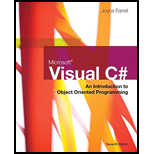
Write a
full name—the first and last names separated by a space. Then perform the following
• Create two child classes of Salesperson: Real EstateSalesperson and Girl Scout. The Real EstateSalesperson class contains fields for total value sold in dollars and total commission earned (both of which are initialized to 0), and a commission rate field required by the class constructor. The Girl Scout class includes a field to hold the number of boxes of cookies sold, which is initialized to 0. Include properties for every field.
• Create an interface named ISell able that contains two methods: SalesSpeech() and MakeSale(). In each Real EstateSalesperson and Girl Scout class, implement SalesSpeech() to display an appropriate one- or two-sentence sales speech that the objects of the class could use.
In the Real Estatesalesperson class, implement the MakeSale() method to accept an integer dollar value for a house, add the value to the Real EstateSalesperson’s total value sold, and compute the total commission earned. In the Girl Scout class, implement the MakeSale() method to accept an integer representing the number of boxes of cookies sold and add it to the total field.

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Microsoft Visual C#
- This is the question - Create a class named Rock that acts as a superclass for rock samples collected and catalogued by a natural history museum. The Rock class contains the following fields: sampleNumber - of type int description - A description of the type of rock (of type String) weight - The weight of the rock in grams (of type double) Include a constructor that accepts parameters for the sample number and weight. The Rock constructor sets the description value to "Unclassified". Include get methods for each field. Create three child classes named IgneousRock, SedimentaryRock, and MetamorphicRock. The constructors for these classes require parameters for the sample number and weight. Search the Internet for a brief description of each rock type and assign it to the description field using a method named setDescription inside of the constructor. This is the code I have, the program does not like at all what I have - import java.util.*; public class DemoRock { public static…arrow_forwardThis is the question - Create a class named Rock that acts as a superclass for rock samples collected and catalogued by a natural history museum. The Rock class contains the following fields: sampleNumber - of type int description - A description of the type of rock (of type String) weight - The weight of the rock in grams (of type double) Include a constructor that accepts parameters for the sample number and weight. The Rock constructor sets the description value to "Unclassified". Include get methods for each field. Create three child classes named IgneousRock, SedimentaryRock, and MetamorphicRock. The constructors for these classes require parameters for the sample number and weight. Search the Internet for a brief description of each rock type and assign it to the description field using a method named setDescription inside of the constructor. I am only missing two checks and they seem to be with the rock, here is my code then I will copy one check - import java.util.*;…arrow_forwardConstruct a Time class containing integer data members seconds, minutes, and hours. Have the class contain two constructors: The first should be a default constructor having the prototype Time(int, int, int), which uses default values of 0 for each data member. The second constructor should accept a long integer representing a total number of seconds and disassemble the long integer into hours, minutes, and seconds. The final member method should display the class data members.arrow_forward
- 1- Create a hierarchy of Java classes as follows: MyRectangle is_a MyShape; MyOval is a MyShape. Class MyPoint: Class MyPoint is used by class MyShape to define the reference point, p(x, y), of the Java display coordinate system, as well as by all subclasses in the class hierarchy to define the points stipulated in the subclass definition. The class utilizes a color of enum reference type MyColor, and includes appropriate class constructors and methods. The class also includes draw and toString methods, as well as methods that perform point related operations, including but not limited to, shift of point position, distance to the origin and to another point, angle [in degrees] with the x-axis of the line extending from this point to another point. Enum MyColor: Enum MyColor is used by class MyShape and all subclasses in the class hierarchy to define the colors of the shapes. The enum reference type defines a set of constant colors by their red, green, blue, and opacity, components,…arrow_forwardIn Java Programming, 2 class needs a no-arg constructor and a constructor that initializes all fields with data (The ProductionWorker class constructor should receive data for the Employee class fields as well). I need help to create a method within the Employee and ProductionWorker classes called displayInfo. (displayInfo accepts no arguments and returns void). To display the data for each constructor, the demonstration class will call the subclass displayInfo method and it will call the superclass displayInfo method. User input is not required for the demonstration class. Have a class named "Employee" with the following fields: - Employee Name - Employee Number in format XXX-L, X = digit w/ range 0-9 & L = letter w/ range A-M. - Hire Date Then one or more constructors and the appropriate accessor and mutator methods for the class. Also, another class named "ProductionWorker" that extends "Employee" class. The "ProductionWorker" class should have the following fields to hold…arrow_forwardThis is the question - Write an application that uses an abstract Insurance class and Health and Life subclasses to display different types of insurance policies and the cost per month. The Insurance class contains a String representing the type of insurance and a double that holds the monthly price. The Insurance class constructor requires a String argument indicating the type of insurance, but the Life and Health class constructors require no arguments. The Insurance class contains a get method for each field; it also contains two abstract methods named setCost() and display(). The Life class setCost() method sets the monthly fee to $36, and the Health class sets the monthly fee to $196. Code I was given - import java.util.*; public class Health extends Insurance { public Health() { // write your code here } public void setCost() { // write your code here } public void display() { // write your code here } } public…arrow_forward
- To determine if two objects in a class are equal, we should NOT use "==". We should override the _____ method of the Object class with our own definition of equality.arrow_forwardCreate a class called Rational to be able to perform arithmetic operations with fractions. testing your class and Write a program that presents a menu to the user to perform the desired action. User enters -1 value You can trade as much as you want. Use integer variables to represent the private data members (numerator and denominator) of the class. to this class Write a constructor function that initializes an object of the property as soon as it is declared. Constructive the function must contain default values for cases where no initial values are given and use the fractional number should store it in reduced form (i.e. the fraction 2/4 should be reduced to 1 for the numerator and 2 for the denominator). Provide public member functions for each of the following: ‐ The sum of two fractions - Subtraction of two fractions ‐ Multiplication of two fractions ‐ The division of two fractions In all of the above functions, results must be stored in a reduced form. Also, write the…arrow_forwardWrite a program IN C# named SalespersonDemo that instantiates objects using classes named RealEstateSalesperson and GirlScout. Demonstrate that each object can use a SalesSpeech() method appropriately. Also, use a MakeSale() method two or three times with each object, and display the final contents of each object’s data fields. First, create an abstract class named Salesperson. Fields include firstName and lastName; the Salesperson constructor requires both these values. Include properties for the fields. Include a method called GetName that returns a string that holds the Salesperson’s full name—the first and last names separated by a space. Then perform the following tasks: Create two child classes of Salesperson: RealEstateSalesperson and GirlScout. The RealEstateSalesperson class contains fields for the following: TotalValueSold - The total value sold in dollars (an int initialized to 0) TotalCommissionEarned - Total commission earned (a double initialized to 0) CommissionRate -…arrow_forward
- Vrite a general program that creates a class called Worker that has 3 pieces of information as data members. These data members are a name, a surname, and a monthly salary. Include a constructor to initialize the 3 data members. You are expected to have get and set functions for every data member. Negative valued monthly salary should be set to zero. To test the software, write a test program that shows all the capabilities of employee class. Create 2 Worker objects and show each object's yearly earning. Then give every Worker a %10 raise and show each Workers yearly earning again.arrow_forwardSanchez Construction Loan Co. makes loans of up to $100,000 for construction projects. There are two categories of Loans—those to businesses and those to individual applicants. Write an application that tracks all new construction loans. The application also must calculate the total amount owed at the due date (original loan amount + loan fee). The application should include the following classes: Loan—A public abstract class that implements the LoanConstants interface. A Loan includes a loan number, customer last name, amount of loan, interest rate, and term. The constructor requires data for each of the fields except interest rate. Do not allow loan amounts greater than $100,000. Force any loan term that is not one of the three defined in the LoanConstants class to a short-term, 1-year loan. Create a toString() method that displays all the loan data. LoanConstants—A public interface class. LoanConstants includes constant values for short-term (1 year), medium-term (3 years), and…arrow_forwardin C++arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
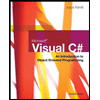
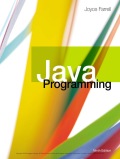
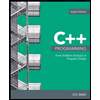