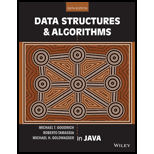
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781118771334
Author: Michael T. Goodrich
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Question
Chapter 3, Problem 32C
Program Plan Intro
Initial consideration:
A circular version of a doubly linked list has to be implemented that is not having any sentinels that can generally supports the public behaviors of the original and new update methods.
- The implemented circular doubly linked list should have method called rotate () which is used to rotate the linked list counter clockwise and rotateBackward() method that can support the public behaviors of the linked list.
- The rotateBackward() method reverses the doubly linked list in an incremented order while changing the public behavior of the original linked list.
- There is no need to use the sentinel nodes which are more generally useful with linked list implementation that helps to maintain the reference of all nodes of a doubly linked list.
- The node in the circular version of doubly linked list maintains two references with the previous and the next node in the doubly linked list.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Import the ArrayList and List classes from the java.util package to create a list of phone numbers and also import the HashSet and Set classes from the java.util package to create a set of unique prefixes.
Create a class called PhoneNumberPrefix with a main method that will contain the code to find the unique prefixes.
Create a List called phoneNumbers and use the add method to add several phone numbers to the list.
List<String> phoneNumbers = new ArrayList<>(); phoneNumbers.add("555-555-1234"); phoneNumbers.add("555-555-2345"); phoneNumbers.add("555-555-3456"); phoneNumbers.add("444-444-1234"); phoneNumbers.add("333-333-1234");
Create a Set called prefixes and use a for-each loop to iterate over the phoneNumbers list. For each phone number, we use the substring method to extract the first 7 characters, which represent the prefix, and add it to the prefixes set using the add method.
Finally, use the println method to print the prefixes set, which will contain all of…
Implement unique method that takes an ArrayList as input and returns an ArrayList withexactly a single occurrence of each element from the input list. For example, if the input listcontains 5, 6, 7, 6, 3, 5, 5, 4 then the output list should have 5, 6, 7, 3, 4.Note: (1) You may assume that the ArrayList has a specific type of elements in it. In the class, we assumedit to have String type values. (2) You may only work with ArrayList(s).
Write a method replace to be included in the class KWLinkedList (for doubly linked list) that accepts two parameters, searchItem and repItem of type E. The method searches for searchItem in the doubly linked list, if found then replace it with repItem and return true. If the searchItem is not found in the doubly linked list, then insert repItem at the end of the linked list and return false. Assume that the list is not empty.
You can use ListIterator and its methods to search the searchItem in the list and replace it with repItem if found. Do not call any method of class KWLinkedList to add a new node at the end of the list.
Method Heading:
public boolean replace(E searchItem, E repItem)
Example:
searchItem: 15 repItem: 17
List (before method call): 9 10 15 20 4 5 6
List (after method call) : 9 10 17 20 4 5 6
Chapter 3 Solutions
Data Structures and Algorithms in Java
Ch. 3 - Prob. 1RCh. 3 - Write a Java method that repeatedly selects and...Ch. 3 - Prob. 3RCh. 3 - The TicTacToe class of Code Fragments 3.9 and 3.10...Ch. 3 - Prob. 5RCh. 3 - Prob. 6RCh. 3 - Prob. 7RCh. 3 - Prob. 8RCh. 3 - Prob. 9RCh. 3 - Prob. 10R
Ch. 3 - Prob. 11RCh. 3 - Prob. 12RCh. 3 - Prob. 13RCh. 3 - Prob. 14RCh. 3 - Prob. 15RCh. 3 - Prob. 16RCh. 3 - Prob. 17CCh. 3 - Prob. 18CCh. 3 - Prob. 19CCh. 3 - Give examples of values for a and b in the...Ch. 3 - Suppose you are given an array, A, containing 100...Ch. 3 - Write a method, shuffle(A), that rearranges the...Ch. 3 - Suppose you are designing a multiplayer game that...Ch. 3 - Write a Java method that takes two...Ch. 3 - Prob. 25CCh. 3 - Prob. 26CCh. 3 - Prob. 27CCh. 3 - Prob. 28CCh. 3 - Prob. 29CCh. 3 - Prob. 30CCh. 3 - Prob. 31CCh. 3 - Prob. 32CCh. 3 - Prob. 33CCh. 3 - Prob. 34CCh. 3 - Prob. 35CCh. 3 - Write a Java program for a matrix class that can...Ch. 3 - Write a class that maintains the top ten scores...Ch. 3 - Prob. 38PCh. 3 - Write a program that can perform the Caesar cipher...Ch. 3 - Prob. 40PCh. 3 - Prob. 41PCh. 3 - Prob. 42PCh. 3 - Prob. 43P
Knowledge Booster
Similar questions
- Implement a member method for the List ADT called removeDuplicates(). The method removes every duplicate from the list such that there is only ONE copy of each element in the list. The method also returns the number of copies it removed. For example, if L = {Joe, Bob, Joe, Ned, Bob, Ron, Ron}, a call to L.removeDuplicates modifies the list such that L = {Joe, Bob, Ned, Ron} and returns 3. import java.util.Iterator;import java.util.NoSuchElementException; public class Mock1ListWrapper { public static interface List<E> extends Iterable<E> { public int size(); public boolean isEmpty(); public boolean isMember(E e); public int firstIndexOf(E e); public int lastIndexOf(E e); public void add(E e); public void add(E e, int position); public E get(int position); public E remove(int position); public E replace(int position, E newElement); public void clear(); public Object[] toArray(); public int removeDuplicates(); } @SuppressWarnings("unchecked") public static…arrow_forwardThe curly bracket used in java to enclose units of code can not be used in a fill in questions. For this questions the curly brackets will be substituted with comments. Interpret the begin and end comments as curly brackets. Complete the code for the method filter. The method receives two parameter of generic type E called low and high. The method filters the elements within the linked list and return a linked list with all the elements from the low value to the high value. Example: [1,2,4,5,8,9,11,12] Called with low = 2 and high =10 will return [2,4,5,8,91. public //begin method MyLinkedList newlist = 0; Node befPtrD if(this. ==null) return newlist; for (Node clptr= //begin for if && //begin if newlist.append(clptr.element); if( head=head.next; else if(clptr==tail) +ail-bofPtrarrow_forwardDesign and implement a getLastHalf() method for the MyLinkedList class. The method should return the last half of the list as a new list leaving the original list intact. If the list has an uneven number of elements the extra one element must be in the list returned. Example : [1,3,5,4] return [5,4] and [1,6,9] return [6,9]. Follow the three step method for designing the method Develop the java code for the method Create a test program to test the method thoroughly using the Integer wrapper class. public class MyLinkedList<E> { private Node<E> head, tail; public MyLinkedList() { head = null; tail = null; } /** Return the head element in the list */ public E getFirst() { if (head == null) { return null; } else { return head.element; } } /** Return the last element in the list */ public E getLast() { if (head==null) { return null; } else { return tail.element; } } /** Add an element to the beginning of the list */ public void prepend(E e) { Node<E> newNode = new…arrow_forward
- Design and implement a getLastHalf() method for the MyLinkedList class. The method should return the last half of the list as a new list leaving the original list intact. If the list has an uneven number of elements the extra one element must be in the list returned. Example : [1,3,5,4] return [5,4] and [1,6,9] return [6,9]. Follow the three step method for designing the method Develop the java code for the method Create a test program to test the method thoroughly using the Integer wrapper class.arrow_forwardYou may find a doubly-linked list implementation below. Our first class is Node which we can make a new node with a given element. Its constructor also includes previous node reference prev and next node reference next. We have another class called DoublyLinkedList which has start_node attribute in its constructor as well as the methods such as: 1. insert_to_empty_list() 2. insert_to_end() 3. insert_at_index() Hints: Make a node object for the new element. Check if the index >= 0.If index is 0, make new node as head; else, make a temp node and iterate to the node previous to the index.If the previous node is not null, adjust the prev and next references. Print a message when the previous node is null. 4. delete_at_start() 5. delete_at_end() . 6. display() the task is to implement these 3 methods: insert_at_index(), delete_at_end(), display(). hint on how to start thee code # Initialize the Node class Node: def __init__(self, data): self.item = data…arrow_forwardAdd a method findMiddle() that finds the middle node of a doubly linked list by link hopping, without relying on explicit knowledge of the size of the list. Counting nodes is not the correct way to do this. In the case of an even number of nodes, report the node slightly left of center as the middle. You can assume the list has at least one item. Your findMiddle() code will be located in DoublyLinkedList.java. If you’re using size(), you’re doing it wrong. Starter code for the findMiddle() method: // Janet McGregor 4/20/2053 public E findMiddle() { Node<E> middleNode = header.next; Node<E> currentNode = header.next; // the rest of your code goes here return middleNode.element; } You will test findMiddle() with the following three tests cases. Sample program output: Finding the middle of the list: (1, 2, 3, 4, 5, 6, 7) The middle value is: 4 Finding the middle of this list: (1, 2, 3, 4) The middle value is: 2 Finding the middle of this…arrow_forward
- You are required to complete the LinkedList class. This class is used as a linked list that has many methods to perform operations on the linked list. To create a linked list, create an object of this class and use the addFirst or addLast or add(must be completed) to add nodes to this linked list. Your job is to complete the empty methods. For every method you have to complete, you are provided with a header, Do not modify those headers(method name, return type or parameters). You have to complete the body of the method. package chapter02; public class LinkedList { protected LLNode list; public LinkedList() { list = null; } public void addFirst(T info) { LLNode node = new LLNode(info); node.setLink(list); list = node; } public void addLast(T info) { LLNode curr = list; LLNode newNode = new LLNode(info); if(curr == null) { list = newNode; } else { while(curr.getLink() !=…arrow_forwardSuppose we want to extend the PositionalList ADT with a method, indexOf(p), that returns the current index of the element stored at position p. Write this method using only other methods of the PositionalList interface (not details of our LinkedPositionalList implementation). Write the necessary code to test the method. Hint: Count the steps while traversing the list until encountering position p public interface PositionalList<E> extends Iterable<E> { int size(); boolean isEmpty(); Position<E> first(); Position<E> last(); Position<E> before(Position<E> p) throws IllegalArgumentException; Position<E> after(Position<E> p) throws IllegalArgumentException; Position<E> addFirst(E e); Position<E> addLast(E e); Position<E> addBefore(Position<E> p, E e) throws IllegalArgumentException; Position<E> addAfter(Position<E> p, E e) throws IllegalArgumentException; E set(Position<E> p, E e) throws…arrow_forwardFor both ArrayList and LinkedList, which methods do not exist in the List interface? Just why do you think these methods are left out the List?arrow_forward
- PLEASE HELP FAST, javaarrow_forwardAdd a method swapTwoNodes to SinglyLinkedList class from week 2 lecture examples. This method should swap two nodes node1 and node2 (and not just their contents) given references only to node1 and node2. The new method should check if node1 and node2 are the same node, etc. Write the main method to test the swapTwoNodes method. Hint: You may need to traverse the list. Please give me the full code and share how it works thank you! I need java code, not C ++ or C#.arrow_forwardImplement class “LinkedList” which has two private data members head: A pointer to the Node class length: length of the linked listImplement the following private method:1. Node* GetNode(int index) const;A private function which is only accessible to the class methods. . For example, index 0 corresponds to the head and index length-1 corresponds to end node of the linked list. The function returns NULL if the index is out of bound.Implement the following public methods:2. LinkedList();Constructor that sets head to NULL and length equal to zero.3. bool InsertAt(int data, int index);Insert a new node at the index. Return true if successful, otherwise,return false. The new node should be at the position “index” in the linked list after inserting it. You might have to use GetNode private function. these 3 partsarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
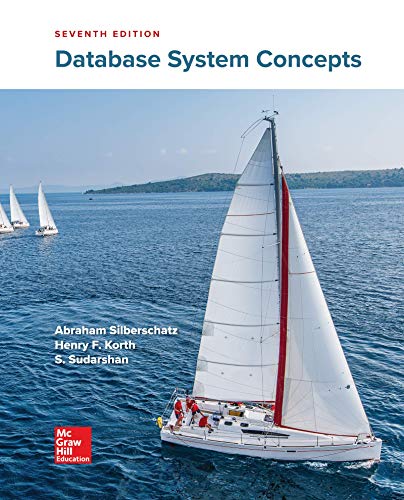
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
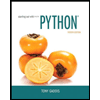
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
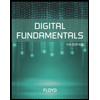
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
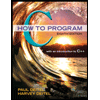
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
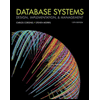
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
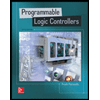
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education