Implement a lastIndex method for the LinkedIntList class you worked on for HW4. This method takes an int argument and returns the largest index such that the int argument appears at that index in the list. If the int argument does not appear in the list, then -1 is returned. For example if the list example contained the numbers [4, -1, 2, 7, -1, 3] in that order, then example.lastIndex(-1) should return 4 and example.lastIndex(5) should return -1. In the text area below, write the definition of the lastIndex method. (In other words, write the code that should replace the //TODO comment.) Your code cannot call any methods. public class LinkedIntList { private class Node { private int item; private Node next; public Node() {} public Node(int number, Node nextNode) { item = number; next = nextNode; } } private Node first; // first node of the list public LinkedIntList() { first = null; } /* Other LinkedIntList methods not listed here for the sake of space */ public int lastIndex(int target) { // TODO } }
Implement a lastIndex method for the LinkedIntList class you worked on for HW4. This method takes an int argument and returns the largest index such that the int argument appears at that index in the list. If the int argument does not appear in the list, then -1 is returned. For example if the list example contained the numbers [4, -1, 2, 7, -1, 3] in that order, then example.lastIndex(-1) should return 4 and example.lastIndex(5) should return -1.
In the text area below, write the definition of the lastIndex method. (In other words, write the code that should replace the //TODO comment.) Your code cannot call any methods.

Solution:
There is no //TODO comment in the code mentioned in comment
Hence, you can insert lastIndex function code after LinkedList Constructor.
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

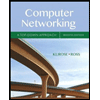
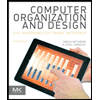
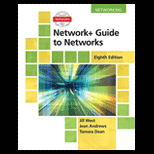
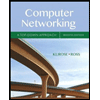
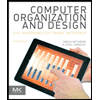
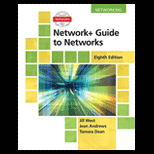
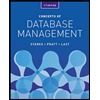
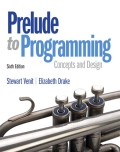
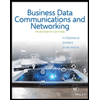