Using the following c++ header file near the bottom for context, fill in the "TODO" commented parts of the following function to finish the function implementation for the following findMax function: FUNCTION: // PURPOSE: Finds the Maximum element in the left sub-tree of V // and also deletes that vertex el_t BST::findMax(Vertex *V) { Vertex *Parent = V; V = V->left; // start with the left child of V while (/*TODO*/) {// TODO while the right child of V is still available //TODO update Parent and V to go to the right } // reached NULL Right -- V now has the MAX element el_t X = V->elem; cout << "...Max is " << X << endl; remove(V, Parent); // remove the MAX vertex return X; // return the MAX element }// end of FindMax --------------------------------------------------------------------------------------------- HEADER FILE: // tree element type is int for nowtypedef int el_t; // el_t is hidden from the client // definition of what a Vertex is - also hidden from the clientstruct Vertex{ Vertex *up; // points to the parent node Vertex *left; el_t elem; Vertex *right; int height; int balance;}; // this is set up to be inherited by another classclass BST {public: BST(); // intializes Root ~BST(); // destructor calls dtraverse to destroy the dynamic tree // PURPOSE: these will show the vertices in IN order // TO CALL: No parameter but provide a pointer to // the root vertex in calling INorderTraversal void Display(); void InOrderTraversal(Vertex*); // recursive // PURPOSE: these will search in PRE order - same as Depth First // TO CALL: provide the element to search for; provide a pointer to // the root vertex in calling PREorderSearch bool Search(el_t); bool PreOrderSearch(Vertex*, el_t); // recursive
Using the following c++ header file near the bottom for context, fill in the "TODO" commented parts of the following function to finish the function implementation for the following findMax function:
FUNCTION:
HEADER FILE:
// tree element type is int for now
typedef int el_t; // el_t is hidden from the client
// definition of what a Vertex is - also hidden from the client
struct Vertex
{
Vertex *up; // points to the parent node
Vertex *left;
el_t elem;
Vertex *right;
int height;
int balance;
};
// this is set up to be inherited by another class
class BST {
public:
BST(); // intializes Root
~BST(); // destructor calls dtraverse to destroy the dynamic tree
// PURPOSE: these will show the vertices in IN order
// TO CALL: No parameter but provide a pointer to
// the root vertex in calling INorderTraversal
void Display();
void InOrderTraversal(Vertex*); // recursive
// PURPOSE: these will search in PRE order - same as Depth First
// TO CALL: provide the element to search for; provide a pointer to
// the root vertex in calling PREorderSearch
bool Search(el_t);
bool PreOrderSearch(Vertex*, el_t); // recursive
Unlock instant AI solutions
Tap the button
to generate a solution
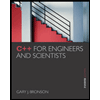
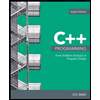
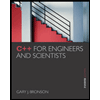
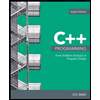
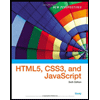
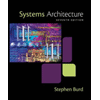