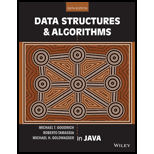
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781118771334
Author: Michael T. Goodrich
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 3, Problem 9R
Explanation of Solution
Method size() in Singularly linked list:
// Define the size() method
public int size()
{
// Declare and initialize a variable
int counter=0;
// Create a node to hold the head value
Node<E> node = head;
/* Loop executes until the "node" is not equal to "null" */
while (node != null)
{
// Increment "counter" variable by "1"
counter++;
/* Next node is determined by getNext() and assigned to "node" variable.*/
node = node...
Expert Solution & Answer

Trending nowThis is a popular solution!
Learn your wayIncludes step-by-step video

schedule03:03
Students have asked these similar questions
We have been given a vehicle class (Image 1) that is a domain class in java and we want to create a vehicle service class named VehicleArrayIListlmpl (Image 2). The Service
class will store multiple domain objects, support the implementation of the domain class, and include a java collection array list. Use java to create the VehicleArraylListImpl, with
appropriate methods (based on the class diagram)
Business rule - When adding a Vehicle, confirm the Vehicle is not present. Confirm the Owner does exist. If either does not pass, print a message to the console and stop the
add. Use the isPresent method you created above
VehicleArrayListImpl
dava Enumeration
OvehicleClassification
f
carShows
List
main
VANTIQUE VehicleClossification
VCLASSC VehicleClessification
VuoCERN Vehicieclassification
fvenicieCiassificationo
m VehicleArrayListImpl()
m add(Vehicle)
boolean
1.1
m dump()
m find(String)
void
cjava Classa
@Wehicle
Vehicle
main
vehicleD: String
a pwnero String
e manufacturer String
a…
For the AVLTree class, create a deletion function that makes use of lazy deletion.There are a number of methods you can employ, but one that is straightforward is to merely include a Boolean field in the Node class that indicates whether or not the node is designated for elimination. Then, your other approaches must take into consideration this field.
Consider the Person class in Problem 1. Implement the interface PersonPriorityQueueInterface provided in the assignment. In your implementation, you must use an instance of AList (which you used in Problem 1) to store the list of persons.
We consider that a person whose age is higher than a second person also has a higher priority. Thus, the method peek(), for example, should return the person who is the oldest in the list.
Your implementation should be O(n) for add, and O(1) for the remaining methods.
Consider the Person class in Problem 1. Implement the interface PersonPriorityQueueInterface provided in the assignment. In your implementation, you must use an instance of AList (which you used in Problem 1) to store the list of persons.
We consider that a person whose age is higher than a second person also has a higher priority. Thus, the method peek(), for example, should return the person who is the oldest in the list.
Your implementation should be O(n) for add, and O(1)…
Chapter 3 Solutions
Data Structures and Algorithms in Java
Ch. 3 - Prob. 1RCh. 3 - Write a Java method that repeatedly selects and...Ch. 3 - Prob. 3RCh. 3 - The TicTacToe class of Code Fragments 3.9 and 3.10...Ch. 3 - Prob. 5RCh. 3 - Prob. 6RCh. 3 - Prob. 7RCh. 3 - Prob. 8RCh. 3 - Prob. 9RCh. 3 - Prob. 10R
Ch. 3 - Prob. 11RCh. 3 - Prob. 12RCh. 3 - Prob. 13RCh. 3 - Prob. 14RCh. 3 - Prob. 15RCh. 3 - Prob. 16RCh. 3 - Prob. 17CCh. 3 - Prob. 18CCh. 3 - Prob. 19CCh. 3 - Give examples of values for a and b in the...Ch. 3 - Suppose you are given an array, A, containing 100...Ch. 3 - Write a method, shuffle(A), that rearranges the...Ch. 3 - Suppose you are designing a multiplayer game that...Ch. 3 - Write a Java method that takes two...Ch. 3 - Prob. 25CCh. 3 - Prob. 26CCh. 3 - Prob. 27CCh. 3 - Prob. 28CCh. 3 - Prob. 29CCh. 3 - Prob. 30CCh. 3 - Prob. 31CCh. 3 - Prob. 32CCh. 3 - Prob. 33CCh. 3 - Prob. 34CCh. 3 - Prob. 35CCh. 3 - Write a Java program for a matrix class that can...Ch. 3 - Write a class that maintains the top ten scores...Ch. 3 - Prob. 38PCh. 3 - Write a program that can perform the Caesar cipher...Ch. 3 - Prob. 40PCh. 3 - Prob. 41PCh. 3 - Prob. 42PCh. 3 - Prob. 43P
Knowledge Booster
Similar questions
- Give the names of the two methods that we have to have to implement interface Iterator as shown in RED below.arrow_forwardFor this exercise, we are going to revisit the Pie superclass that we saw as an example in the last lesson. We have added the Pumpkin class, which takes the number of slices and a boolean to indicate if it is made with canned pumpkin. We also added a getType() method in the Pie class. For this exercise, you need to create either an Array or an ArrayList and add 3 pies to that list, an Apple, a Pumpkin, and a third type using the Pie class. After adding these to your list, loop through and print out the type using the getType() method in the pie class. Sample Output Pie: Pumpkin Pie: Apple Pie: Blueberryarrow_forwardFor this exercise, we are going to revisit the Pie superclass that we saw as an example in the last lesson. We have added the Pumpkin class, which takes the number of slices and a boolean to indicate if it is made with canned pumpkin. We also added a getType() method in the Pie class. For this exercise, you need to create either an Array or an ArrayList and add 3 pies to that list, an Apple, a Pumpkin, and a third type using the Pie class. After adding these to your list, loop through and print out the type using the getType() method in the pie class. ---------------------------------------------- import java.util.ArrayList; public class PieTester{public static void main(String[] args){// Start here!}} ---------------------------------------------- public class PumpkinPie extends Pie {private boolean canned; public PumpkinPie (int slices, boolean canned) {super("Pumpkin", slices);this.canned = canned;}public boolean canned(){return canned;}}…arrow_forward
- There’s a somewhat imperfect analogy between a linked list and a railroad train, where individual cars represent links. Imagine how you would carry out various linked list operations, such as those implemented by the member functions insertFirst(), removeFirst(), and remove(int key) from the LinkList class in this hour. Also implement an insertAfter() function. You’ll need some sidings and switches. You can use a model train set if you have one. Otherwise, try drawing tracks on a piece of paper and using business cards for train cars.arrow_forwardUse java and indent the code properly.arrow_forwardProgramming in Java. What would the difference be in the node classes for a singly linked list, doubly linked list, and a circular linked list? I attached the node classes I have for single and double, but I feel like I do not change enough? Also, I use identical classes for singular and circular node which does not feel right. Any help would be appreciated.arrow_forward
- I am trying to practice Java Programming and would like to see and example of a program so I can better understand ArrayList using generics, Asymptotics, Searching, and Sorting. All variables should be inaccessible from other classes and must require an instance to be accessed through, unless specified otherwise. All methods should be accessible from everywhere and must require an instance to be accessed through, unless specified otherwise. Make sure to reuse code when applicable. I only one part of the full program I am writing from you. This is for practice so I can better understand some areas I am having trouble with. Thank you Cake.java This class will describe a certain kind of dessert that Bob can pick: cakes. This class extends the Dessert class.Variables:• String frosting – what the frosting of the cake isConstructor(s):• A constructor that takes in flavor, sweetness, and frosting of the cake (in this order).• A constructor that takes in the flavor and sets the sweetness to…arrow_forwardI am trying to practice Java Programming and would like to see and example of a program so I can better understand ArrayList using generics, Asymptotics, Searching, and Sorting. All variables should be inaccessible from other classes and must require an instance to be accessed through, unless specified otherwise. All methods should be accessible from everywhere and must require an instance to be accessed through, unless specified otherwise. Make sure to reuse code when applicable. I only one part of the full program I am writing from you. This is for practice so I can better understand some areas I am having trouble with. Thank you Store.java This will be the class that stores the data for the dessert store. We are assuming that a store can sell all kinds of dessert.Variables:• String name – name of the store.• ArrayList desserts – an ArrayList of type DessertConstructor(s):• A constructor that only takes in name and creates an empty ArrayList of type Dessert.Methods:•…arrow_forwardI am trying to practice Java Programming and would like to see and example of a program so I can better understand ArrayList using generics, Asymptotics, Searching, and Sorting. All variables should be inaccessible from other classes and must require an instance to be accessed through, unless specified otherwise. All methods should be accessible from everywhere and must require an instance to be accessed through, unless specified otherwise. Make sure to reuse code when applicable. I only one part of the full program I am writing from you. This is for practice so I can better understand some areas I am having trouble with. Thank you IceCream.Java This class will describe a certain kind of dessert that Bob can pick: ice cream. Write this class so Bob can have ice cream. This class will have to extend the Dessert class.Variables:• int scoops – the number of scoops of ice cream you get• boolean cone – represents if the ice cream has a coneConstructor(s):• A constructor that takes in…arrow_forward
- Develop classes STint and STdouble for maintaining ordered symbol tables where keys are primitive int and double types, respectively. (Convert genericsto primitive types in the code of RedBlackBST.) Test your solution with a version ofSparseVector as a client.arrow_forwardFor this Problem, you will implement a brute force solution to the maximum-subarray problem that runs in O(n2) time (i.e., your solutions should have two nested loops). You will implement this solution for an array of ints and a simple LinkedList of ints. To get started, import the two starter files into the subarray package you created in a new Java Project. Please do not change any of the method signatures or any code in the provided LinkedList class. Implement the two methods described below. public LinkedList findMaximumSubList(LinkedList nums) This method should return a new LinkedList that represents the maximum sublist of the given LinkedList, nums. For example, the maximum sublist of 13->-3->-25->-20->-3->-16->-23->18->20->-7->12->-5->-22->15->-4->7 is 18->20->-7->12 public int[] findMaximumSubArray(int[] nums) This method should return a new int[] that represents the maximum subarray of the given int[], nums. For example,…arrow_forwardWrite a generic method filter() that receives two arrays (in and out) from any type that implements the Comparable interface and a parameter e of the same type. All elements of in array will be add to out array unless the value of the element is greeter then e.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
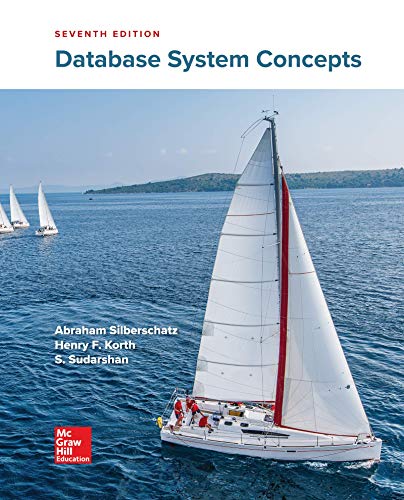
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
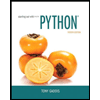
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
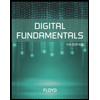
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
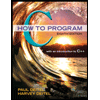
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
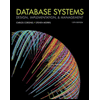
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
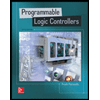
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education