Create a Database in JAVA Netbeans that saves name of the player and how many labyrinths did the player solve. Record the number of how many labyrinths did the player solve, and if he loses his life, then save this number together with his name into the database. Create a menu item, which displays a highscore table of the players for the 10 best scores public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private void checkGameState() { if (player.getX() == 0 && player.getY() == labyrinth.getSize() - 1) { JOptionPane.showMessageDialog(this, "You escaped! Congratulations!"); System.exit(0); } if (Math.abs(player.getX() - dragon.getX()) <= 1 && Math.abs(player.getY() - dragon.getY()) <= 1) { JOptionPane.showMessageDialog(this, "The dragon caught you! Game Over."); System.exit(0); } } } public class Game { /** * @param args the command line arguments */ public static void main(String[] args) { Labyrinth labyrinth = new Labyrinth(10); Player player = new Player(9, 0); Random rand = new Random(); Dragon dragon = new Dragon(rand.nextInt(10), 9); JFrame frame = new JFrame("Labyrinth Game"); //ask player's name GameGUI gui = new GameGUI(labyrinth, player, dragon); frame.setLayout(new BorderLayout()); frame.setSize(600, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.add(gui, BorderLayout.CENTER); frame.pack(); frame.setResizable(false); frame.setVisible(true); }
Create a
Record the number of how many labyrinths did the player solve, and if he loses his life, then save this number together with his name into the database. Create a menu item, which displays a highscore table of the players for the 10 best scores
public class GameGUI extends JPanel {
private final Labyrinth labyrinth;
private final Player player;
private final Dragon dragon;
private void checkGameState() {
if (player.getX() == 0 && player.getY() == labyrinth.getSize() - 1) {
JOptionPane.showMessageDialog(this, "You escaped! Congratulations!");
System.exit(0);
}
if (Math.abs(player.getX() - dragon.getX()) <= 1 &&
Math.abs(player.getY() - dragon.getY()) <= 1) {
JOptionPane.showMessageDialog(this, "The dragon caught you! Game Over.");
System.exit(0);
}
}
}
public class Game {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
Labyrinth labyrinth = new Labyrinth(10);
Player player = new Player(9, 0);
Random rand = new Random();
Dragon dragon = new Dragon(rand.nextInt(10), 9);
JFrame frame = new JFrame("Labyrinth Game");
//ask player's name
GameGUI gui = new GameGUI(labyrinth, player, dragon);
frame.setLayout(new BorderLayout());
frame.setSize(600, 600);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(gui, BorderLayout.CENTER);
frame.pack();
frame.setResizable(false);
frame.setVisible(true);
}
Unlock instant AI solutions
Tap the button
to generate a solution
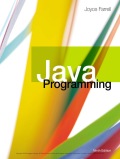
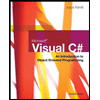
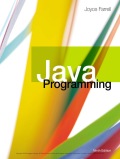
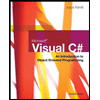
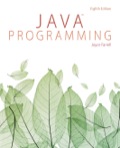