Linked List, create your own code. (Do not use the build in function or classes of Java or from the textbook). Create a LinkedList class: Call the class MyLinkedList, (hint) Create a second class called Node.java and use it, remember in the class I put the Node class inside the LinkedList Class, but you should do it outside. This class should have o Variables you may need for a Node, o (optional) Constructor Your linked list is of an int type. (you may do it as General type as ) For this Linked List you need to have the following methods: add, addAfter, remove, size, contain, toString, compare, addInOrder. This is just a suggestion, if you use Generic type, you must modify this Write a main function or Main class to test all the methods, o Create a 2 linked list and test all your methods. (Including the compare)
Linked List, create your own code. (Do not use the build in function or classes of Java or from the textbook).
Create a LinkedList class: Call the class MyLinkedList,
(hint) Create a second class called Node.java and use it, remember in the class I put the Node class inside the LinkedList Class, but you should do it outside. This class should have
o Variables you may need for a Node,
o (optional) Constructor
Your linked list is of an int type. (you may do it as General type as <E>)
For this Linked List you need to have the following methods:
add, addAfter, remove, size, contain, toString, compare, addInOrder.
This is just a suggestion, if you use Generic type, you must modify this
Write a main function or Main class to test all the methods,
o Create a 2 linked list and test all your methods. (Including the compare)


Step by step
Solved in 5 steps

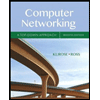
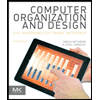
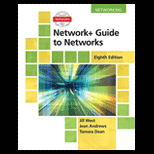
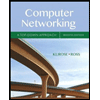
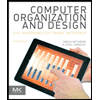
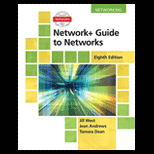
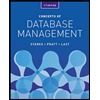
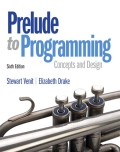
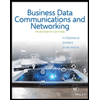