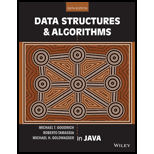
Explanation of Solution
Consequences when delete the two lines from code:
Note: Refer Code Fragment 3.15 “SinglyLinkedList class definition” in the Text book.
The SinglyLinkedList class contains number of methods such as “size()”, “first()”, “last()”, “addFirst()”, “addLast()”, “removeLast()”, and “removeFirst()”.
In removeFirst() method,
- Remove the line number “51” and “52” in this method.
- That is, resetting the “tail” field to “null”.
Modified:
In the above code, just remove or delete the Line “51” and “52”. Then the code is given below:
//Method definition
public E removeFirst( ) //Line 46
{
//Check whether the linked list is empty
if (isEmpty( )) //Line 47
//Return null, there is no element to remove
return null;
/*Get the head node from linked list and assigned it. */
E answer = head.getElement( ); //Line 48
//Assign the head node as next node of head
head = head...

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Data Structures and Algorithms in Java
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
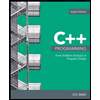
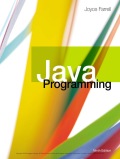
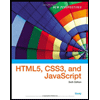
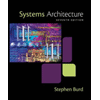
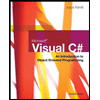