c. You can use the automatic generation approach for Getters, setters and toString() in Eclipse. d. In getMonthlyInterest Rate(), please return the monthly interest rate. DO NOT leave this method body blank! e. In getMonthlyInterest(), please returns the monthly interest (NOT THE INTEREST RATE), DO NOT leave this method body blank! f. In deposit() method, please deposit a specified amount to the account (Hint: balance += depositAmount). DO NOT leave this method body blank! g. In withdraw() method, please withdraw a specified amount from the account (Hint: balance == withdrawAmount). DO NOT leave this method body blank! h. Please use decimal to represent the annual interest rate. Example: if the annual interest rate is 2%, please use 0.02 in the annuallnterest Rate data field. HINT: a. Monthly interest is balance * Monthly Interest Rate. b. Monthly Interest Rate is Annual Interest Rate / 12. c. Example, if the balance is $1,000 and the annual interest rate is 0.02, the monthly interest rate is 0.02 / 12 = 0.001666666666667 and the monthly interest is 1000 * 0.02 / 12 = 1.666666666666667. d. When create dataCreated, use: private java.util.Date dateCreated = new java.util.Date(); 3. Create a AccountTest class in another .jave file to test the class Account you just created (10 points). Requirements: a. Create an object of Account class with an account ID of 1122, a balance of $20,000, object name is Account1. b. Set the annualInterest Rate to 0.045 using the setter of annuallnterestRate. c. Use the withdraw() method to withdraw $2,500 d. Print the balance, the monthly interest, and the date. e. Take a screenshot of the Java console outputs. CPS 2231 Computer Programming Homework #3 Due Date: Posted on Canvas 1. Provide answers to the following Check Point Questions from our textbook (5 points): a. How do you define a class? How do you define a class in Eclipse? b. How do you declare an object's reference variable (Hint: object's reference variable is the name of that object)? c. How do you create an object? d. What are the differences between constructors and regular methods? e. Explain why we need classes and objects in Java programming. 2. Write the Account class. The UML diagram of the class is represented below (10 points): Account id: int = 0 - balance: double = 0 - annualInterestRate: double = 0.02 - dateCreated: java.util.Date + Account() + Account(id: int, balance: double) + getId(): int + setId(newId: int): void + getBalance(): double + setBalance(newBalance: double): void + getAnnualInterestRate(): double + setAnnualInterest Rate (newRate: double): void + toString(): String + getDataCreated(): java.util.Date + getMonthly InterestRate(): double + + getMonthlyInterest(): double deposit(depositAmount: double): void + withdraw(withdrawAmount: double): void Requirements: a. Implement the class strictly according to its UML one-to-one (do not include anything extra, do not miss any data fields or methods) b. Add comments to your program (mark where data fields, constructors, getters, setters and toString() are)
c. You can use the automatic generation approach for Getters, setters and toString() in Eclipse. d. In getMonthlyInterest Rate(), please return the monthly interest rate. DO NOT leave this method body blank! e. In getMonthlyInterest(), please returns the monthly interest (NOT THE INTEREST RATE), DO NOT leave this method body blank! f. In deposit() method, please deposit a specified amount to the account (Hint: balance += depositAmount). DO NOT leave this method body blank! g. In withdraw() method, please withdraw a specified amount from the account (Hint: balance == withdrawAmount). DO NOT leave this method body blank! h. Please use decimal to represent the annual interest rate. Example: if the annual interest rate is 2%, please use 0.02 in the annuallnterest Rate data field. HINT: a. Monthly interest is balance * Monthly Interest Rate. b. Monthly Interest Rate is Annual Interest Rate / 12. c. Example, if the balance is $1,000 and the annual interest rate is 0.02, the monthly interest rate is 0.02 / 12 = 0.001666666666667 and the monthly interest is 1000 * 0.02 / 12 = 1.666666666666667. d. When create dataCreated, use: private java.util.Date dateCreated = new java.util.Date(); 3. Create a AccountTest class in another .jave file to test the class Account you just created (10 points). Requirements: a. Create an object of Account class with an account ID of 1122, a balance of $20,000, object name is Account1. b. Set the annualInterest Rate to 0.045 using the setter of annuallnterestRate. c. Use the withdraw() method to withdraw $2,500 d. Print the balance, the monthly interest, and the date. e. Take a screenshot of the Java console outputs. CPS 2231 Computer Programming Homework #3 Due Date: Posted on Canvas 1. Provide answers to the following Check Point Questions from our textbook (5 points): a. How do you define a class? How do you define a class in Eclipse? b. How do you declare an object's reference variable (Hint: object's reference variable is the name of that object)? c. How do you create an object? d. What are the differences between constructors and regular methods? e. Explain why we need classes and objects in Java programming. 2. Write the Account class. The UML diagram of the class is represented below (10 points): Account id: int = 0 - balance: double = 0 - annualInterestRate: double = 0.02 - dateCreated: java.util.Date + Account() + Account(id: int, balance: double) + getId(): int + setId(newId: int): void + getBalance(): double + setBalance(newBalance: double): void + getAnnualInterestRate(): double + setAnnualInterest Rate (newRate: double): void + toString(): String + getDataCreated(): java.util.Date + getMonthly InterestRate(): double + + getMonthlyInterest(): double deposit(depositAmount: double): void + withdraw(withdrawAmount: double): void Requirements: a. Implement the class strictly according to its UML one-to-one (do not include anything extra, do not miss any data fields or methods) b. Add comments to your program (mark where data fields, constructors, getters, setters and toString() are)
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter12: Event-driven Gui Programming, Multithreading, And Animation
Section: Chapter Questions
Problem 8PE
Related questions
Question

Transcribed Image Text:c. You can use the automatic generation approach for Getters, setters and toString() in
Eclipse.
d. In getMonthlyInterest Rate(), please return the monthly interest rate. DO NOT leave this
method body blank!
e. In getMonthlyInterest(), please returns the monthly interest (NOT THE INTEREST RATE),
DO NOT leave this method body blank!
f. In deposit() method, please deposit a specified amount to the account (Hint: balance +=
depositAmount). DO NOT leave this method body blank!
g. In withdraw() method, please withdraw a specified amount from the account (Hint:
balance == withdrawAmount). DO NOT leave this method body blank!
h. Please use decimal to represent the annual interest rate. Example: if the annual interest
rate is 2%, please use 0.02 in the annuallnterest Rate data field.
HINT:
a. Monthly interest is balance * Monthly Interest Rate.
b. Monthly Interest Rate is Annual Interest Rate / 12.
c. Example, if the balance is $1,000 and the annual interest rate is 0.02, the monthly
interest rate is 0.02 / 12 = 0.001666666666667 and the monthly interest is 1000 * 0.02 /
12 = 1.666666666666667.
d. When create dataCreated, use: private java.util.Date dateCreated = new
java.util.Date();
3. Create a AccountTest class in another .jave file to test the class Account you just created
(10 points).
Requirements:
a. Create an object of Account class with an account ID of 1122, a balance of $20,000,
object name is Account1.
b. Set the annualInterest Rate to 0.045 using the setter of annuallnterestRate.
c. Use the withdraw() method to withdraw $2,500
d. Print the balance, the monthly interest, and the date.
e. Take a screenshot of the Java console outputs.

Transcribed Image Text:CPS 2231 Computer Programming
Homework #3
Due Date: Posted on Canvas
1. Provide answers to the following Check Point Questions from our textbook (5 points):
a. How do you define a class? How do you define a class in Eclipse?
b. How do you declare an object's reference variable (Hint: object's reference variable
is the name of that object)?
c. How do you create an object?
d. What are the differences between constructors and regular methods?
e. Explain why we need classes and objects in Java programming.
2. Write the Account class. The UML diagram of the class is represented below (10 points):
Account
id: int = 0
- balance: double = 0
- annualInterestRate: double = 0.02
- dateCreated: java.util.Date
+ Account()
+
Account(id: int, balance: double)
+ getId(): int
+ setId(newId: int): void
+ getBalance(): double
+ setBalance(newBalance: double): void
+
getAnnualInterestRate(): double
+ setAnnualInterest Rate (newRate: double): void
+ toString(): String
+ getDataCreated(): java.util.Date
+ getMonthly InterestRate(): double
+
+
getMonthlyInterest(): double
deposit(depositAmount: double): void
+ withdraw(withdrawAmount: double): void
Requirements:
a. Implement the class strictly according to its UML one-to-one (do not include anything
extra, do not miss any data fields or methods)
b. Add comments to your program (mark where data fields, constructors, getters, setters
and toString() are)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
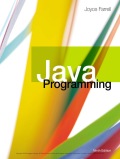
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
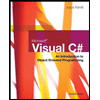
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
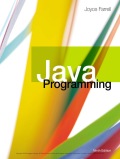
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
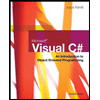
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
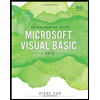
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
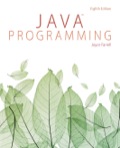
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
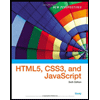
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning