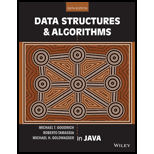
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781118771334
Author: Michael T. Goodrich
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 3, Problem 28C
Explanation of Solution
Method reverse()in singly linked list:
/*Define the reverse() method to reverse the elements from singly linked list. */
public void reverse()
{
//Create node and assign it as "null"
Node<E> prevNode = null;
//Create node and assign it as "head"
Node<E> hNode = head;
//Check whether the "hNode" is not equal to "null"
while (hNode != null)
{
//Get next node of head and assign it into "temp"
Node<E> temp = hNode.getNext();
/*Set next node of head is assigned with "prevNode". */
hNode...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Solve this
can I have the solution for this question in Java coding please?
Write an algorithm for the following to swap two adjacent elements by adjusting only the links (and not the data) using:
Singly-linked lists.
Doubly linked lists.
Implement a function to insert a node at the end of a doubly linked list. Explain the steps involved and analyze the time complexity of your implementation.
Chapter 3 Solutions
Data Structures and Algorithms in Java
Ch. 3 - Prob. 1RCh. 3 - Write a Java method that repeatedly selects and...Ch. 3 - Prob. 3RCh. 3 - The TicTacToe class of Code Fragments 3.9 and 3.10...Ch. 3 - Prob. 5RCh. 3 - Prob. 6RCh. 3 - Prob. 7RCh. 3 - Prob. 8RCh. 3 - Prob. 9RCh. 3 - Prob. 10R
Ch. 3 - Prob. 11RCh. 3 - Prob. 12RCh. 3 - Prob. 13RCh. 3 - Prob. 14RCh. 3 - Prob. 15RCh. 3 - Prob. 16RCh. 3 - Prob. 17CCh. 3 - Prob. 18CCh. 3 - Prob. 19CCh. 3 - Give examples of values for a and b in the...Ch. 3 - Suppose you are given an array, A, containing 100...Ch. 3 - Write a method, shuffle(A), that rearranges the...Ch. 3 - Suppose you are designing a multiplayer game that...Ch. 3 - Write a Java method that takes two...Ch. 3 - Prob. 25CCh. 3 - Prob. 26CCh. 3 - Prob. 27CCh. 3 - Prob. 28CCh. 3 - Prob. 29CCh. 3 - Prob. 30CCh. 3 - Prob. 31CCh. 3 - Prob. 32CCh. 3 - Prob. 33CCh. 3 - Prob. 34CCh. 3 - Prob. 35CCh. 3 - Write a Java program for a matrix class that can...Ch. 3 - Write a class that maintains the top ten scores...Ch. 3 - Prob. 38PCh. 3 - Write a program that can perform the Caesar cipher...Ch. 3 - Prob. 40PCh. 3 - Prob. 41PCh. 3 - Prob. 42PCh. 3 - Prob. 43P
Knowledge Booster
Similar questions
- Given the head of a singly linked list of integers, write the function to arrange the elements such thatall the even numbers are placed after all the odd numbers. The relative order of the odd and eventerms should remain unchanged.Input Format:Elements of linked listOutput Format:Resultant linked listSample Input 1:1 4 5 2Sample Output 1:1 5 4 2Sample Input 2:1 11 3 6 8 0 9Sample Output 2:1 11 3 9 6 8 0 in javaarrow_forwardIN C LANGUAGE an implementation of the Sequence ADT using a singly linked list. Dont use dynamic / fixed-length arrays. Some differences include: it can be empty its size can grow and shrink items can be inserted at any positionfor example, if your sequence is [42,10,27,31,99] and you insert 28 at index 2, your new sequence is [42,10,28,27,31,99]. similarly, items can be removed from any position You may modify the sequence structure to add extra fields as needed.arrow_forwardA C++ program to add 5 nodes in a linked list. Now add values of first 2 nodes and subtract values of last two nodes and then add both resultants and make its placement at the value of 3rd Node. Note: solve as soon as possible explain by double line commentsarrow_forward
- Java Programarrow_forwardWrite a program which should implement a linear linked list. Elements of this linked list should be of float type, user will provide values as input for elements of this linked list. Your program should allow deletion of a value of fist and last node of link list.arrow_forwardPython Implement a singly linked list with the following functions: - add_head(e) - add_tail(e) - find_3rd_to_last() - returns element located at third-to-last in the list - reverse() - reveres the linked list, note, this is not just printing elements in reverse order, this is actually reversing the listarrow_forward
- Java Design and draw a method called check() to check if characters in a linked list is a palindrome or not e.g "mom" or "radar" or "racecar. spaces are ignored, we can call the spaces the “separator”. The method should receive the separator as a variable which should be equal to “null” when no separator is used.arrow_forwardImplement a circular singly linked list in C programming language. Create functions for the ff: 1. Transversal 2. Insertion of element (at the beginning, in between nodes, and at the end) 3. Deletion of element 4. Search 5. Sortarrow_forwardThe add method of the sorted array-based list implementation takes O(N) time because searching in the array takes O(N) time. True Falsearrow_forward
- Write a program which should implement a linear linked list. Elements of this linked list should be of integer type, user will provide values as input for elements of this linked list. Your program should allow searching of a value specified by user in linked list and display also it index.arrow_forwardCreate an implementation of singly linked list using classes with minimum 5 nodes (spectre, togusa, yuri, siren, shepherd) in Python with the following capabilities/functions: Traverse - print out all data from the linked list Insert - generate a node and attach to an existing linked list Search - find an item (data) from the linked list and return the node Remove - remove a node from the linked listarrow_forwardThere are two n-element arrays A and B the task is to permute them such that A[i]+b[i]>=k Can someone walk me through the code. I dont understand the iList and jList and why we have if j and i not in List we do List.append. Also at the end why we do if len(iList)+len(jList)==len(A)+len(B) ? def twoArrays (k, A, B): A.sort() B. sort() iList = [] jList = [] for i in range (len (A)): for j in range (len (B)): if(A[i]+B[j]>=k): if j not in jList and i not in iList: iList.append(i) jList.append(j) print (iList) print (jList) if(len (iList) + len(jList) ==len (A) + len(B)) return "YES" else: return "NO"arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
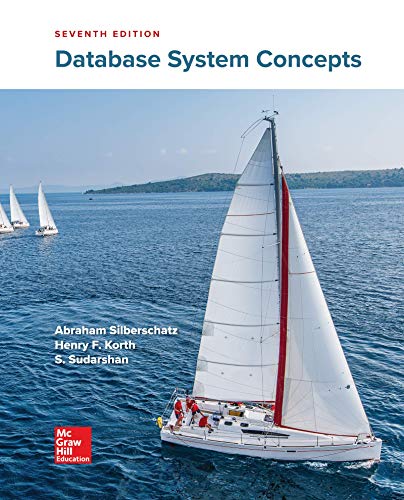
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
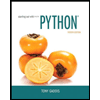
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
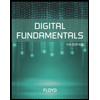
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
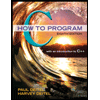
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
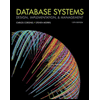
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
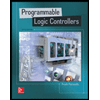
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education