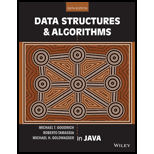
Explanation of Solution
Reimplementation of DoublyLinkedList class using only one sentinel node:
The doubly linked list is a linked data structure which contains the collection of sequentially data with two sentinel nodes such as “header” and “trailer”.
- If there is only one sentinel node, then the list is circularly linked using sentinel node.
- That is, header is sentinel node means, the next of the header is first node of list and previous node of header is last node of list.
Code for DoublyLinkedList class using only one sentinel node:
//Define the DoublyLinkedList class
public class DoublyLinkedList<E>
{
//Declare only one sentinel node
private Node<E> header;
//Declare and initialize the "size" variable
private int size = 0;
/*Define the Constructor to construct a new empty list. */
public DoublyLinkedList()
{
//Create header sentinel
header = new Node<>(null, null, null);
}
//Define the size() method
public int size()
{
/*Returns the number of elements in the linked list. */
return size;
}
//Define isEmpty() method
public boolean isEmpty()
{
//Check whether the linked list is empty
return size == 0;
}
//Define first() method
public E first()
{
//Check whether the list is empty
if(isEmpty())
//Return null
return null;
//Returns the first element of the list
return header.getNext().getElement();
}
//Define last() method
public E last( )
{
//Check whether the list is empty
if(isEmpty())
//Return null
return null;
/*Returns the last element of the list using the single sentinel node "header". */
return header.getPrev().getElement( );
}
//Define addFirst() method
public void addFirst(E e)
{
/*Call addBetween() method to adds element "e" to the front of the list. */
addBetween(e, header, header.getNext
}
/Define the addLast() method
public void addLast(E e)
{
/*Call addBetween() method to adds element e to the end of the list using single sentinel node "header". */
addBetween(e, header.getPrev(), header);
}
//Define the removeFirst() method
public E removeFirst()
{
//Check whether the list is empty
if (isEmpty())
//Return null
return null;
/*Call remove() method to removes and returns the first element of the list. */
return remove(header...

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Data Structures and Algorithms in Java
- Given the linked list data structure, implement a sub-class TSortedList that makes sure that elements are inserted and maintained in an ascending order in the list. So, given the input sequence {1,7,3,11,5}, when printing the list after inserting the last element it should print like 1, 3, 5, 7, 11. Note that with inheritance, you have to only care about the insertion situation as deletion should still be handled by the parent class.arrow_forwardCreate an implementation of a doubly linked DoubleOrderedList class. You will need to create a DoubleNode class, a DoubleList class, and a DoubleIterator classarrow_forwardImplement class “LinkedList” which has two private data members head: A pointer to the Node class length: length of the linked list // Node* GetNode(int index) const;A private function which is only accessible to the class methods. This function takes an array-like index and returns the address of the node at that index. For example, index 0 corresponds to the head and index length-1 corresponds to end node of the linked list. The function returns NULL if the index is out of bound.// Implement the following public method: 1. bool RemoveHead();// Use RemoveAt FunctionRemove first node. Return true if successful, otherwise, false.2. bool RemoveEnd();// Use RemoveAt FunctionRemove last node. Return true if successful, otherwise, false.3 . void DisplayList() const;Display data of the whole linked list this in c++.arrow_forward
- Use java and indent code properly.arrow_forwardJava - Mileage for a Runnerarrow_forwardCreate an implementation of singly linked list using classes with minimum 5 nodes (spectre, togusa, yuri, siren, shepherd) in Python with the following capabilities/functions: Traverse - print out all data from the linked list Insert - generate a node and attach to an existing linked list Search - find an item (data) from the linked list and return the node Remove - remove a node from the linked listarrow_forward
- Write a method replace to be included in the class KWLinkedList (for doubly linked list) that accepts two parameters, searchItem and repItem of type E. The method searches for searchItem in the doubly linked list, if found then replace it with repItem and return true. If the searchItem is not found in the doubly linked list, then insert repItem at the end of the linked list and return false. Assume that the list is not empty. You can use ListIterator and its methods to search the searchItem in the list and replace it with repItem if found. Do not call any method of class KWLinkedList to add a new node at the end of the list. Method Heading: public boolean replace(E searchItem, E repItem) Example: searchItem: 15 repItem: 17 List (before method call): 9 10 15 20 4 5 6 List (after method call) : 9 10 17 20 4 5 6arrow_forwardImplementing a Double-Ended List:contains the firstLastList.cpp program, which demonstrates a doubleended list. (Incidentally, don’t confuse the double-ended list with the doubly linked list, which we’ll explore later in Hour 10, “Specialized Lists.”)arrow_forwardCreate a lazy elimination deletion function for the AVLTree class.There are several approaches you can take, but one of the simplest is to add a Boolean property to the Node class that indicates whether or not the node is designated for deletion. This variable must then be considered by your other techniques.arrow_forward
- Using classes , write down a program that implements creation of a head pointer of a linked list , then add first node to the list and make it pointing to null .arrow_forwardImplements clone which duplicates a list. Pay attention, because if there are sublists, they must be duplicated as well. Understand the implementation of the following function, which recursively displays the ids of each node in a list Develop your solution as follows: First copy the nodes of the current list (self) Create a new list with the copied nodes Loop through the nodes of the new list checking the value field If this field is also a list (use isinstance as in the show_ids function) then it calls clone on that list and substitutes the value. Complete the code: def L4(*args,**kwargs): class L4_class(L): def clone(self): def clone_node(node): return <... YOUR CODE HERE ...> r = <... YOUR CODE HERE...> return r return L4_class(*args,**kwargs)arrow_forwardProblem 3: In classroom, we implemented MyStack by including an ArrayList as private data field of the class (using composition). In this problem, we will use another way to implement the stack class. Define a new MyStack class that extends ArrayList. Draw the UML diagram for the classes and then implement MyStack. Write a test program that prompts the user to enter five strings and displays them in reverse order. (1) Your UML diagram: (3)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
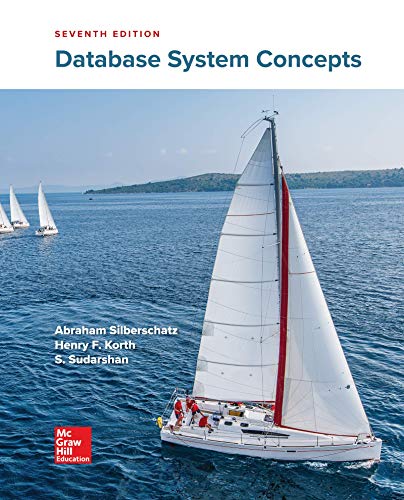
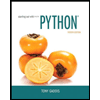
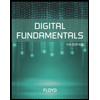
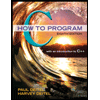
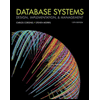
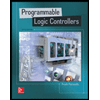