods work as expected.arrow_forwardJava Programming problem Create a checking account class with three attributes: account number, owner’s name, and balance. Create constructor, getters and setters. If one is to set the initial balance of an account as a negative value in a setter or a constructor, remind the user about the error and set it as 0. Create a CheckingAccountDemo class, and create a main method in which Ask for the name, account number, and initial balance for one account from the keyboard input. With these input values as arguments in a call to the constructor, create a CheckingAccount object. Print the summary of this account that includes information on the name, account number, and balance by calling the getters.arrow_forward
- We have a need to develop an Object-Oriented Parking System. Attached below are all the classes in our system, but we only need a parking office class for this problem. Use the class diagrams and the full specification below to create a Parking Office class for an object-oriented parking system. Write your code using java. Full specification We have a need to develop an Object-Oriented Parking System. The system has several parking lots and the parking fees are different for each parking lot. Some parking lots scan permits only on entry. Charge is on entry, plus daily charges if car is parked overnight. Some parking lots scan permits on entry and exit, and charge is based on total hours parked, upon exit. Customers must have registered with the parking office in order to use any parking lot. Customers can use any parking lot. Each parking transaction will incur a charge to their account. Customers can have more than one car, and so they may request more than one parking permit for…arrow_forwardWrite a java program to create a class Employee. The class should include threeinstance variables: name (type: String), ID (type: int), and position (type: String).Write a class TestEmployee to test the class Employee. Read the name, ID andposition of three employees from screen input, create three employee objects usingthe constructor, and display each employee’s name, ID and position.arrow_forwardJava program: 1. Write a PaypalAccount class to include both balance and accountID as the instance variables. Make sure each instance of this account will have a unique accountID. In other words, different account object should have different accountID (hint: class variable). 2. Write a Bank class with main method. In the main method, ask the user to input how many accounts (say numOfAccount) to be generated in the bank (assuming less than 1000). Then create an array to hold these numOfAccount of Account objects. For eachAccount object, generate a random balance in the range of 0.0-1000.0. Assume that your campus ID is 141-88-2014; search the array to see if there is an account with accountID as 141 (the first three digits of your campus ID). If there is not an account with accountID as 141, then set the accountID of the last account in the array as 141; transfer all the balance of the first account to the account with accountID of 141. Set the balance of the account with accountID…arrow_forward
- Java program: 1. Write a PaypalAccount class to include both balance and accountID as the instance variables. Make sure each instance of this account will have a unique accountID. In other words, different account object should have different accountID (hint: class variable). 2. Write a Bank class with main method. In the main method, ask the user to input how many accounts (say numOfAccount) to be generated in the bank (assuming less than 1000). Then create an array to hold these numOfAccount of Account objects. For eachAccount object, generate a random balance in the range of 0.0-1000.0. Assume that your campus ID is abc-de-fghi; search the array to see if there is an account with accountID as abc (the first three digits of your campus ID). If there is not an account with accountID as abc, then set the accountID of the last account in the array as abc; transfer all the balance of the first account to the account with accountID of abc. Set the balance of the account with accountID…arrow_forwardNote: Write a java programarrow_forwardJava program: 1. Write a class Point. Points are described as having an (x,y) location. Instance variables should be private. Your class should contain a constructor that takes two integer values to set the point, accessor and mutator methods for the private instance variables and a toString method that prints the coordinates of the point. 2. Write a class Circle. Since circles are described by a centerpoint and a radius, the Circle class should extend the Point class. You need to add a private double for the radius. You should include a constructor, an accessor and a mutator method for the radius. Negative radii are not permitted (check and print an error message in the mutator). You should override the toString method to print the coordinates of the point, the radius, and the area of the circle. 3. Write a class Cylinder. Since cylinders are circles with heights, the Cylinder class should extend the Circle class. Add the appropriate instance variable and include a constructor, an…arrow_forward
- Write a JAVA PROGRAM that a class has an integer data member "i" and a method named "printNum" to print the value of 'i'. Its subclass also has an integer data member 'j'and a method named 'printNum'to print the value of 'j'. Make an object of the subclass and use it to assign a value to 'i'and to 'j'. Now call the method 'printNum'by this object.arrow_forwardJava- Suppose that Vehicle is a class and Car is a new class that extends Vehicle. Write a description of which kind of assignments are permitted between Car and Vehicle variables.arrow_forwardIn the class diagram below we have a parking charge class for an object-oriented parking system that is to be designed using java. Briefly explain any implementation decisions and the reasoning behind those without writing the complete code. N.B explain how the implementation will proceed instead of writing codearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
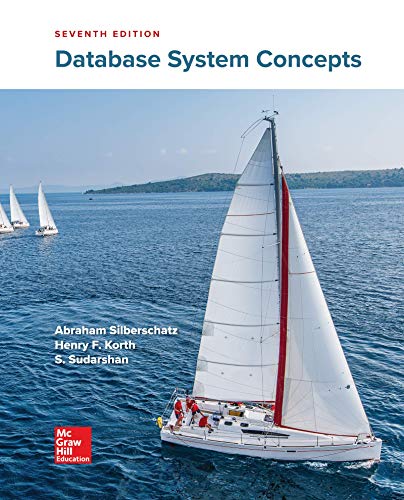
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
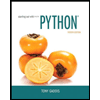
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
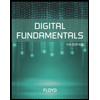
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
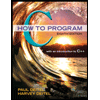
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
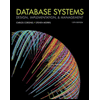
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
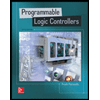
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education