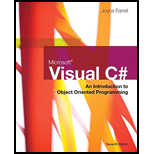
a. Write a FractionDemo
• Create properties for each field. The set access or for the denominator should not allow a 0 value; the value defaults to 1.
• Add three constructors. One takes three parameters for a whole number, numerator, and denominator. Another accepts two parameters for the numerator and denominator; when this constructor is used, the whole number value is 0. The last constructor is parameterless; it sets the whole number and numerator to 0 and the denominator to 1. (After construction, Fractions do not have to be reduced to proper form. For example, even though 3/9 could be reduced to 1/3, your constructors do not have to perform this task.)
• Add a Reduce() method that reduces a Fraction if it is in improper form. For example, 2/4 should be reduced to 1/2.
• Add an operator+() method that adds two Fractions. To add two fractions, first eliminate any whole number part of the value. For example, 2 1/4 becomes 9/4 and 1 3/5 becomes 8/5. Find a common denominator and convert the fractions to it. For example, when adding 9/4 and 8/5, you can convert them to 45/20 and 32/20. Then you can add the numerators, giving 77/20. Finally, call the Reduce() method to reduce the result, restoring any whole number value so the fractional part of the number is less than 1. For example, 77/20 becomes 3 17/20.
• Include a function that returns a string that contains a Fraction in the usual display format—the whole number, a space, the numerator, a slash (D, and a denominator. When the whole number is 0, just the Fraction part of the value should be displayed (for example, 1/2 instead of 0 1/2). If the numerator is 0, just the whole number should be displayed (for example, 2 instead of 2 0/3).
b. Add an operator*() method to the Fraction class created in Exercise 11a so that it correctly multiplies two Fractions. The result should be in proper, reduced format. Demonstrate that the method works correctly in a program named FractionDemo2.
c. Write a program named FractionDem03 that includes an array of four Fractions. Prompt the user for values for each. Display every possible combination of addition results and every possible combination of multiplication results for each Fraction pair (that is, each type will have 16 results).

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Microsoft Visual C#
- Answer this JAVA OOP question below: An Employee has a name, employee ID, and department. An Employee object must be created with all its attributes. The UML diagram is provided below: - name: String - employeeId: String - department: String + Employee(name: String, employeeId: String, department: String) + setName(name: String): void + setEmployeeId(employeeId: String): void + setDepartment(department: String): void + getName(): String + getEmployeeId(): String + getDepartment(): String + toString(): String A faculty is an Employee with an additional field String field: rank public class TestImplementation{ public static void main(String[] args){ Employee[] allEmployee = new Employee[100]; // create an employee object with name Tom Evan, employee ID 001 and department IST and store it in allEmployee // create a faculty object with name Adam Scott, employee ID 002, department IST and rank Professor and store it in allEmployee } }arrow_forwardPlease answer this JAVA OOP question that is given below: An Employee has a name, employee ID, and department. An Employee object must be created with all its attributes. The UML diagram is provided below: - name: String - employeeId: String - department: String + Employee(name: String, employeeId: String, department: String) + setName(name: String): void + setEmployeeId(employeeId: String): void + setDepartment(department: String): void + getName(): String + getEmployeeId(): String + getDepartment(): String + toString(): String A faculty is an Employee with an additional field String field: rank Assuming the Employee class is fully implemented, define a Professor class in Java with the following: A toString() method that includes both the inherited attributes and the specializationarrow_forwardPlease answer JAVA OOP question below: An Employee has a name, employee ID, and department. An Employee object must be created with all its attributes. The UML diagram is provided below: - name: String - employeeId: String - department: String + Employee(name: String, employeeId: String, department: String) + setName(name: String): void + setEmployeeId(employeeId: String): void + setDepartment(department: String): void + getName(): String + getEmployeeId(): String + getDepartment(): String + toString(): String A faculty is an Employee with an additional field String field: rank Assuming the Employee class is fully implemented, define a Professor class in Java with the following: Instance variable(s) A Constructorarrow_forward
- Develop a C++ program that execute the operation as stated by TM for addition of two binary numbers (see attached image). Your code should receive two binary numbers and output the resulting sum (also in binary). Make sure your code mimics the TM operations (dealing with the binary numbers as a string of characters 1 and 0, and following the logic to increase the first number and decreasing the second one. Try your TM for the following examples: 1101 and 101, resulting 10010; and 1101 and 11, resulting 10000.arrow_forwardI need to define and discuss the uses of one monitoring or troubleshooting tool in Windows Server 2019. thank youarrow_forwardI would likr toget help with the following concepts: - Windows Server features - Windows Server versus Windows 10 used as a client-server networkarrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
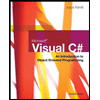
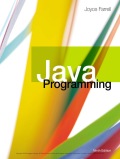
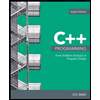
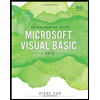
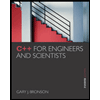