JAVA Create a new class HashMapManipulation with a main method. Import the necessary classes from the java.util package, including the HashMap class. Create a HashMap object, named mapand use the put() method to add key-value pairs to the map. The keys are strings "A", "B", and "C", and the values are integers 1, 2, and 3 respectively. Use the size() method to print the size of the map. Use a for loop to print the key-value pairs in the map. The loop should iterate over the entries in the map using the entrySet() method, which will return a set of Map.Entry objects representing the key-value pairs in the map. The key and value of each entry are printed using the getKey() and getValue() methods, respectively. Use theget() method to get the value of key "A", and assigns it to a variable value. The value is then printed. Use the put() method to add a new key-value pair to the map. The key is "D" and the value is 4. The map is then printed again, to show the added key-value pair. Use the containsKey() method to check if the key "C" exists in the map and print the result of the method.
JAVA
-
Create a new class HashMapManipulation with a main method.
-
Import the necessary classes from the java.util package, including the HashMap class.
-
Create a HashMap object, named mapand use the put() method to add key-value pairs to the map. The keys are strings "A", "B", and "C", and the values are integers 1, 2, and 3 respectively.
-
Use the size() method to print the size of the map.
-
Use a for loop to print the key-value pairs in the map. The loop should iterate over the entries in the map using the entrySet() method, which will return a set of Map.Entry objects representing the key-value pairs in the map. The key and value of each entry are printed using the getKey() and getValue() methods, respectively.
-
Use theget() method to get the value of key "A", and assigns it to a variable value. The value is then printed.
-
Use the put() method to add a new key-value pair to the map. The key is "D" and the value is 4. The map is then printed again, to show the added key-value pair.
-
Use the containsKey() method to check if the key "C" exists in the map and print the result of the method.

Step by step
Solved in 4 steps with 2 images

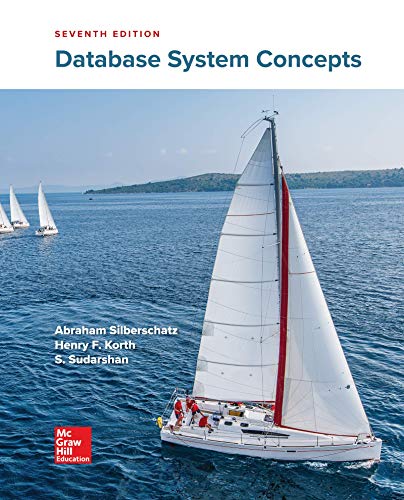
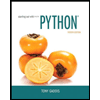
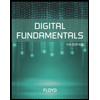
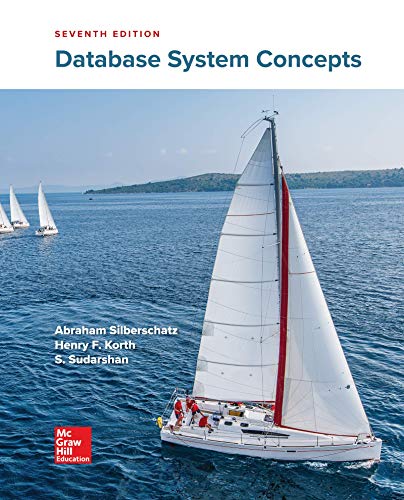
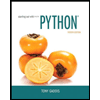
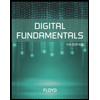
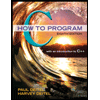
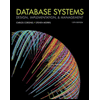
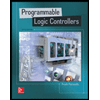