How to get insert method to accept two values? Right now it accepts one, and ignores the other. class Node: """ Represents a node in a linked list """ def __init__(self, data): self.data = data self.next = None class LinkedList: """ A linked list implementation of the List ADT """ def __init__(self): self._head = None def add(self, val): """ Adds a node containing val to the linked list """ self._head = self.rec_add(self._head, val) def rec_add(self, a_node, val): """ Adds a node containing val to the linked list """ if a_node is None: return Node(val) else: a_node.next = self.rec_add(a_node.next, val) return a_node def insert(self, val, pos): """ Adds a node containing val to the linked list """ self._head = self.insert_add(self._head, val, pos) def insert_add(self, a_node, val, pos): """ Adds a node containing val to the linked list """ if a_node is None: return Node(val) if pos == 0: n = Node(val) n.next = a_node return n else: a_node.next = self.insert_add(a_node.next, val, pos-1) return a_node def remove(self, val): """ Removes the node containing val from the linked list """ self._head = self.rec_remove(self._head, val) def rec_remove(self, a_node, val): """ Removes a node containing val to the linked list """ if a_node is None: return a_node elif a_node.val == val: return a_node.next else: a_node.next = self.rec_remove(a_node.next, val) return a_node def contains(self, val): return self.rec_contains(self._head, val) def rec_contains(self, a_node, val): """ Removes a node containing val to the linked list """ if a_node is None: return False if a_node.data == val: return True return self.rec_contains(a_node.next, val) def is_empty(self): """ Returns True if the linked list is empty, returns False otherwise """ return self._head is None def rec_display(self, a_node): """recursive display method""" if a_node is None: return print(a_node.data, end=" ") self.rec_display(a_node.next) def display(self): """recursive display helper method""" self.rec_display(self._head) print() def reverse(self): self._head = self.rec_reverse(self._head) def rec_reverse(self, node): if node is None or node.next is None: return node nextNode = node.next remaining = self.rec_reverse(node.next) nextNode.next = node node.next = None return remaining def to_regular_list (self): result = [] self.rec_to_regular_list(self._head, result) return result def rec_to_regular_list (self, node, result): if node is None: return result result.append(node.data) return self.rec_to_regular_list(node.next, result) l = LinkedList() l.add(1) l.add(2) l.add(3) l.add(4) l.add(5) l.display() l.insert(7, 2) l.display() l.insert(7, 12) l.display() print(l.is_empty()) print(l.contains(2)) print(l.contains(20)) l.reverse() l.display() print(l.to_regular_list())
How to get insert method to accept two values? Right now it accepts one, and ignores the other.
class Node:
""" Represents a node in a linked list """
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
""" A linked list implementation of the List ADT """
def __init__(self):
self._head = None
def add(self, val):
""" Adds a node containing val to the linked list """
self._head = self.rec_add(self._head, val)
def rec_add(self, a_node, val):
""" Adds a node containing val to the linked list """
if a_node is None:
return Node(val)
else:
a_node.next = self.rec_add(a_node.next, val)
return a_node
def insert(self, val, pos):
""" Adds a node containing val to the linked list """
self._head = self.insert_add(self._head, val, pos)
def insert_add(self, a_node, val, pos):
""" Adds a node containing val to the linked list """
if a_node is None:
return Node(val)
if pos == 0:
n = Node(val)
n.next = a_node
return n
else:
a_node.next = self.insert_add(a_node.next, val, pos-1)
return a_node
def remove(self, val):
""" Removes the node containing val from the linked list """
self._head = self.rec_remove(self._head, val)
def rec_remove(self, a_node, val):
""" Removes a node containing val to the linked list """
if a_node is None:
return a_node
elif a_node.val == val:
return a_node.next
else:
a_node.next = self.rec_remove(a_node.next, val)
return a_node
def contains(self, val):
return self.rec_contains(self._head, val)
def rec_contains(self, a_node, val):
""" Removes a node containing val to the linked list """
if a_node is None:
return False
if a_node.data == val:
return True
return self.rec_contains(a_node.next, val)
def is_empty(self):
""" Returns True if the linked list is empty, returns False otherwise """
return self._head is None
def rec_display(self, a_node):
"""recursive display method"""
if a_node is None:
return
print(a_node.data, end=" ")
self.rec_display(a_node.next)
def display(self):
"""recursive display helper method"""
self.rec_display(self._head)
print()
def reverse(self):
self._head = self.rec_reverse(self._head)
def rec_reverse(self, node):
if node is None or node.next is None:
return node
nextNode = node.next
remaining = self.rec_reverse(node.next)
nextNode.next = node
node.next = None
return remaining
def to_regular_list (self):
result = []
self.rec_to_regular_list(self._head, result)
return result
def rec_to_regular_list (self, node, result):
if node is None:
return result
result.append(node.data)
return self.rec_to_regular_list(node.next, result)
l = LinkedList()
l.add(1)
l.add(2)
l.add(3)
l.add(4)
l.add(5)
l.display()
l.insert(7, 2)
l.display()
l.insert(7, 12)
l.display()
print(l.is_empty())
print(l.contains(2))
print(l.contains(20))
l.reverse()
l.display()
print(l.to_regular_list())

Step by step
Solved in 2 steps

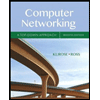
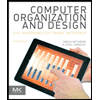
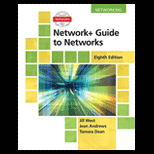
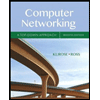
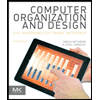
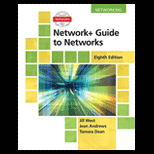
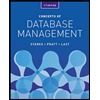
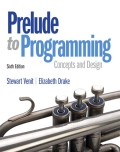
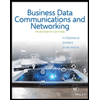