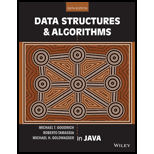
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781118771334
Author: Michael T. Goodrich
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Question
Chapter 3, Problem 40P
Program Plan Intro
Substitution Cipher
Program plan:
- Import the required java packages.
- Create the class SubstitutionCipher.
- Create object “pac” for the class SubstitutionCipher1.
- Declare the constructor.
- Create the nested class SubstitutionCipher1.
- Declare and initialize the string.
- Declare one character array.
- Declare the constructor.
- Define the method encoding() to encode the given string.
- Define the main() method,
- Create the object for SubstitutionCipher class.
- Gets the input string from the user using the scanner.
- Pass the values for parameters using the object and call the constructor to perform the defined function.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
Implement the Plates class buildMap function so that it populates the HashMap with the state abbreviations as keys and the counts of how many each appear in the file as values.
Sometimes, the parking attendant will add special notation to help her remember something about a specific entry. There are just non alphabetic characters that she adds to the state - your program should ignore these characters so that an entry like NY* still counts toward the NY plate count.
She is also very inconsistent with how she enters the plates. Sometimes she uses upper case, sometimes lowercase, and sometimes she even uses a mix. Be sure to account for this in your program.
Only add information for plates in New England (Maine, New Hampshire, Vermont, Massachusetts, Rhode Island, and Connecticut).
Plates.java
import java.util.Arrays;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
public class Plates {
private Map<String, Integer> plateMap;…
Write a Java class that would match a string. The class has method match with String as a return type. It accepts two inputs: the phrase/sentence string (text) and the pattern string (word). This method finds the first (or all) instances of the pattern in the text and changes that word in all uppercase and return the new phrase. Method countOccurence accepts a phrase/sentence and a pattern string and returns its number of occurrences.
Add a main method that will allow the user to input the phrase/sentence and pattern. Call method match and countOccurence. See test case below.
Sample Input:
Text string: You will always have my love, my love, for the love I love is as lovely as love itself.
Pattern string: love
Output:
New text: You will always have my LOVE, my LOVE, for the LOVE I LOVE is as lovely as LOVE itself.
Number of occurrence: 5
For example:
Input
Result
You will always have my love, my love, for the love I love is as lovely as love itself. love
New text: You…
For this problem, you can use all classes in the java.util package.
As a genius IT/SE student, you are asked by the Ministry of Health to help them analyze the Covid sequences. In particular, given 2 Covid variants, your task is to find the
longest common subsequence between them.
Each Covid variant has 2 properties: code (String) and sequence (String). Both properties contain only upper case letters 'A' to 'Z'. The length of the sequence is up to 100
characters.
Subsequence definition: given a string S consists of the letters s1, s2,
sn in that order. If you delete zero, one, or more letters from S without changing the letters' order, the
remaining string S2 is called a subsequence of S.
For example: given the string "HELLO", the following strings are some of its subsequences: "HELLO", "HLO", "O", "HO", "'" (empty string). The following strings are not its
subsequences: "OL", "HELLOO", "LEH".
Create a type CovidVariant that contains 2 properties: code (String), and sequence (String).…
Chapter 3 Solutions
Data Structures and Algorithms in Java
Ch. 3 - Prob. 1RCh. 3 - Write a Java method that repeatedly selects and...Ch. 3 - Prob. 3RCh. 3 - The TicTacToe class of Code Fragments 3.9 and 3.10...Ch. 3 - Prob. 5RCh. 3 - Prob. 6RCh. 3 - Prob. 7RCh. 3 - Prob. 8RCh. 3 - Prob. 9RCh. 3 - Prob. 10R
Ch. 3 - Prob. 11RCh. 3 - Prob. 12RCh. 3 - Prob. 13RCh. 3 - Prob. 14RCh. 3 - Prob. 15RCh. 3 - Prob. 16RCh. 3 - Prob. 17CCh. 3 - Prob. 18CCh. 3 - Prob. 19CCh. 3 - Give examples of values for a and b in the...Ch. 3 - Suppose you are given an array, A, containing 100...Ch. 3 - Write a method, shuffle(A), that rearranges the...Ch. 3 - Suppose you are designing a multiplayer game that...Ch. 3 - Write a Java method that takes two...Ch. 3 - Prob. 25CCh. 3 - Prob. 26CCh. 3 - Prob. 27CCh. 3 - Prob. 28CCh. 3 - Prob. 29CCh. 3 - Prob. 30CCh. 3 - Prob. 31CCh. 3 - Prob. 32CCh. 3 - Prob. 33CCh. 3 - Prob. 34CCh. 3 - Prob. 35CCh. 3 - Write a Java program for a matrix class that can...Ch. 3 - Write a class that maintains the top ten scores...Ch. 3 - Prob. 38PCh. 3 - Write a program that can perform the Caesar cipher...Ch. 3 - Prob. 40PCh. 3 - Prob. 41PCh. 3 - Prob. 42PCh. 3 - Prob. 43P
Knowledge Booster
Similar questions
- Imagine you are reading in a stream of integers. Periodically, you wishto be able to look up the rank of a number x (the number of values less than or equal to x). Implement the data structures and algorithms to support these operations. That is, implement the method track(int x), which is called when each number is generated, and the method getRankOfNumber(int x), which returns the number of values less than or equal to x (not including x itself).EXAMPLEStream(in order of appearance):5, 1, 4, 4, 5, 9, 7, 13, 3getRankOfNumber(l) 0getRankOfNumber(3) 1getRankOfNumber(4) 3arrow_forwardImagine you are reading in a stream of integers. Periodically, you wishto be able to look up the rank of a number x (the number of values less than or equal to x). Implement the data structures and algorithms to support these operations. That is, implement the method track(int x), which is called when each number is generated, and the method getRankOfNumber(int x), which returns the number of values less than or equal to x (notincluding x itself).EXAMPLEStream(in order of appearance):5, 1, 4, 4, 5, 9, 7, 13, 3getRankOfNumber(l) 0getRankOfNumber(3) 1getRankOfNumber(4) 3arrow_forwardIn Java Pleasearrow_forward
- Code it. Code with explanation.arrow_forwardImport the HashMap and Map classes from the java.util package. Within the main method, a sentence is declared as a string variable and assigned the value "This is a test sentence with no repeating words". Split the sentence into words using the split method and store the result in a String array called "words". Create a new Map called "wordFrequency" that stores each word as a key and the frequency of the word in the sentence as the corresponding value using the HashMap implementation. Use a for loop to iterate through each word in the "words" array. Within the for loop, the code should use the containsKey method to check if the word is already in the Map. If the word is already in the Map, its frequency should be incremented by 1. Use the put method to add the word as the key and the incremented frequency as the value to increment it. If the word is not in the map, add it as a new key with a frequency of 1 as its value. Outside of the for loop, use System.out.println to…arrow_forwardWrite a java program with a method that reverses a string The method must accept one parameter of type String (string to reverse), and returns every second element from the end to the beginning. For example: String: thisisstringResult is: g i t s s harrow_forward
- Write a string class. To avoid conflicts with other similarly named classes, we will call our version MyString. This object is designed to make working with sequences of characters a little more convenient and less error-prone than handling raw c-strings, (although it will be implemented as a c-string behind the scenes). The MyString class will handle constructing strings, reading/printing, and accessing characters. In addition, the MyString object will have the ability to make a full deep-copy of itself when copied. Your class must have only one data member, a c-string implemented as a dynamic array. In particular, you must not use a data member to keep track of the size or length of the MyString. This is the first part of a two part assignment. In the next assignment you will be making some refinements to the class that you create in this assignment. For example, no documentation is required this week, but full documentation will be required next week. Here is a list of the…arrow_forwardWrite a string class. To avoid conflicts with other similarly named classes, we will call our version MyString. This object is designed to make working with sequences of characters a little more convenient and less error-prone than handling raw c-strings, (although it will be implemented as a c-string behind the scenes). The MyString class will handle constructing strings, reading/printing, and accessing characters. In addition, the MyString object will have the ability to make a full deep-copy of itself when copied. Your class must have only one data member, a c-string implemented as a dynamic array. In particular, you must not use a data member to keep track of the size or length of the MyString. #include "mystring.h" #include <cctype> #include <iostream> #include <string> using namespace std; using namespace cs_mystring; void BasicTest(); void RelationTest(); void CopyTest(); MyString AppendTest(const MyString& ref, MyString val); string boolString(bool…arrow_forwardWrite a Java program that prompts a user for vehicle data and stores it in a linked list, and then sorts the list in ascending order based on miles-per-gallon and writes the sorted data to a text file, you can follow these steps: Create a class named Vehicle with private fields: make (String), model (String), and milesPerGallon (double). Include getters and setters for these fields. Implement the Comparable interface for Vehicle class and override the compareTo() method to compare vehicles based on their milesPerGallon. Create a main class (for example, VehicleDriver.java) to handle user input and perform the necessary operations. Inside the main method, create a BufferedReader object for user input. Prompt the user to enter the number of vehicle data they want to enter and store it in a variable (for example, nVehicles). Use a loop to iterate nVehicles times and prompt the user to enter make, model, and miles per gallon for each vehicle. Create Vehicle objects using the input data…arrow_forward
- Make a recursive method for factoring an integer n. First, find a factor f, then recursively factor n / f. This assignment needs a resource class and a driver class; these two classes will need to be in two separate files. The resource class will contain all of the methods and the driver class only needs to call the methods. The driver class needs to have only 5 lines of code. The code needs to be written in Java.arrow_forwardThere is a class Country that has methods getContinent() and getPopulation(). Write a function int getPopulation(List countries, String continent) that computes the total population of a given continent, given a list of all countries and the name of a continent.arrow_forwardJava. Implement a method that will return a String with an array's elements separated by spaces. The mehod will recieve three parameters: the integer array, a start index, and an end index. Both start and end are includive. The method's signature is as follows: public static String printRange(int [], int, int) - make the method public to faciliate testing - If the indexes are outside the bounds of the array, the method should return "Invalid Range." Otherwise, the method will return a String containing one integer after the other on the same line separated by one space. Example 1: Given {4,1,2,36,6}, 1, 3 as the parameters; the method should return "1 2 36" Example 2: Given {4,1,2,36,6}, 0, 7 as the parameters; the method should return "Invalid Range"arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
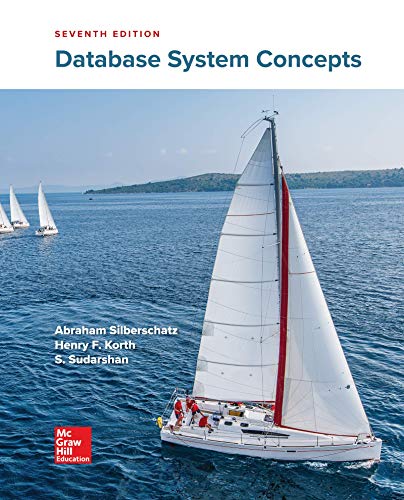
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
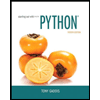
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
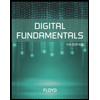
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
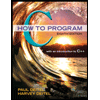
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
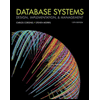
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
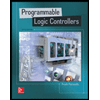
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education