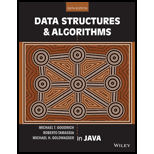
Explanation of Solution
The algorithm to concatenate two doubly linked list “L” and “M” into single list “L'” is given below:
Algorithm:
Input: Two doubly linked list “L” and “M”.
Output: Concatenate the two doubly linked lists into single list “L'”.
Concatenate(L, M):
//Create new nodes
Create a new node "n" and "v"
/*Get previous node for trailer of list "L" using getPrev() method and assign into "n". */
n = (L.getTrailer()).getPrev()
/*Get next node for header of list "M" using getNext() method and assign into "v" */
v = (M.getHeader()).getNext()
// Set next node for header of list "M" as "null"
(M.getHeader()).setNext(null)
//Set previous node for trailer of list "L" as "null"
(L.getTrailer()).setPrev(null)
/*Call setNext() method by passing the "v" node to set the next node as "v". */
n.setNext(v)
/*Call setPrev() method by passing the "n" node to set the previous node as "n". */
v.setPrev(n)
//Assign the concatenated list into single list "L'"
L' = L
/*Set trailer for list "L'" by calling setTrailer() method by passing parameter as "trailer of list "M"...

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Data Structures and Algorithms in Java
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
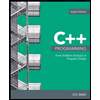
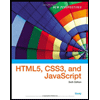
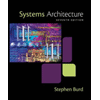
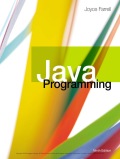
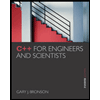