Add a method findMiddle() that finds the middle node of a doubly linked list by link hopping, without relying on explicit knowledge of the size of the list. Counting nodes is not the correct way to do this. In the case of an even number of nodes, report the node slightly left of center as the middle. You can assume the list has at least one item. Your findMiddle() code will be located in DoublyLinkedList.java. If you’re using size(), you’re doing it wrong. Starter code for the findMiddle() method: // Janet McGregor 4/20/2053 public E findMiddle() { Node middleNode = header.next; Node currentNode = header.next; // the rest of your code goes here return middleNode.element; } You will test findMiddle() with the following three tests cases. Sample program output: Finding the middle of the list: (1, 2, 3, 4, 5, 6, 7) The middle value is: 4 Finding the middle of this list: (1, 2, 3, 4) The middle value is: 2 Finding the middle of this list: (First, Second, Third, Fourth, Fifth)
Add a method findMiddle() that finds the middle node of a doubly linked list by link hopping, without relying on explicit knowledge of the size of the list. Counting nodes is not the correct way to do this.
In the case of an even number of nodes, report the node slightly left of center as the middle.
You can assume the list has at least one item.
Your findMiddle() code will be located in DoublyLinkedList.java. If you’re using size(), you’re doing it wrong.
Starter code for the findMiddle() method:
// Janet McGregor 4/20/2053 public E findMiddle() { Node<E> middleNode = header.next; Node<E> currentNode = header.next;
// the rest of your code goes here return middleNode.element; } |
You will test findMiddle() with the following three tests cases. Sample program output:
Finding the middle of the list:
(1, 2, 3, 4, 5, 6, 7)
The middle value is: 4
Finding the middle of this list:
(1, 2, 3, 4)
The middle value is: 2
Finding the middle of this list:
(First, Second, Third, Fourth, Fifth)
The middle value is: Third

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 5 images

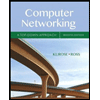
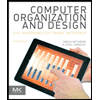
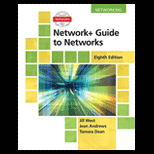
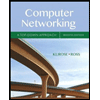
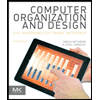
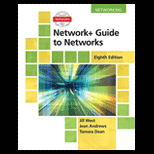
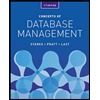
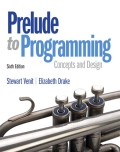
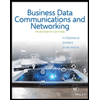