Use the following formula to calculate the area of a triangle: A = 1/2 × base x height TASK 2: Create a TestTriangle class in an individual .java file. In the main method, (1) Create a Triangle object with default base and height values. (2) Create a Triangle object with a specified base = 3 and height =4. TASK 3: Add a toString() method to the Triangle class. In this toString() method, (1) Print the base, height, and area of a Triangle object. (2) Call the toString() method of the superclass using the super key word. Then, in the main method of the TestTriangle class, make the two Triangle objects that you created in Task 2 call the toString() method of the Triangle class. Ensure it runs without errors. Take a screenshot of your console output. TASK 4: Let's test the method overriding. 1. Add a void testOverriding() method to the SimpleGeometricObject class. Make it print "This is the testOverriding() method of the SimpleGeometricObject class". 2. In the main method of the TestTriangle class, make the two Triangle objects that you created in Task 2 call the testOverriding () method. Ensure it runs without errors. Take a screenshot of your console output. 3. Override the testOverriding() method in the Triangle class. Make it print "This is the overridden testOverriding() method". 4. In the main method of the TestTriangle class, make the two Triangle objects that you created in Task 2 call the testOverriding () method. Ensure it runs without errors. Take a screenshot of your console output. Question 1 (15 Points) Inheritance: In this question, we are going to create a new subclass of the SimpleGeometricObject class, named Triangle. Create a SimpleGeometricObject.java and Copy the source code of the SimpleGeometricObject class from the following link: https://liveexample.pearsoncmg.com/html/SimpleGeometricObject.html TASK 1: Create a Triangle class that extends the SimpleGeometricObject class in Eclipse, following the below UML diagram. + base:double = 5 + height:double = 10 Triangle + Triangle() + Triangle(newBase: double, newHeight: double) + getArea(): double + setBase(): void + setHeight(): void + getBase(): double + getHeight(): double
Use the following formula to calculate the area of a triangle: A = 1/2 × base x height TASK 2: Create a TestTriangle class in an individual .java file. In the main method, (1) Create a Triangle object with default base and height values. (2) Create a Triangle object with a specified base = 3 and height =4. TASK 3: Add a toString() method to the Triangle class. In this toString() method, (1) Print the base, height, and area of a Triangle object. (2) Call the toString() method of the superclass using the super key word. Then, in the main method of the TestTriangle class, make the two Triangle objects that you created in Task 2 call the toString() method of the Triangle class. Ensure it runs without errors. Take a screenshot of your console output. TASK 4: Let's test the method overriding. 1. Add a void testOverriding() method to the SimpleGeometricObject class. Make it print "This is the testOverriding() method of the SimpleGeometricObject class". 2. In the main method of the TestTriangle class, make the two Triangle objects that you created in Task 2 call the testOverriding () method. Ensure it runs without errors. Take a screenshot of your console output. 3. Override the testOverriding() method in the Triangle class. Make it print "This is the overridden testOverriding() method". 4. In the main method of the TestTriangle class, make the two Triangle objects that you created in Task 2 call the testOverriding () method. Ensure it runs without errors. Take a screenshot of your console output. Question 1 (15 Points) Inheritance: In this question, we are going to create a new subclass of the SimpleGeometricObject class, named Triangle. Create a SimpleGeometricObject.java and Copy the source code of the SimpleGeometricObject class from the following link: https://liveexample.pearsoncmg.com/html/SimpleGeometricObject.html TASK 1: Create a Triangle class that extends the SimpleGeometricObject class in Eclipse, following the below UML diagram. + base:double = 5 + height:double = 10 Triangle + Triangle() + Triangle(newBase: double, newHeight: double) + getArea(): double + setBase(): void + setHeight(): void + getBase(): double + getHeight(): double
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 5PE
Related questions
Question

Transcribed Image Text:Use the following formula to calculate the area of a triangle:
A = 1/2 × base x height
TASK 2: Create a TestTriangle class in an individual .java file. In the main method,
(1) Create a Triangle object with default base and height values.
(2) Create a Triangle object with a specified base = 3 and height =4.
TASK 3: Add a toString() method to the Triangle class. In this toString() method,
(1) Print the base, height, and area of a Triangle object.
(2) Call the toString() method of the superclass using the super key word.
Then, in the main method of the TestTriangle class, make the two Triangle objects that you
created in Task 2 call the toString() method of the Triangle class. Ensure it runs without errors.
Take a screenshot of your console output.
TASK 4: Let's test the method overriding.
1. Add a void testOverriding() method to the SimpleGeometricObject class. Make it print
"This is the testOverriding() method of the SimpleGeometricObject class".
2. In the main method of the TestTriangle class, make the two Triangle objects that you
created in Task 2 call the testOverriding () method.
Ensure it runs without errors. Take a screenshot of your console output.
3. Override the testOverriding() method in the Triangle class. Make it print "This is the
overridden testOverriding() method".
4. In the main method of the TestTriangle class, make the two Triangle objects that you
created in Task 2 call the testOverriding () method.
Ensure it runs without errors. Take a screenshot of your console output.

Transcribed Image Text:Question 1 (15 Points) Inheritance:
In this question, we are going to create a new subclass of the SimpleGeometricObject class,
named Triangle. Create a SimpleGeometricObject.java and Copy the source code of the
SimpleGeometricObject class from the following link:
https://liveexample.pearsoncmg.com/html/SimpleGeometricObject.html
TASK 1: Create a Triangle class that extends the SimpleGeometricObject class in Eclipse, following
the below UML diagram.
+ base:double = 5
+ height:double = 10
Triangle
+ Triangle()
+ Triangle(newBase: double, newHeight: double)
+ getArea(): double
+ setBase(): void
+ setHeight(): void
+ getBase(): double
+ getHeight(): double
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 6 images

Recommended textbooks for you
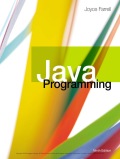
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
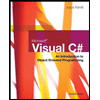
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
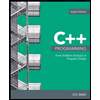
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
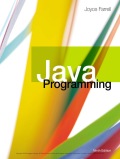
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
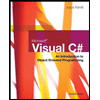
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
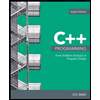
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage