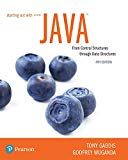
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 21, Problem 4PC
Program Plan Intro
Prefix Calculator
Program plan:
- Include necessary header files.
- Define the class “PreficCalc”.
- Declare the necessary variables.
- Define the method “start()”.
- Create labels and text fields to enter the prefix expression.
- Create a button “evaluate” to submit and calculate the resultant value.
- Define the class “CmdTextListener”.
- Define the method “handle()”.
- Declare the string variable to get a prefix expression.
- Declare the method “buildExpr()” to read and build a binary tree.
- Declare the method “eval()” to evaluate the tree expression.
- Display the result into the resultant text field.
- Define the method “eval()” to evaluate the expression.
-
- The “if” statement check, left and right child of tree is equal to null.
- True, return the integer value.
- Otherwise,
- Call the method “eval()” and assign the result into “leftOp”.
- Call the method “eval()” and assign the result into “rightOp”.
- The “if” statement check, tree value is equal to “*”.
- True, return the multiplied value.
- The “if” statement check, tree value is equal to “+”.
- True, return the added value.
- The “if” statement check, tree value is equal to “+”.
- True, return the multiplied value.
- Return “0”.
- Define the method “isNumber()”.
- If the given expression is number then, return the number itself.
- Define the method “isNumber()”.
- Define the class “Node”.
- Declare the necessary variables.
- Define the parameterized constructor.
- Define the method “buildExpr()” to read and build a binary tree.
- Read the input from the user.
- The “if” statement check the expression is number.
- True, return the node itself.
- Otherwise, build a non-leaf tree.
- Call the method “Node()” and return a value.
- Otherwise, build a non-leaf tree.
- True, return the node itself.
- The “if” statement check, left and right child of tree is equal to null.
- Define the method “handle()”.
- Define the “main()” method.
- Declare the method “launch()” to call the “start()” method eventually.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
We are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7).
A code breaker manages to factories 247 = 13 x 19
Determine Alice's secret key.
To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.
Consider the following Turing Machine (TM). Does the TM halt if it begins on the empty tape? If it halts, after how many steps? Does the TM halt if it begins on a tape that contains a single letter A followed by blanks? Justify your answer.
Pllleasassseee ssiiirrrr soolveee thissssss questionnnnnnn
Chapter 21 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 21.1 - Prob. 21.2CPCh. 21.1 - Prob. 21.3CPCh. 21 - Prob. 1MCCh. 21 - Prob. 2MCCh. 21 - Prob. 3MCCh. 21 - Prob. 4MCCh. 21 - Prob. 5MCCh. 21 - Prob. 6MCCh. 21 - Prob. 7MCCh. 21 - Prob. 8MC
Ch. 21 - Prob. 9MCCh. 21 - Prob. 10MCCh. 21 - Prob. 11TFCh. 21 - Prob. 12TFCh. 21 - Prob. 13TFCh. 21 - Prob. 14TFCh. 21 - Prob. 15TFCh. 21 - Prob. 16TFCh. 21 - Prob. 17TFCh. 21 - Prob. 18TFCh. 21 - Prob. 19TFCh. 21 - Prob. 20TFCh. 21 - Prob. 21TFCh. 21 - Prob. 1FTECh. 21 - Prob. 2FTECh. 21 - Prob. 3FTECh. 21 - Prob. 1SACh. 21 - Prob. 2SACh. 21 - Prob. 3SACh. 21 - Prob. 4SACh. 21 - What is a priority queue?Ch. 21 - Prob. 6SACh. 21 - Prob. 7SACh. 21 - Prob. 1AWCh. 21 - Prob. 2AWCh. 21 - Prob. 3AWCh. 21 - Prob. 4AWCh. 21 - Prob. 5AWCh. 21 - Prob. 6AWCh. 21 - Prob. 7AWCh. 21 - Prob. 4PCCh. 21 - Prob. 6PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forwardOf the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization? Part A - Define each primary component of the information system. Part B - Include your perspective on why your selection is most important. Part C - Provide an example from your personal experience to support your answer.arrow_forward
- Management Information Systemsarrow_forwardQ2/find the transfer function C/R for the system shown in the figure Re དarrow_forwardPlease original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forward
- Please original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forwardWhat are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
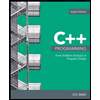
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning