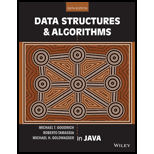
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781118771334
Author: Michael T. Goodrich
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 3, Problem 34C
Explanation of Solution
Method clone() for CircularlyLinkedList class:
Create the method clone() to create and return the duplicate copy of receiver object for CircularlyLinkedList class.
//Define the clone() method
public CircularlyLinkedList<E> clone() throws CloneNotSupportedException
{
/*Create an object for super class of CircularlyLinkedList. */
CircularlyLinkedList<E> cObj = (CircularlyLinkedList<E>) super.clone();
/*Call the clone() method and assign that element to object of CircularlyLinkedList "cObj" element...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write the same splice method for an ArrayList with member variables called data and
size and methods grow() and rangeCheck ().
Implement a recursive version of the size method for SinglyLinkedLists. (Hint: A wrapper may be useful.)
Add a void instance method replaceAll(E oldE, E newE) in the MyLinkedList class to replace the occurrence of all oldE value with the specified newE value. Implement this method in O(n) time.
import java.util.*;
public class PExamCh24 {
public static void main(String[] args) { new PExamCh24();}public PExamCh24() { // Create a list for strings MyLinkedList<String> list = new MyLinkedList<>();
// Add elements to the list list.add("America"); // Add America to the list System.out.println("(1) " + list);
list.add(0, "Japan"); // Add Canada to the beginning of the list System.out.println("(2) " + list);
list.add("Russia"); // Add Russia to the end of the list System.out.println("(3) " + list);
list.addLast("Japan"); // Add France to the end of the list System.out.println("(4) " + list);
list.add(2, "Japan"); // Add Germany to the list at index 2 System.out.println("(5) " + list);
list.add(5, "Norway"); // Add Norway to the list…
Chapter 3 Solutions
Data Structures and Algorithms in Java
Ch. 3 - Prob. 1RCh. 3 - Write a Java method that repeatedly selects and...Ch. 3 - Prob. 3RCh. 3 - The TicTacToe class of Code Fragments 3.9 and 3.10...Ch. 3 - Prob. 5RCh. 3 - Prob. 6RCh. 3 - Prob. 7RCh. 3 - Prob. 8RCh. 3 - Prob. 9RCh. 3 - Prob. 10R
Ch. 3 - Prob. 11RCh. 3 - Prob. 12RCh. 3 - Prob. 13RCh. 3 - Prob. 14RCh. 3 - Prob. 15RCh. 3 - Prob. 16RCh. 3 - Prob. 17CCh. 3 - Prob. 18CCh. 3 - Prob. 19CCh. 3 - Give examples of values for a and b in the...Ch. 3 - Suppose you are given an array, A, containing 100...Ch. 3 - Write a method, shuffle(A), that rearranges the...Ch. 3 - Suppose you are designing a multiplayer game that...Ch. 3 - Write a Java method that takes two...Ch. 3 - Prob. 25CCh. 3 - Prob. 26CCh. 3 - Prob. 27CCh. 3 - Prob. 28CCh. 3 - Prob. 29CCh. 3 - Prob. 30CCh. 3 - Prob. 31CCh. 3 - Prob. 32CCh. 3 - Prob. 33CCh. 3 - Prob. 34CCh. 3 - Prob. 35CCh. 3 - Write a Java program for a matrix class that can...Ch. 3 - Write a class that maintains the top ten scores...Ch. 3 - Prob. 38PCh. 3 - Write a program that can perform the Caesar cipher...Ch. 3 - Prob. 40PCh. 3 - Prob. 41PCh. 3 - Prob. 42PCh. 3 - Prob. 43P
Knowledge Booster
Similar questions
- For the AVLTree class, create a deletion function that makes use of lazy deletion.There are a number of methods you can employ, but one that is straightforward is to merely include a Boolean field in the Node class that indicates whether or not the node is designated for elimination. Then, your other approaches must take into consideration this field.arrow_forwardGive an implementation of the size() method for a DoublyLinkedLIst class, assuming that we did not maintain size as an instance variablearrow_forwardConsider the List interface's add (i, x) and remove (i) methods. When i is big (say n-2 or n-1), which of the following is true with regards to asymptotic runtime (ignoring amortization/resize)? the ArrayDeque and (doubly) LinkedList are equal, and faster than the SkiplistList the ArrayDeque, (doubly) LinkedList, and SkiplistList are all equal the (doubly) LinkedList is faster than the ArrayDeque which is faster than the SkiplistList the ArrayDeque is faster than the (doubly) LinkedList which is faster than the SkiplistList the ArrayDeque is faster than the SkiplistList, which is faster than the (doubly) LinkedListarrow_forward
- Write and test an efficient Java/Python method for reversing a doubly linked list L using only a constant amount of additional space. Hint: Add the method to DoublyLinkedList class.arrow_forwardWrite a program to test various operations of the class doublyLinkedList.arrow_forwardMake a doubly linked DoubleOrderedList class implementation. A DoubleNode class, DoubleList class, and DoubleIterator class must all be created.arrow_forward
- Design and implement a getLastHalf() method for the MyLinkedList class. The method should return the last half of the list as a new list leaving the original list intact. If the list has an uneven number of elements the extra one element must be in the list returned. Example : [1,3,5,4] return [5,4] and [1,6,9] return [6,9]. Follow the three step method for designing the method Develop the java code for the method Create a test program to test the method thoroughly using the Integer wrapper class.arrow_forwardDesign and implement a getLastHalf() method for the MyLinkedList class. The method should return the last half of the list as a new list leaving the original list intact. If the list has an uneven number of elements the extra one element must be in the list returned. Example : [1,3,5,4] return [5,4] and [1,6,9] return [6,9]. Follow the three step method for designing the method Develop the java code for the method Create a test program to test the method thoroughly using the Integer wrapper class. public class MyLinkedList<E> { private Node<E> head, tail; public MyLinkedList() { head = null; tail = null; } /** Return the head element in the list */ public E getFirst() { if (head == null) { return null; } else { return head.element; } } /** Return the last element in the list */ public E getLast() { if (head==null) { return null; } else { return tail.element; } } /** Add an element to the beginning of the list */ public void prepend(E e) { Node<E> newNode = new…arrow_forwardDesign and implement a getLastHalf() method for the MyLinkedList class. The method should return the last half of the list as a new list leaving the original list intact. If the list has an uneven number of elements the extra one element must be in the list returned. Example : [1,3,5,4] return [5,4] and [1,6,9] return [6,9]. Follow the three step method for designing the method Develop the java code for the method Create a test program to test the method thoroughly using the Integer wrapper class.arrow_forward
- Write the Java code for implementing the add method (based on index) of ArrayList class.arrow_forwardImplement the insert function in SLList class to insert an item x at a particular position in the list indexed by i. For example, let L= [1,2,3]. A call toL.insert (4,1) will make L=[1,4,2,3]. You should not change the item of existing nodes. Below is a skeleton of the Node and SLList class: template class Node public: ItemType item; Node *next; Node (ItemIype i, Node *n = nullptr) { item - i; next = n; } ; template class SLList { private: /** Pointer pointing to the sentinel node. */ Node *sentinel; /** Stores the current size of the list. */ int count; public: // Other functions omitted... void insert (const Item &x, int i) { // Copy this function in the answer and write code below this line. } };arrow_forwardFor both ArrayList and LinkedList, which methods do not exist in the List interface? Just why do you think these methods are left out the List?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
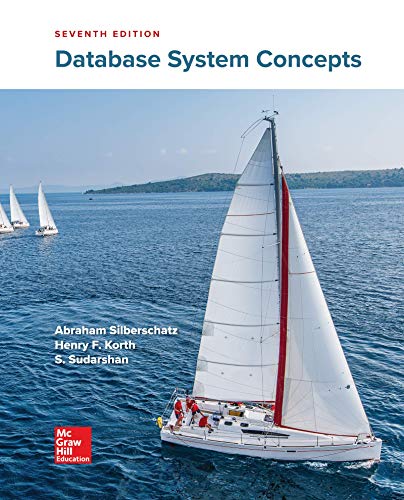
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
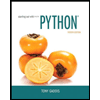
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
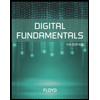
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
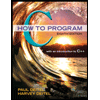
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
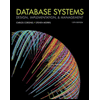
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
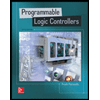
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education