Code these three methods.
Code these three methods.
![public class TwoDArrayMethods
{
/* Method #1
* Write a static method named matrixAdd that accepts a pair of two-dimensional arrays of
integers as parameters,
treats the arrays as 2D matrices and adds them, returning the result.
The sum of two matrices A and B is a matrix C where
for every row i and column j, Cij
* You may assume that the arrays passed as parameters have the same dimensions.
* /
Aij + Bij.
/* Method #2
* Write a static method called loadArray that takes in a 2D array of integers and will
fill
* the array with values from 1 to the number of elements in the array in reverse
row
order.
* This call to loadArray(some2DArray), where some2DArray is a 2D array of integers that
is 3x4
* would result in some2DArray containing the values:
*
4 3 2 1
8 7 6 5
12 11 10 9
* /
/* Method 3
* Write a method called allRowSums that takes in a 2D array of integers and calculates
the row sum for every row.
Index i of the return array contains the sum of elements in row i.
The method should return each of the sums in an array.
Example: allRowSums ({1, 2, 3},
{ 4, 5, 6})
→ [6, 15]
*
public static void main(String args[])
{
//Test all three of your methods to ensure they work!](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fee043644-8c82-4555-bd39-471becdabd6c%2F930ec9f4-abff-4f2d-b9ea-7801761c0969%2F5cfr88b_processed.png&w=3840&q=75)

1 st method
public int[][] matrixAdd(int[][] a, int[][] b) {
for(int i = 0; i < a.length; i++) {
for(int j = 0; j < a[0].length; j++) {
a[i][j] = a[i][j] + b[i][j];
}
}
return a;
}
or
public class MatrixAdditionExample{
public static void main(String args[]){
//creating two matrices
int a[][]={{1,3,4},{2,4,3},{3,4,5}};
int b[][]={{1,3,4},{2,4,3},{1,2,4}};
//creating another matrix to store the sum of two matrices
int c[][]=new int[3][3]; //3 rows and 3 columns
//adding and printing addition of 2 matrices
for(int i=0;i<3;i++){
for(int j=0;j<3;j++){
c[i][j]=a[i][j]+b[i][j]; //use - for subtraction
System.out.print(c[i][j]+" ");
}
System.out.println();//new line
}
}}
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

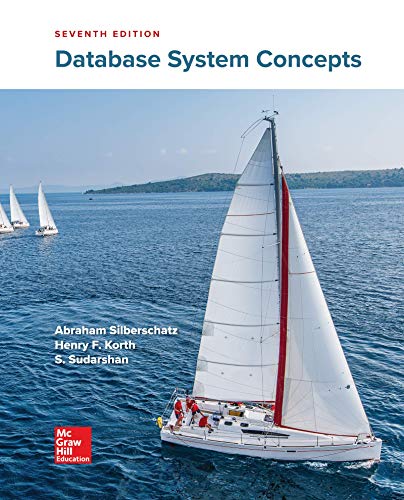
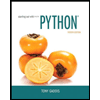
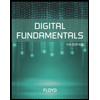
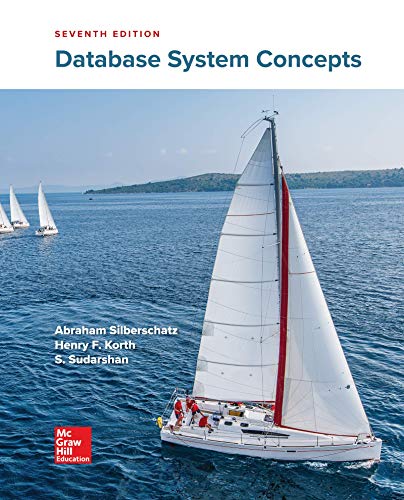
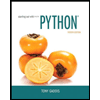
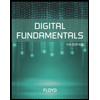
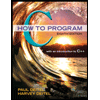
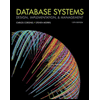
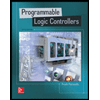