Create a java class called ArrayOperations. Add the static methods described below. Then write a test class to verify that all of your methods work. Static Methods for ArrayOperations The lcass name is ArrayOperations It has one static method called findSum. This method returns the sum of the elements in an array To invoke this method from another class, we use the class name (ArrayOperations.findSum(MyArray)); toString : This method signature should be: public static String toString(int[] anArray). It should return a String that consists of all elements in the array. For example if the input array consists of {1, 5, 9, 2}, then toString should return the string 1 5 9 2 findMaxMin : The signature for this method should be public static int[] findMaxMin(int[] anArray) Notice that the method returns an array of int – not a single value. In this method Write code to declare an array of int with two elements – say limits Find the largest element in the array and store it in limits[0] Find the smallest element in the array and store it in limits[1] return limits findAverage: The signature for this method should be public static double findAverage(int[] myArray) In the implementation, we simply need to compute the sum of the array elements and divide them by the length of the array. We can do this by using findSum and dividing by the array length. The test program ArrayOperationsTest The test class should conform to the following specifications: The name of the class is ArrayOperationsTest The test class should contain a static method called makeArray The test class should contain a static method called menu that offers the user the following choices Make a new array (ask the user for the number of elements) Calculate and print the sum of the array elements Find and print max and min values in the array Print the toString for the array Calculate and print the average value in the array QUIT The program should have a main method that works as follows Display the menu and get user choice Call the appropriate method(s) to do what the user needs and display the results Loop until the user selects QUIT Summary ArrayOperations has static methods findSum, findAverage, findVariance, and findMaxMin. ArrayOperationsTest has methods makeArray, menu, and main. You will need to import Scanner and Random. A sample run might consist of Creating an array with 10 elements Printing the sum of the array elements Printing the max and min values in the array Printing the average value for the array Printing the variance for the array Printing the toString for the array
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Create a java class called ArrayOperations. Add the static methods described below. Then write a test class to verify that all of your methods work.
Static Methods for ArrayOperations
- The lcass name is ArrayOperations
- It has one static method called findSum. This method returns the sum of the elements in an array
- To invoke this method from another class, we use the class name (ArrayOperations.findSum(MyArray));
- toString : This method signature should be: public static String toString(int[] anArray). It should return a String that consists of all elements in the array. For example if the input array consists of {1, 5, 9, 2}, then toString should return the string 1 5 9 2
- findMaxMin : The signature for this method should be public static int[] findMaxMin(int[] anArray) Notice that the method returns an array of int – not a single value. In this method
- Write code to declare an array of int with two elements – say limits
- Find the largest element in the array and store it in limits[0]
- Find the smallest element in the array and store it in limits[1]
- return limits
- findAverage: The signature for this method should be public static double findAverage(int[] myArray)
In the implementation, we simply need to compute the sum of the array elements and divide them by the length of the array. We can do this by using findSum and dividing by the array length.
The test program ArrayOperationsTest
The test class should conform to the following specifications:
- The name of the class is ArrayOperationsTest
- The test class should contain a static method called makeArray
- The test class should contain a static method called menu that offers the user the following choices
- Make a new array (ask the user for the number of elements)
- Calculate and print the sum of the array elements
- Find and print max and min values in the array
- Print the toString for the array
- Calculate and print the average value in the array
- QUIT
- The program should have a main method that works as follows
- Display the menu and get user choice
- Call the appropriate method(s) to do what the user needs and display the results
- Loop until the user selects
- QUIT
Summary
ArrayOperations has static methods findSum, findAverage, findVariance, and findMaxMin.
ArrayOperationsTest has methods makeArray, menu, and main. You will need to import Scanner and Random.
A sample run might consist of
- Creating an array with 10 elements
- Printing the sum of the array elements
- Printing the max and min values in the array
- Printing the average value for the array
- Printing the variance for the array
- Printing the toString for the array
Unlock instant AI solutions
Tap the button
to generate a solution
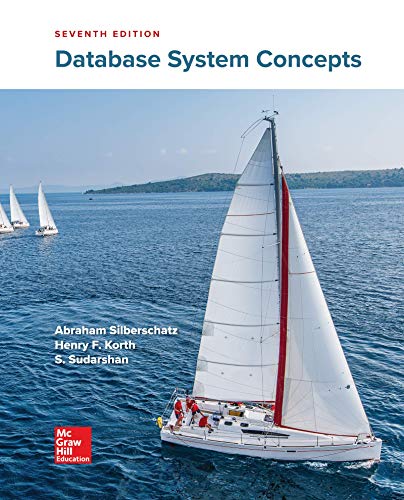
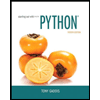
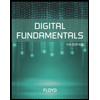
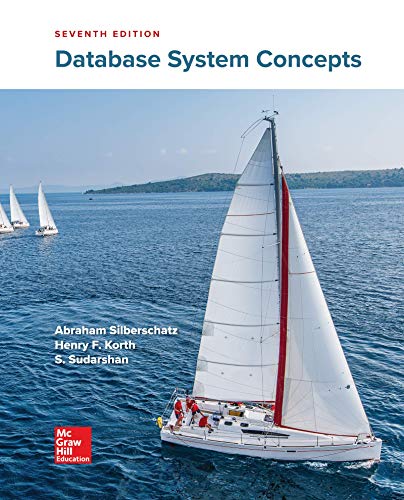
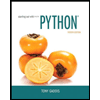
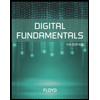
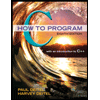
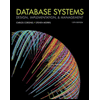
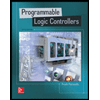