I have a code for an assignment, but the code isn't working please help me. The code of the resource class: import java.util.ArrayList; import java.util.Collections; import java.util.Scanner; /** A table for lookups and reverse lookups */ public class U10E08R { private ArrayList people; /** Constructs a LookupTable object. */ public U10E08R() { people = new ArrayList(); } /** Reads key/value pairs. @param in the scanner for reading the input */ public void read(Scanner in) { while(in.hasNext()){ String name = in.nextLine(); String number = in.nextLine(); people.add(new U10E08Item(name, number)); } } /** Looks up an item in the table. @param k the key to find @return the value with the given key, or null if no such item was found. */ public String lookup(String k) { String output = null; for(U10E08Item item: people){ if(k.equals(item.getName())){ output = item.getNumber(); } } return output; } /** Looks up an item in the table. @param v the value to find @return the key with the given value, or null if no such item was found. */ public String reverseLookup(String v) { String output = null; for(U10E08Item item: people){ if(v.equals(item.getNumber())){ output = item.getName(); } } return output; } } The code of the Item class: public class U10E08Item { private String name, number; public U10E08Item(String aName, String aNumber){ name = aName; number = aNumber; } public String getName(){ return name; } public String getNumber(){ return number; } The code of the driver class: import java.io.FileReader; import java.io.IOException; import java.util.Scanner; public class U10E08D { public static void main(String[] args) throws IOException { Scanner in = new Scanner(System.in); System.out.println("Enter the name of the phonebook file: "); String fileName = in.nextLine(); U10E08R table = new U10E08R(); FileReader reader = new FileReader(fileName); table.read(new Scanner(reader)); boolean more = true; while (more) { System.out.println("Lookup N)ame, P)hone number, Q)uit?"); String cmd = in.nextLine(); if (cmd.equalsIgnoreCase("Q")) more = false; else if (cmd.equalsIgnoreCase("N")) { System.out.println("Enter name:"); String n = in.nextLine(); System.out.println("Phone number: " + table.lookup(n)); } else if (cmd.equalsIgnoreCase("P")) { System.out.println("Enter phone number:"); String n = in.nextLine(); System.out.println("Name: " + table.reverseLookup(n)); } } } } The errors are shown in the picture I attached
I have a code for an assignment, but the code isn't working please help me.
The code of the resource class:
import java.util.ArrayList;
import java.util.Collections;
import java.util.Scanner;
/**
A table for lookups and reverse lookups
*/
public class U10E08R
{
private ArrayList<U10E08Item> people;
/**
Constructs a LookupTable object.
*/
public U10E08R()
{
people = new ArrayList<U10E08Item>();
}
/**
Reads key/value pairs.
@param in the scanner for reading the input
*/
public void read(Scanner in)
{
while(in.hasNext()){
String name = in.nextLine();
String number = in.nextLine();
people.add(new U10E08Item(name, number));
}
}
/**
Looks up an item in the table.
@param k the key to find
@return the value with the given key, or null if no
such item was found.
*/
public String lookup(String k)
{
String output = null;
for(U10E08Item item: people){
if(k.equals(item.getName())){
output = item.getNumber();
}
}
return output;
}
/**
Looks up an item in the table.
@param v the value to find
@return the key with the given value, or null if no
such item was found.
*/
public String reverseLookup(String v)
{
String output = null;
for(U10E08Item item: people){
if(v.equals(item.getNumber())){
output = item.getName();
}
}
return output;
}
}
The code of the Item class:
public class U10E08Item {
private String name, number;
public U10E08Item(String aName, String aNumber){
name = aName;
number = aNumber;
}
public String getName(){
return name;
}
public String getNumber(){
return number;
}
The code of the driver class:
import java.io.FileReader;
import java.io.IOException;
import java.util.Scanner;
public class U10E08D
{
public static void main(String[] args) throws IOException
{
Scanner in = new Scanner(System.in);
System.out.println("Enter the name of the phonebook file: ");
String fileName = in.nextLine();
U10E08R table = new U10E08R();
FileReader reader = new FileReader(fileName);
table.read(new Scanner(reader));
boolean more = true;
while (more)
{
System.out.println("Lookup N)ame, P)hone number, Q)uit?");
String cmd = in.nextLine();
if (cmd.equalsIgnoreCase("Q"))
more = false;
else if (cmd.equalsIgnoreCase("N"))
{
System.out.println("Enter name:");
String n = in.nextLine();
System.out.println("Phone number: " + table.lookup(n));
}
else if (cmd.equalsIgnoreCase("P"))
{
System.out.println("Enter phone number:");
String n = in.nextLine();
System.out.println("Name: " + table.reverseLookup(n));
}
}
}
}
The errors are shown in the picture I attached
![import java.io.FileReader;
import java.io.I0Exception;
import java.util.Scanner;
public class U10E08D
{
public static void main(String[] args) throws IOException
{
Scanner in = new Scanner(System.in);
System.out.println("Enter the name of the phonebook file: ");
String fileName = in.nextLine();
U10E08R table = new U10E08R ();
FileReader reader = new FileReader(fileName);
table.read(new Scanner(reader));
boolean more = true;
while (more)
{
System.out.println("Lookup N) ame, P)hone number, Q)uit?");
String cmd = in.nextline();
GU10E03R.java
G U10E02R.java
GU10E02D.java
g U10E03D.java
GU10P01R.java
GU10P1D.java
E U10E08R.java
C PhoneLookup
Compile Messages
JGRASP Messages
Run I/O
Interactions
----JGRASP wedge2: exit code for process is 1.
----JGRASP: operation complete.
End
Clear
----JGRASP exec: java U10E08D
Enter the name of the phonebook file:
Help
demo.txt
Exception in thread "main" java.io.FileNotFoundException: demo.txt (The system cannot find the file specified)
at java.base/java.io.FileInputstream.open@(Native Method)
at java.base/java.io.FileInputStream.open(FileInputStream.java:211)
at java.base/java.io.FileInputStream.<init>(FileInputStream.java:153)
at java.base/java.io.FileInputStream.<init>(FileInputStream.java:108)
at java.base/java.io.FileReader.<init>(FileReader.java:60)
at U10E08D.main(U10E08D.java:15)
----JGRASP wedge2: exit code for process is 1.
----JGRASP: operation complete.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9662a84a-bfe1-4836-aca1-8bd4583109d9%2F15a3b426-4a64-4419-bf19-c92b3b4bb635%2Fnc5rmgh_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps with 1 images

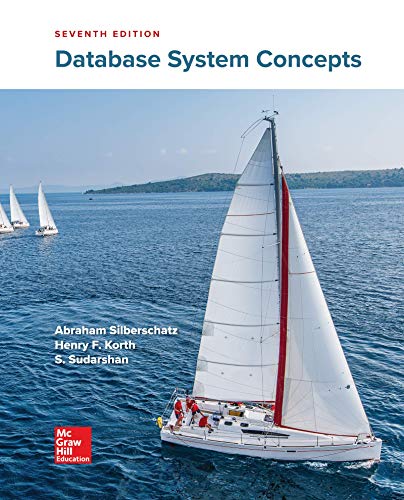
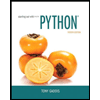
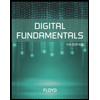
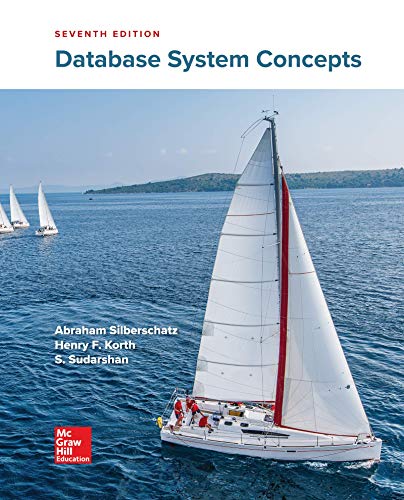
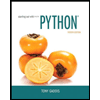
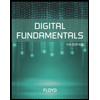
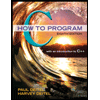
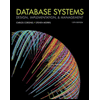
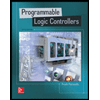