In Java please help with the following: Sees whether this list is empty. @return True if the list is empty, or false if not. */ public boolean isEmpty(); } // end ListInterface Hint: Node class definition should look something like: public class Node { T element; Node next; Node prev; public Node(T element, Node next, Node prev) { this.element = element; this.next = next; this.prev = prev; } }
In Java please help with the following: Sees whether this list is empty. @return True if the list is empty, or false if not. */ public boolean isEmpty(); } // end ListInterface Hint: Node class definition should look something like: public class Node { T element; Node next; Node prev; public Node(T element, Node next, Node prev) { this.element = element; this.next = next; this.prev = prev; } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In Java please help with the following:
Sees whether this list is empty.
@return True if the list is empty, or false if not. */
public boolean isEmpty(); } // end ListInterface
Hint:
Node class definition should look something like:
public class Node<T> { T element; Node next; Node prev; |
public Node(T element, Node next, Node prev) { this.element = element; } } |

Transcribed Image Text:1. Implement List ADT using Doubly Linked List.
Create a class DoubleLinkedList that implements both Doublelterable interface ListInterface interfaces:
public interface DoubleIterator<T>
{
/** Detects whether this iterator has completed its traversal
and gone beyond the last entry in the collection of data.
@return True if the iterator has another entry to return. */
public boolean hasNext ();
/** Retrieves the next entry in the collection and
advances this iterator by one position.
@return A reference to the next entry in the iteration,
if one exists.
@throws NoSuchElementException if the iterator had reached the
end already, that is, if hasNext () is false. */
public T next ();
/** Detects whether this iterator has completed its back traversal
and gone below the first entry in the collection of data.
@return True if the iterator has a prior entry to return. */
public boolean hasPrior ();
/ ** Retrieves the prior entry in the collection and
advances this iterator by one position.
@return A reference to the prior entry in the iteration,
if one exists.
@throws NoSuchElementException if the iterator had reached the
end already, that is, if hasPrior() is false. */
public T prior ();
} // end Iterator
public interface DoubleIterable<T>
{
/** @return A doubleIterator for a collection of objects of type T.
* /
DoubleIterator<T> iterator();
} // end DoubleIterable
public interface ListInterface<T> {
/** Adds a new entry to the end of this list.
Entries currently in the list are unaffected.
The list's size is increased by 1.
@param newEntry
public void add(T newEntry);
The object to be added as a
new entry. */
![/** Adds a new entry at a specified position within this list.
Entries originally at and above the specified position
are at the next higher position within the list.
The list's size is increased by 1.
@param newPosition
An integer that specifies the desired
position of the new entry.
The object to be added as a new entry.
@param newEntry
@throws
IndexOutOfBoundsException if either
newPosition < 1 or newPosition > getLength ( )
+ 1. */
public void add(int newPosition, T newEntry);
/** Removes the entry at a given position from this list.
Entries originally at positions higher than the given
position are at the next lower position within the list,
and the list's size is decreased by 1.
@param givenPosition
An integer that indicates the position of
the entry to be removed.
@return
A reference to the removed entry.
@ throws
IndexOutOfBoundsException if either
givenPosition < 1 or givenPosition > getLength(). * /
public T remove (int givenPosition);
/** Removes all entries from this list. */
public void clear();
/** Replaces the entry at a given position in this list.
@param givenPosition An integer that indicates the position of
the entry to be replaced.
@param newEntry
The object that will replace the entry at the
position givenPosition.
@return
The original entry that was replaced.
IndexOutOfBoundsException if either
givenPosition < 1 or givenPosition > getLength(). */
@throws
public T replace(int givenPosition, T newEntry) ;
/** Retrieves the entry at a given position in this list.
@param givenPosition
An integer that indicates the position of
the desired entry.
A reference to the indicated entry.
IndexOutOfBoundsException if either
givenPosition < 1 or givenPosition > getLength (). * /
@return
@throws
public T getEntry(int givenPosition);
/** Retrieves all entries that are in this list in the order in which
they occur in the list.
@return
A newly allocated array of all the entries in the list.
If the list is empty, the returned array is empty. */
public T[] toArray();
/** Sees whether this list contains a given entry.
@param anEntry
@return
The object that is the desired entry.
True if the list contains anEntry, or false if not. */
public boolean contains (T anEntry);
/** Gets the length of this list.
@return
The integer number of entries currently in the list. * /
public int getLength() ;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb44b9793-0ad6-40fb-a2b1-d5aa6c545792%2Fc83e75e4-0cb8-485b-a43c-553877b0eaac%2Ffg06rfh_processed.png&w=3840&q=75)
Transcribed Image Text:/** Adds a new entry at a specified position within this list.
Entries originally at and above the specified position
are at the next higher position within the list.
The list's size is increased by 1.
@param newPosition
An integer that specifies the desired
position of the new entry.
The object to be added as a new entry.
@param newEntry
@throws
IndexOutOfBoundsException if either
newPosition < 1 or newPosition > getLength ( )
+ 1. */
public void add(int newPosition, T newEntry);
/** Removes the entry at a given position from this list.
Entries originally at positions higher than the given
position are at the next lower position within the list,
and the list's size is decreased by 1.
@param givenPosition
An integer that indicates the position of
the entry to be removed.
@return
A reference to the removed entry.
@ throws
IndexOutOfBoundsException if either
givenPosition < 1 or givenPosition > getLength(). * /
public T remove (int givenPosition);
/** Removes all entries from this list. */
public void clear();
/** Replaces the entry at a given position in this list.
@param givenPosition An integer that indicates the position of
the entry to be replaced.
@param newEntry
The object that will replace the entry at the
position givenPosition.
@return
The original entry that was replaced.
IndexOutOfBoundsException if either
givenPosition < 1 or givenPosition > getLength(). */
@throws
public T replace(int givenPosition, T newEntry) ;
/** Retrieves the entry at a given position in this list.
@param givenPosition
An integer that indicates the position of
the desired entry.
A reference to the indicated entry.
IndexOutOfBoundsException if either
givenPosition < 1 or givenPosition > getLength (). * /
@return
@throws
public T getEntry(int givenPosition);
/** Retrieves all entries that are in this list in the order in which
they occur in the list.
@return
A newly allocated array of all the entries in the list.
If the list is empty, the returned array is empty. */
public T[] toArray();
/** Sees whether this list contains a given entry.
@param anEntry
@return
The object that is the desired entry.
True if the list contains anEntry, or false if not. */
public boolean contains (T anEntry);
/** Gets the length of this list.
@return
The integer number of entries currently in the list. * /
public int getLength() ;
Expert Solution

Step 1
We have to write the program in java that implements both DoubleIterable Interface and ListInterface Interface
Step by step
Solved in 2 steps with 3 images

Recommended textbooks for you
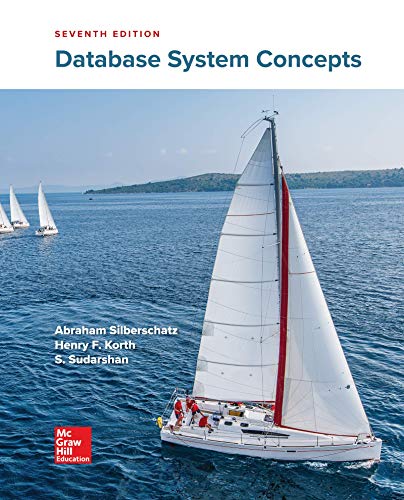
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
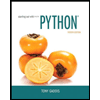
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
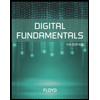
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
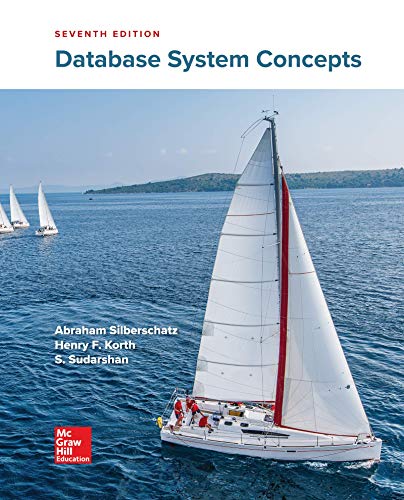
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
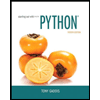
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
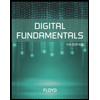
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
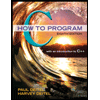
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
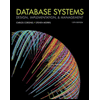
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
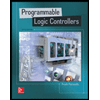
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education