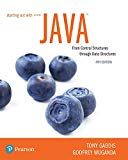
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 21, Problem 4AW
Explanation of Solution
Binary search tree:
- Binary search tree is a tree in which the nodes are sorted in the semantic order and it has the shape of binary tree.
- Nodes in the binary search tree can have zero, one, or two children.
- In a binary search tree, any node value is greater than the left sub tree and lesser than the right sub tree.
- Node without children is called a leaf or end node.
- A node that does not have a superior node is called a root node.
- Root node is the starting node.
- The binary search will be performed until finding a search node or reaching the end of the tree.
Step 1: Define a function named “leafCount” with the parameter “btree”.
Step 2: Check if “btree” is equal to null. If it is true, then return 0.
Step 3: Check if “btree.left” is equal to null and “btree.right” is equal to null. If this condition is true, then return 1.
Step 4: If both the condition fails, then return the summation of count of left tree and right tree by calling the function “leafCount ()” recursively...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
We are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7).
A code breaker manages to factories 247 = 13 x 19
Determine Alice's secret key.
To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.
Consider the following Turing Machine (TM). Does the TM halt if it begins on the empty tape? If it halts, after how many steps? Does the TM halt if it begins on a tape that contains a single letter A followed by blanks? Justify your answer.
Pllleasassseee ssiiirrrr soolveee thissssss questionnnnnnn
Chapter 21 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 21.1 - Prob. 21.2CPCh. 21.1 - Prob. 21.3CPCh. 21 - Prob. 1MCCh. 21 - Prob. 2MCCh. 21 - Prob. 3MCCh. 21 - Prob. 4MCCh. 21 - Prob. 5MCCh. 21 - Prob. 6MCCh. 21 - Prob. 7MCCh. 21 - Prob. 8MC
Ch. 21 - Prob. 9MCCh. 21 - Prob. 10MCCh. 21 - Prob. 11TFCh. 21 - Prob. 12TFCh. 21 - Prob. 13TFCh. 21 - Prob. 14TFCh. 21 - Prob. 15TFCh. 21 - Prob. 16TFCh. 21 - Prob. 17TFCh. 21 - Prob. 18TFCh. 21 - Prob. 19TFCh. 21 - Prob. 20TFCh. 21 - Prob. 21TFCh. 21 - Prob. 1FTECh. 21 - Prob. 2FTECh. 21 - Prob. 3FTECh. 21 - Prob. 1SACh. 21 - Prob. 2SACh. 21 - Prob. 3SACh. 21 - Prob. 4SACh. 21 - What is a priority queue?Ch. 21 - Prob. 6SACh. 21 - Prob. 7SACh. 21 - Prob. 1AWCh. 21 - Prob. 2AWCh. 21 - Prob. 3AWCh. 21 - Prob. 4AWCh. 21 - Prob. 5AWCh. 21 - Prob. 6AWCh. 21 - Prob. 7AWCh. 21 - Prob. 4PCCh. 21 - Prob. 6PC
Knowledge Booster
Similar questions
- 4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forwardOf the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization? Part A - Define each primary component of the information system. Part B - Include your perspective on why your selection is most important. Part C - Provide an example from your personal experience to support your answer.arrow_forward
- Management Information Systemsarrow_forwardQ2/find the transfer function C/R for the system shown in the figure Re དarrow_forwardPlease original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forward
- Please original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forwardWhat are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
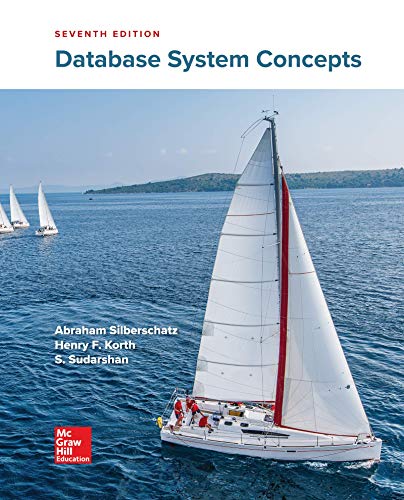
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
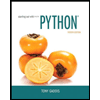
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
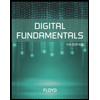
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
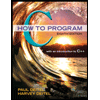
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
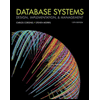
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
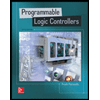
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education