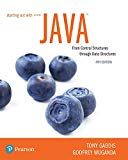
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 21.1, Problem 21.3CP
Program Plan Intro
Preorder traversal:
It process all the nodes of the tree in the following order.
- First, traverse the root node.
- Then, traverse all the nodes in the left subtree.
- Finally, traverse all the nodes in the right subtree.
Method “preorder” to print the values stored in a binary tree is as follows:
Node preorder(Node bTree)
{
//Check tree is not empty
if (tree != null)
{
//Print the root node value
System.out.print(bTree.value + " ");
//Print value of left subtree
preorder(bTree.left);
//Print value of right subtree
preorder(bTree.right);
}
}
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Problem Statement
You are working as a Devops Administrator.
Y
ou’ve been
t
asked to deploy a multi
-
tier application on Kubernetes Cluster. The application is a NodeJS application
available on Docker Hub with the following name:
d
evopsedu/emp
loyee
This Node
JS application works with a mongo database. MongoDB
image
is available
on
D
ockerHub with the following name:
m
ongo
You are required to deploy this application on Kubernetes:
•
NodeJS is available on port 8888 in the container
and will be reaching out to por
t
27017 for mongo database connection
•
MongoDB will be accepting connections on
port 27017
You must deploy this application using the CL
I
.
Once your application is up and running, ensure you can add an employee from the
NodeJS application and verify by
going to Get Employee page and retrieving your input.
Hint:
Name the Mongo DB Service and deployment, specifically as “mongo”.
I need help in server client project. It is around 1200 lines of code in both . I want to meet with the expert online because it is complicated. I want the server send a menu to the client and the client enters his choice and keep on this until the client chooses to exit . the problem is not in the connection itself as far as I know.I tried while loops but did not work. please help its emergent
I need help in my server client in C language
Chapter 21 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 21.1 - Prob. 21.2CPCh. 21.1 - Prob. 21.3CPCh. 21 - Prob. 1MCCh. 21 - Prob. 2MCCh. 21 - Prob. 3MCCh. 21 - Prob. 4MCCh. 21 - Prob. 5MCCh. 21 - Prob. 6MCCh. 21 - Prob. 7MCCh. 21 - Prob. 8MC
Ch. 21 - Prob. 9MCCh. 21 - Prob. 10MCCh. 21 - Prob. 11TFCh. 21 - Prob. 12TFCh. 21 - Prob. 13TFCh. 21 - Prob. 14TFCh. 21 - Prob. 15TFCh. 21 - Prob. 16TFCh. 21 - Prob. 17TFCh. 21 - Prob. 18TFCh. 21 - Prob. 19TFCh. 21 - Prob. 20TFCh. 21 - Prob. 21TFCh. 21 - Prob. 1FTECh. 21 - Prob. 2FTECh. 21 - Prob. 3FTECh. 21 - Prob. 1SACh. 21 - Prob. 2SACh. 21 - Prob. 3SACh. 21 - Prob. 4SACh. 21 - What is a priority queue?Ch. 21 - Prob. 6SACh. 21 - Prob. 7SACh. 21 - Prob. 1AWCh. 21 - Prob. 2AWCh. 21 - Prob. 3AWCh. 21 - Prob. 4AWCh. 21 - Prob. 5AWCh. 21 - Prob. 6AWCh. 21 - Prob. 7AWCh. 21 - Prob. 4PCCh. 21 - Prob. 6PC
Knowledge Booster
Similar questions
- Exercise docID document text docID document text 1 hot chocolate cocoa beans 7 sweet sugar 2345 9 cocoa ghana africa 8 sugar cane brazil beans harvest ghana 9 sweet sugar beet cocoa butter butter truffles sweet chocolate 10 sweet cake icing 11 cake black forest Clustering by k-means, with preprocessing tokenization, term weighting TFIDF. Manhattan Distance. Number of cluster is 2. Centroid docID 2 and docID 9.arrow_forwardChange the following code so that there is always at least one way to get from the left corner to the top right, but the labyrinth is still randomized. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. Take care that the player and the dragon cannot start off on walls. Also the dragon starts off from a randomly chosen position public class Labyrinth { private final int size; private final Cell[][] grid; public Labyrinth(int size) { this.size = size; this.grid = new Cell[size][size]; generateLabyrinth(); } private void generateLabyrinth() { Random rand = new Random(); for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { // Randomly create walls and paths grid[i][j] = new Cell(rand.nextBoolean()); } } // Ensure start and end are…arrow_forwardChange the following code so that it checks the following 3 conditions: 1. there is no space between each cells (imgs) 2. even if it is resized, the components wouldn't disappear 3. The GameGUI JPanel takes all the JFrame space, so that there shouldn't be extra space appearing in the frame other than the game. Main(): Labyrinth labyrinth = new Labyrinth(10); Player player = new Player(9, 0); Dragon dragon = new Dragon(9, 9); JFrame frame = new JFrame("Labyrinth Game"); GameGUI gui = new GameGUI(labyrinth, player, dragon); frame.add(gui); frame.setSize(600, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; //labyrinth, player, dragon are just public classes private final ImageIcon playerIcon = new ImageIcon("data/images/player.png");…arrow_forward
- Make the following game user friendly with GUI, with some simple graphics. The GUI should be in another seperate class, with some ImageIcon, and Game class should be added into the pane. The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return…arrow_forwardDiscuss the negative and positive impacts or information technology in the context of your society. Provide two references along with with your answerarrow_forwardA cylinder of diameter 10 cm rotates concentrically inside another hollow cylinder of inner diameter 10.1 cm. Both cylinders are 20 cm long and stand with their axis vertical. The annular space is filled with oil. If a torque of 100 kg cm is required to rotate the inner cylinder at 100 rpm, determine the viscosity of oil. Ans. μ= 29.82poisearrow_forward
- Make the following game user friendly with GUI, with some simple graphics The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return isWall; } public void setWall(boolean isWall) { this.isWall = isWall; } @Override public String toString() {…arrow_forwardPlease original work What are four of the goals of information lifecycle management think they are most important to data warehousing, Why do you feel this way, how dashboards can be used in the process, and provide a real life example for each. Please cite in text references and add weblinksarrow_forwardThe following is code for a disc golf program written in C++: // player.h #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(int playerId, const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H // player.cpp #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, then check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum…arrow_forward
- In this assignment, you will implement a multi-threaded program (using C/C++) that will check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome numbers are numbers that their decimal representation can be read from left to right and from right to left (e.g. 12321, 5995, 1234321). The program will create T worker threads to check for prime and palindrome numbers in the given range (T will be passed to the program with the Linux command line). Each of the threads works on a part of the numbers within the range. Your program should have some global shared variables: • numOfPrimes: which will track the total number of prime numbers found by all threads. numOfPalindroms: which will track the total number of palindrome numbers found by all threads. numOfPalindromic Primes: which will count the numbers that are BOTH prime and palindrome found by all threads. TotalNums: which will count all the processed numbers in the range. In addition, you need to have arrays…arrow_forwardHow do you distinguish between hardware and a software problem? Discuss theprocedure for troubleshooting any hardware or software problem. give one reference with your answer.arrow_forwardYou are asked to explain what a computer virus is and if it can affect computer’shardware or software. How do you protect your computer against virus? give one reference with your answer.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
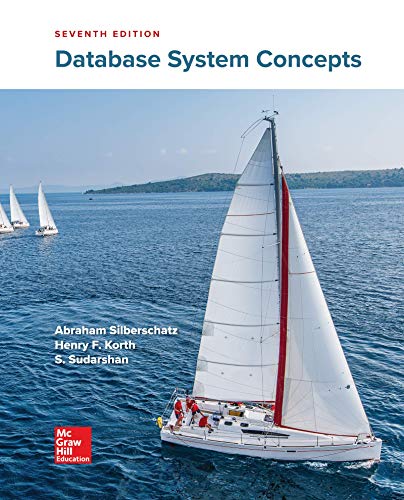
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
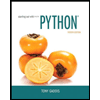
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
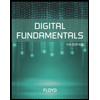
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
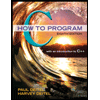
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
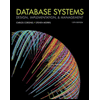
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
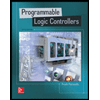
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education