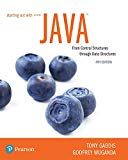
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 21.1, Problem 21.2CP
Program Plan Intro
Postorder traversal:
It process all the nodes of the tree in the following order.
- First, traverse all the nodes in the left subtree.
- Then, traverse all the nodes in the right subtree.
- Finally, traverse the root node.
Method “postorder” to print the values stored in a binary tree is as follows:
Node postorder(Node bTree)
{
//Check tree is not empty
if (tree != null)
{
//Print value of left subtree
postorder(bTree.left);
//Print value of right subtree
postorder(bTree.right);
//Print the root node value
System.out.print(bTree.value + " ");
}
}
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Given the schema below for the widgetshop, provide a
schema diagram.
Schema name
Attributes
Widget-schema
Customer-schema
(stocknum, manufacturer, description, weight,
price, inventory)
(custnum, name, address)
Purchased-schema (custnum, stocknum, pdate)
Requestedby-schema (stocknum, custnum)
Newitem-schema (stocknum, manufacturer, description)
Employee-schema (ssn, name, address, salary)
You can remove the Newitem-schema (red).
True or False: Given the sets F and G with F being an element of G, is it always ture that P(F) is an element of P(G)? (P(F) and P(G) mean power sets). Why?
Can you please simplify (the domain is not empty) ∃xF (x) → ¬∃x(F (x) ∨ ¬G(x)). Fo
Chapter 21 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 21.1 - Prob. 21.2CPCh. 21.1 - Prob. 21.3CPCh. 21 - Prob. 1MCCh. 21 - Prob. 2MCCh. 21 - Prob. 3MCCh. 21 - Prob. 4MCCh. 21 - Prob. 5MCCh. 21 - Prob. 6MCCh. 21 - Prob. 7MCCh. 21 - Prob. 8MC
Ch. 21 - Prob. 9MCCh. 21 - Prob. 10MCCh. 21 - Prob. 11TFCh. 21 - Prob. 12TFCh. 21 - Prob. 13TFCh. 21 - Prob. 14TFCh. 21 - Prob. 15TFCh. 21 - Prob. 16TFCh. 21 - Prob. 17TFCh. 21 - Prob. 18TFCh. 21 - Prob. 19TFCh. 21 - Prob. 20TFCh. 21 - Prob. 21TFCh. 21 - Prob. 1FTECh. 21 - Prob. 2FTECh. 21 - Prob. 3FTECh. 21 - Prob. 1SACh. 21 - Prob. 2SACh. 21 - Prob. 3SACh. 21 - Prob. 4SACh. 21 - What is a priority queue?Ch. 21 - Prob. 6SACh. 21 - Prob. 7SACh. 21 - Prob. 1AWCh. 21 - Prob. 2AWCh. 21 - Prob. 3AWCh. 21 - Prob. 4AWCh. 21 - Prob. 5AWCh. 21 - Prob. 6AWCh. 21 - Prob. 7AWCh. 21 - Prob. 4PCCh. 21 - Prob. 6PC
Knowledge Booster
Similar questions
- HistogramUse par(mfrow=c(2,2)) and output 4 plots with different argument settings.arrow_forward(use R language)Scatter plot(a). Run the R code example, and look at the help file for plot() function. Try different values for arguments:type, pch, lty, lwd, col(b). Use par(mfrow=c(3,2)) and output 6 plots with different argument settings.arrow_forward1. Draw flow charts for each of the following;a) A system that reads three numbers and prints the value of the largest number.b) A system reads an employee name (NAME), overtime hours worked (OVERTIME), hours absent(ABSENT) and determines the bonus payment (PAYMENT).arrow_forward
- Scenario You work for a small company that exports artisan chocolate. Although you measure your products in kilograms, you often get orders in both pounds and ounces. You have decided that rather than have to look up conversions all the time, you could use Python code to take inputs to make conversions between the different units of measurement. You will write three blocks of code. The first will convert kilograms to pounds and ounces. The second will convert pounds to kilograms and ounces. The third will convert ounces to kilograms and pounds. The conversions are as follows: 1 kilogram = 35.274 ounces 1 kilogram = 2.20462 pounds 1 pound = 0.453592 kilograms 1 pound = 16 ounces 1 ounce = 0.0283 kilograms 1 ounce = 0.0625 pounds For the purposes of this activity the template for a function has been provided. You have not yet covered functions in the course, but they are a way of reusing code. Like a Python script, a function can have zero or more parameters. In the code window you…arrow_forwardmake a screen capture showing the StegExpose resultsarrow_forwardWhich of the following is not one of the recommended criteria for strategic objectives? Multiple Choice a) realistic b) appropriate c) sustainable d) measurablearrow_forward
- Management innovations such as total quality, benchmarking, and business process reengineering always lead to sustainable competitive advantage because everyone else is doing them. a) True b) Falsearrow_forwardVision statements are more specific than strategic objectives. a) True b) Falsearrow_forwardThe three components of the __________ approach to corporate accounting include financial, environmental, and social performance measures. Multiple Choice a) stakeholder b) triple dimension c) triple bottom line d) triple efficiencyarrow_forward
- Competitors, as internal stakeholders, should be included in the stakeholder management consideration of a company and in its mission statement. a) True b) Falsearrow_forwardAt what level in the organization should the strategic management perspective be emphasized? Multiple Choice a) throughout the organization b) from the bottom up in an organization c) at the top of the organization d) at the middle of the organizationarrow_forwardA good manager can be flexible when it comes to sticking to the original plan; to get good results, the intended strategy has to become the realized strategy. a) True b) Falsearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
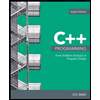
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
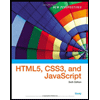
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
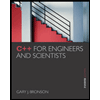
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
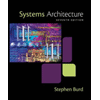
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage