The assignment here is to write an app using a database named CIT321 with a collection named students; we will provide a CSV file of the data. You need to use Vue.js to display 2 pages. You should know that this assignment is similar, all too similar in fact, to the cars4sale2 example in the lecture notes for Vue.js 2. You should study that program first. If you figure out cars4sale2, then program 6 will be extremely straightforward. It is not my intent do drop a ton of new material here in the last few days of class. The database contains 51 documents. The first rows of the CSV file look like this: sid last_name 1 Astaire first_name Humphrey CIT major hrs_attempted gpa_points 10 34 2 Bacall Katharine EET 40 128 3 Bergman Bette EET 42 97 4 Bogart Cary CIT 11 33 5 Brando James WEB 59 183 6 Cagney Marlon CIT 13 40 GPA is calculated as gpa_points divided by hrs_attempted. GPA points would have been arrived at by adding 4 points for each credit hour of A, 3 points for each credit hour of B, and so on. Humphrey Astaire would have a gpa of 34/10 = 3.4. The CSV file will be on the course website. It is named CIT321.csv. Here is what your model should look like. The model is named Student.js var mongoose = require('mongoose'); mongoose.connect ('mongodb://127.0.0.1:27017/CIT321'); var studentSchema = new mongoose. Schema ({ }); sid: {type: Number, required: true, unique: true}, last_name: {type: String, required: true}, first_name: {type: String, required: true}, major: {type: String, required: true}, hrs_attempted: {type: Number, required: true}, gpa_points: {type: Number, required: true} module.exports = mongoose.model ('Student', studentSchema); Here's what the pages should look like, more or less. You have 2 pages, index.html and edit.html in the public folder. The navbar has links named Home and Edit Student Data. Home opens the index.html page that has a drop-down list that will allow us to filter the students by major Our Website Home Edit Student Data CIT 321 Students Select a Major Here are the requirements. Use Vue.js and Bootstrap. Your pages do not need to look exactly like mine, but they need to convey the same information. • Your database and collection, along with your model, must be compatible with mine. The index page should allow filtering all students by major and displaying their GPA • • • The Edit Student data pages should allow displaying each document one at a time, allow editing major, gpa points and hrs attempted. You should be able to delete a student. Oh yeah, and separation of concerns; you need to put your two JavaScript files somewhere in the public folder and link them to the appropriate html page. • Your routes in index.js should only have to provide data. Formatting is done thru Vue.js
The assignment here is to write an app using a database named CIT321 with a collection named students; we will provide a CSV file of the data. You need to use Vue.js to display 2 pages. You should know that this assignment is similar, all too similar in fact, to the cars4sale2 example in the lecture notes for Vue.js 2. You should study that program first. If you figure out cars4sale2, then program 6 will be extremely straightforward. It is not my intent do drop a ton of new material here in the last few days of class. The database contains 51 documents. The first rows of the CSV file look like this: sid last_name 1 Astaire first_name Humphrey CIT major hrs_attempted gpa_points 10 34 2 Bacall Katharine EET 40 128 3 Bergman Bette EET 42 97 4 Bogart Cary CIT 11 33 5 Brando James WEB 59 183 6 Cagney Marlon CIT 13 40 GPA is calculated as gpa_points divided by hrs_attempted. GPA points would have been arrived at by adding 4 points for each credit hour of A, 3 points for each credit hour of B, and so on. Humphrey Astaire would have a gpa of 34/10 = 3.4. The CSV file will be on the course website. It is named CIT321.csv. Here is what your model should look like. The model is named Student.js var mongoose = require('mongoose'); mongoose.connect ('mongodb://127.0.0.1:27017/CIT321'); var studentSchema = new mongoose. Schema ({ }); sid: {type: Number, required: true, unique: true}, last_name: {type: String, required: true}, first_name: {type: String, required: true}, major: {type: String, required: true}, hrs_attempted: {type: Number, required: true}, gpa_points: {type: Number, required: true} module.exports = mongoose.model ('Student', studentSchema); Here's what the pages should look like, more or less. You have 2 pages, index.html and edit.html in the public folder. The navbar has links named Home and Edit Student Data. Home opens the index.html page that has a drop-down list that will allow us to filter the students by major Our Website Home Edit Student Data CIT 321 Students Select a Major Here are the requirements. Use Vue.js and Bootstrap. Your pages do not need to look exactly like mine, but they need to convey the same information. • Your database and collection, along with your model, must be compatible with mine. The index page should allow filtering all students by major and displaying their GPA • • • The Edit Student data pages should allow displaying each document one at a time, allow editing major, gpa points and hrs attempted. You should be able to delete a student. Oh yeah, and separation of concerns; you need to put your two JavaScript files somewhere in the public folder and link them to the appropriate html page. • Your routes in index.js should only have to provide data. Formatting is done thru Vue.js
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter11: More Object-oriented Programming Concepts
Section: Chapter Questions
Problem 2FTB
Related questions
Question

Transcribed Image Text:The assignment here is to write an app using a database named CIT321 with a collection named
students; we will provide a CSV file of the data. You need to use Vue.js to display 2 pages. You should
know that this assignment is similar, all too similar in fact, to the cars4sale2 example in the lecture notes
for Vue.js 2. You should study that program first. If you figure out cars4sale2, then program 6 will be
extremely straightforward. It is not my intent do drop a ton of new material here in the last few days of
class.
The database contains 51 documents. The first rows of the CSV file look like this:
sid
last_name
1 Astaire
first_name
Humphrey CIT
major
hrs_attempted
gpa_points
10
34
2
Bacall
Katharine EET
40
128
3 Bergman
Bette
EET
42
97
4
Bogart
Cary
CIT
11
33
5 Brando
James
WEB
59
183
6 Cagney
Marlon
CIT
13
40
GPA is calculated as gpa_points divided by hrs_attempted. GPA points would have been arrived at by
adding 4 points for each credit hour of A, 3 points for each credit hour of B, and so on. Humphrey Astaire
would have a gpa of 34/10 = 3.4.
The CSV file will be on the course website. It is named CIT321.csv.
Here is what your model should look like. The model is named Student.js
var mongoose = require('mongoose');
mongoose.connect ('mongodb://127.0.0.1:27017/CIT321');
var studentSchema = new mongoose. Schema ({
});
sid: {type: Number, required: true, unique: true},
last_name: {type: String, required: true},
first_name: {type: String, required: true},
major: {type: String, required: true},
hrs_attempted: {type: Number, required: true},
gpa_points: {type: Number, required: true}
module.exports = mongoose.model ('Student', studentSchema);
Here's what the pages should look like, more or less. You have 2 pages, index.html and edit.html in the
public folder. The navbar has links named Home and Edit Student Data. Home opens the index.html page
that has a drop-down list that will allow us to filter the students by major
Our Website Home Edit Student Data
CIT 321 Students
Select a Major

Transcribed Image Text:Here are the requirements.
Use Vue.js and Bootstrap. Your pages do not need to look exactly like mine, but they need to
convey the same information.
•
Your database and collection, along with your model, must be compatible with mine.
The index page should allow filtering all students by major and displaying their GPA
•
•
•
The Edit Student data pages should allow displaying each document one at a time, allow editing
major, gpa points and hrs attempted.
You should be able to delete a student.
Oh yeah, and separation of concerns; you need to put your two JavaScript files somewhere in
the public folder and link them to the appropriate html page.
•
Your routes in index.js should only have to provide data. Formatting is done thru Vue.js
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
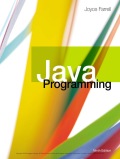
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
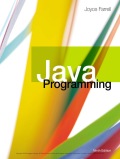
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L
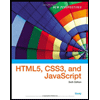
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
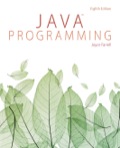
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT