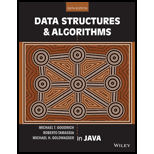
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781118771334
Author: Michael T. Goodrich
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 3, Problem 12R
Explanation of Solution
Method rotate() in Singly linked list:
//Define the rotate() method
public void rotate()
{
//Check whether the list is empty
if(isEmpty())
//Return it
return;
//Otherwise, execute else statement
else
{
/*Set the next node of "tail" as "head" after existing tail. */
tail.setNext((Node<E>) head);
}
/*Set the "tail" as "head"*/
tail=(Node<E>)head;
//Set next node of head as "head"
head = head...
Expert Solution & Answer

Learn your wayIncludes step-by-step video

schedule03:02
Students have asked these similar questions
Whentheuserenters!!,themostrecentcommandinthehistoryisexecuted.In the example above, if the user entered the command:
Osh> !!
The ‘ls -l’ command should be executed and echoed on user’s screen. The command should also be placed in the history buffer as the next command.
Whentheuserentersasingle!followedbyanintegerN,theNthcommandin the history is executed. In the example above, if the user entered the command:
Osh> ! 3
The ‘ps’ command should be executed and echoed on the user’s screen. The command should also be placed in the history buffer as the next command.
Error handling:
The program should also manage basic error handling. For example, if there are no commands in the history, entering !! should result in a message “No commands in history.” Also, if there is no command corresponding to the number entered with the single !, the program should output "No such command in history."
Activity No.
Activity
Time (weeks)
Immediate Predecessors
1
Requirements collection
3
2
Requirements structuring
4
1
3
Process analysis
3
2
4
Data analysis
3
2
5
Logical design
50
3,4
6
Physical design
5
5
7
Implementation
6
6
c. Using the information from part b, prepare a network diagram. Identify the critical path.
2. UNIX Shell and History Feature [20 points]
This question consists of designing a C program to serve as a shell interface that
accepts user commands and then executes each command in a separate process. A
shell interface gives the user a prompt, after which the next command is entered.
The example below illustrates the prompt osh> and the user's next command:
cat prog.c. The UNIX/Linux cat command displays the contents of the file
prog.c on the terminal using the UNIX/Linux cat command and your program
needs to do the same.
osh> cat prog.c
The above can be achieved by running your shell interface as a parent process.
Every time a command is entered, you create a child process by using fork(),
which then executes the user's command using one of the system calls in the
exec() family (as described in Chapter 3). A C program that provides the general
operations of a command-line shell can be seen below.
#include
#include
#define MAX LINE 80 /* The maximum length command */
{
int…
Chapter 3 Solutions
Data Structures and Algorithms in Java
Ch. 3 - Prob. 1RCh. 3 - Write a Java method that repeatedly selects and...Ch. 3 - Prob. 3RCh. 3 - The TicTacToe class of Code Fragments 3.9 and 3.10...Ch. 3 - Prob. 5RCh. 3 - Prob. 6RCh. 3 - Prob. 7RCh. 3 - Prob. 8RCh. 3 - Prob. 9RCh. 3 - Prob. 10R
Ch. 3 - Prob. 11RCh. 3 - Prob. 12RCh. 3 - Prob. 13RCh. 3 - Prob. 14RCh. 3 - Prob. 15RCh. 3 - Prob. 16RCh. 3 - Prob. 17CCh. 3 - Prob. 18CCh. 3 - Prob. 19CCh. 3 - Give examples of values for a and b in the...Ch. 3 - Suppose you are given an array, A, containing 100...Ch. 3 - Write a method, shuffle(A), that rearranges the...Ch. 3 - Suppose you are designing a multiplayer game that...Ch. 3 - Write a Java method that takes two...Ch. 3 - Prob. 25CCh. 3 - Prob. 26CCh. 3 - Prob. 27CCh. 3 - Prob. 28CCh. 3 - Prob. 29CCh. 3 - Prob. 30CCh. 3 - Prob. 31CCh. 3 - Prob. 32CCh. 3 - Prob. 33CCh. 3 - Prob. 34CCh. 3 - Prob. 35CCh. 3 - Write a Java program for a matrix class that can...Ch. 3 - Write a class that maintains the top ten scores...Ch. 3 - Prob. 38PCh. 3 - Write a program that can perform the Caesar cipher...Ch. 3 - Prob. 40PCh. 3 - Prob. 41PCh. 3 - Prob. 42PCh. 3 - Prob. 43P
Knowledge Booster
Similar questions
- Question#2: Design and implement a Java program using Abstract Factory and Singleton design patterns. The program displays date and time in one of the following two formats: Format 1: Date: MM/DD/YYYY Time: HH:MM:SS Format 2: Date: DD-MM-YYYY Time: SS,MM,HH The following is how the program works. In the beginning, the program asks the user what display format that she wants. Then the program continuously asks the user to give one of the following commands, and performs the corresponding task. Note that the program gets the current date and time from the system clock (use the appropriate Java date and time operations for this). 'd' display current date 't': display current time 'q': quit the program. • In the program, there should be 2 product hierarchies: "DateObject” and “TimeObject”. Each hierarchy should have format and format2 described above. • Implement the factories as singletons. • Run your code and attach screenshots of the results. • Draw a UML class diagram for the program.arrow_forward#include <linux/module.h> #include <linux/kernel.h> // part 2 #include <linux/sched.h> // part 2 extra #include <linux/hash.h> #include <linux/gcd.h> #include <asm/param.h> #include <linux/jiffies.h> void print_init_PCB(void) { printk(KERN_INFO "init_task pid:%d\n", init_task.pid); printk(KERN_INFO "init_task state:%lu\n", init_task.state); printk(KERN_INFO "init_task flags:%d\n", init_task.flags); printk(KERN_INFO "init_task runtime priority:%d\n", init_task.rt_priority); printk(KERN_INFO "init_task process policy:%d\n", init_task.policy); printk(KERN_INFO "init_task task group id:%d\n", init_task.tgid); } /* This function is called when the module is loaded. */ int simple_init(void) { printk(KERN_INFO "Loading Module\n"); print_init_PCB(); printk(KERN_INFO "Golden Ration Prime = %lu\n", GOLDEN_RATIO_PRIME); printk(KERN_INFO "HZ = %d\n", HZ); printk(KERN_INFO "enter jiffies = %lu\n", jiffies); return 0; } /* This function is called when the…arrow_forwardList at least five Operating Systems you know. What is the difference between the kernel mode and the user mode for the Linux? What is the system-call? Give an example of API in OS that use the system-call. What is cache? Why the CPU has cache? What is the difference between the Static Linking and Dynamic Linking when compiling the code.arrow_forward
- In the GoF book, List interface is defined as follows: interface List { int count(); //return the current number of elements in the list Object get(int index); //return the object at the index in the list Object first(); //return the first object in the list Object last(); //return the last object in the list boolean include(Object obj); //return true is the object in the list void append(Object obj); //append the object to the end of the list void prepend(Object obj); //insert the object to the front of the list void delete(Object obj); //remove the object from the list void deleteLast(); //remove the last element of the list void deleteFirst(); //remove the first element of the list void deleteAll(); //remove all elements of the list (a) Write a class adapter to adapt Java ArrayList to GoF List interface. (b) Write a main program to test your adapters through List interface. (c) Same requirement as (a) and (b), but write an object adapter to adapt Java ArrayList to GoF List…arrow_forwardIn modern packet-switched networks, including the Internet, the source host segments long, application-layer messages (for example, an image or a music file) into smaller packets and sends the packets into the network. The receiver then reassembles the packets back into the original message. We refer to this process as message segmentation. Figure 1.27 (attached) illustrates the end-to-end transport of a message with and without message segmentation. Consider a message that is 106 bits long that is to be sent from source to destination in Figure 1.27. Suppose each link in the figure is 5 Mbps. Ignore propagation, queuing, and processing delays. a. Consider sending the message from source to destination without message segmentation. How long does it take to move the message from the source host to the first packet switch? Keeping in mind that each switch uses store-and-forward packet switching, what is the total time to move the message from source host to destination host? b. Now…arrow_forwardConsider a packet of length L that begins at end system A and travels over three links to a destination end system. These three links are connected by two packet switches. Let di, si, and Ri denote the length, propagation speed, and the transmission rate of link i, for i = 1, 2, 3. The packet switch delays each packet by dproc. Assuming no queuing delays, in terms of di, si, Ri, (i = 1, 2, 3), and L, what is the total end-to-end delay for the packet? Suppose now the packet is 1,500 bytes, the propagation speed on all three links is 2.5 * 10^8 m/s, the transmission rates of all three links are 2.5 Mbps, the packet switch processing delay is 3 msec, the length of the first link is 5,000 km, the length of the second link is 4,000 km, and the length of the last link is 1,000 km. For these values, what is the end-to-end delay?arrow_forward
- how to know the weight to data and data to weight also weight by infomraion gain in rapid miner , between this flow diagram retrieve then selecte attrbuite then set role and split data and decision tree and apply model and peformance ,please show how the operators should be connected:arrow_forwardusing rapid miner how to creat decison trea for all attribute and another one with delete one or more of them also how i know the weight of each attribute and what that mean in impact the resultarrow_forwardQ.1. Architecture performance [10 marks] Answer A certain microprocessor requires either 2, 4, or 6 machine cycles to perform various operations. ⚫ (40+g+f)% require 2 machine cycles, ⚫ (30-g) % require 4 machine cycles, and ⚫ (30-f)% require 6 machine cycles. (a) What is the average number of machine cycles per instruction for this microprocessor? Answer (b) What is the clock rate (machine cycles per second) required for this microprocessor to be a "1000 MIPS" processor? Answer (c) Suppose that 35% of the instructions require retrieving an operand from memory which needs an extra 8 machine cycles. What is the average number of machine cycles per instruction, including the instructions that fetch operands from memory?arrow_forward
- Q.2. Architecture performance [25 marks] Consider two different implementations, M1 and M2, of the same instruction set. M1 has a clock rate of 2 GHz and M2 has a clock rate of 3.3 GHz. There are two classes of instructions with the following CPIs: Class A CPI for M1 CPI for M2 2.f 1.g B 5 3 C 6 4 Note that the dots in 2 fand 1.g indicate decimal points and not multiplication. a) What are the peak MIPS performances for both machines? b) Which implementation is faster, if half the instructions executed in a certain program are from class A, while the rest are divided equally among classes B and C. c) What speedup factor for the execution of class-A instructions would lead to 20% overall speedup? d) What is the maximum possible speedup that can be achieved by only improving the execution of class-A instructions? Explain why. e) What is the clock rate required for microprocessor M1 to be a "1000 MIPS" (not peak MIPS) processor?arrow_forwardPLEASE SOLVE STEP BY STEP WITHOUT ARTIFICIAL INTELLIGENCE OR CHATGPT I don't understand why you use chatgpt, if I wanted to I would do it myself, I need to learn from you, not from being a d amn robot. SOLVE STEP BY STEP I WANT THE DIAGRAM PERFECTLY IN SIMULINKarrow_forwardI need to develop and run a program that prompts the user to enter a positive integer n, and then calculate the value of n factorial n! = multiplication of all integers between 1 and n, and print the value n! on the screen. This is for C*.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- COMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE LSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
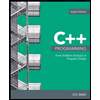
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
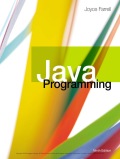
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
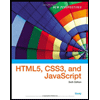
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
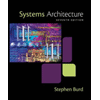
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage