UML Diagram of City Class: City - cityID: int - cityName: String - country:String - population: int +City() +City(cityID: int, cityName: String, country:String, country: String, population: int) +setCityID(cityID:int): void +getCityID():int +setCityName(cityName: String): void +getCityName(): String +setCountry(country:String): void +getCountry(): String +setPopulation (population:int): void +getPopulation():int +getCityCategory(): String +toString:String Assignment Instructions: You are tasked with developing a program to use city data from an online database and generate a city details report. 1) Create a new Project in Eclipse called "HW7". 2) Create a class "City.java" in the project and implement the UML diagram shown below and add comments to your program. 3) The logic for the method "getCityCategory" of City Class is below: a. If the population of a city is greater than 10000000, then the method returns "MEGA" b. If the population of a city is greater than 1000000 and less than 10000000, then the method returns "LARGE" c. If the population of a city is greater than 100000 and less than 1000000, then the method returns "MEDIUM" d. If the population of a city is below 100000, then the method returns "SMALL" 4) You should create another new Java program inside the project. Name the program as "xxxx_program.java”, where xxxx is your Kean username. 3) Implement the following methods inside the xxxx_program program The main method calls another method “readfromFile" which reads each line from the given csv file "worldcities.csv" - The readfromFile method will store the data in an array of objects for City class. - Create a generateReport method that generates the "CityReport.csv" file. - The headings are added as shown below in sample output. Include comments in your program Sample screenshot of the output "CityReport.csv" file 1 CityID City Name 2 1566979497 Abeokuta Country Nigeria Population City Category 888924 MEDIUM 3 1682452296 Ad DammÄm Saudi Arabia 903312 MEDIUM 4 1356304381 Ahmedabad India 7717000 LARGE 5 1566568277 Akure Nigeria 847903 MEDIUM 6 1608824345 Antipolo Philippines 887399 MEDIUM 789 1368838855 Ar RamÄdÄ<< Iraq 874543 MEDIUM 1356410365 Bangalore 1764068610 Bangkok India 13999000 MEGA Thailand 17573000 MEGA
UML Diagram of City Class: City - cityID: int - cityName: String - country:String - population: int +City() +City(cityID: int, cityName: String, country:String, country: String, population: int) +setCityID(cityID:int): void +getCityID():int +setCityName(cityName: String): void +getCityName(): String +setCountry(country:String): void +getCountry(): String +setPopulation (population:int): void +getPopulation():int +getCityCategory(): String +toString:String Assignment Instructions: You are tasked with developing a program to use city data from an online database and generate a city details report. 1) Create a new Project in Eclipse called "HW7". 2) Create a class "City.java" in the project and implement the UML diagram shown below and add comments to your program. 3) The logic for the method "getCityCategory" of City Class is below: a. If the population of a city is greater than 10000000, then the method returns "MEGA" b. If the population of a city is greater than 1000000 and less than 10000000, then the method returns "LARGE" c. If the population of a city is greater than 100000 and less than 1000000, then the method returns "MEDIUM" d. If the population of a city is below 100000, then the method returns "SMALL" 4) You should create another new Java program inside the project. Name the program as "xxxx_program.java”, where xxxx is your Kean username. 3) Implement the following methods inside the xxxx_program program The main method calls another method “readfromFile" which reads each line from the given csv file "worldcities.csv" - The readfromFile method will store the data in an array of objects for City class. - Create a generateReport method that generates the "CityReport.csv" file. - The headings are added as shown below in sample output. Include comments in your program Sample screenshot of the output "CityReport.csv" file 1 CityID City Name 2 1566979497 Abeokuta Country Nigeria Population City Category 888924 MEDIUM 3 1682452296 Ad DammÄm Saudi Arabia 903312 MEDIUM 4 1356304381 Ahmedabad India 7717000 LARGE 5 1566568277 Akure Nigeria 847903 MEDIUM 6 1608824345 Antipolo Philippines 887399 MEDIUM 789 1368838855 Ar RamÄdÄ<< Iraq 874543 MEDIUM 1356410365 Bangalore 1764068610 Bangkok India 13999000 MEGA Thailand 17573000 MEGA
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter10: Object-oriented Programming
Section: Chapter Questions
Problem 11RQ
Related questions
Question

Transcribed Image Text:UML Diagram of City Class:
City
- cityID: int
- cityName: String
- country:String
- population: int
+City()
+City(cityID: int, cityName: String, country:String, country: String,
population: int)
+setCityID(cityID:int): void
+getCityID():int
+setCityName(cityName: String): void
+getCityName(): String
+setCountry(country:String): void
+getCountry(): String
+setPopulation (population:int): void
+getPopulation():int
+getCityCategory(): String
+toString:String

Transcribed Image Text:Assignment Instructions:
You are tasked with developing a program to use city data from an online database and generate a
city details report.
1) Create a new Project in Eclipse called "HW7".
2) Create a class "City.java" in the project and implement the UML diagram shown below and add
comments to your program.
3) The logic for the method "getCityCategory" of City Class is below:
a. If the population of a city is greater than 10000000, then the method returns "MEGA"
b. If the population of a city is greater than 1000000 and less than 10000000, then the
method returns "LARGE"
c. If the population of a city is greater than 100000 and less than 1000000, then the method
returns "MEDIUM"
d. If the population of a city is below 100000, then the method returns "SMALL"
4) You should create another new Java program inside the project. Name the program as
"xxxx_program.java”, where xxxx is your Kean username.
3) Implement the following methods inside the xxxx_program program
The main method calls another method “readfromFile" which reads each line from the given
csv file "worldcities.csv"
-
The readfromFile method will store the data in an array of objects for City class.
-
Create a generateReport method that generates the "CityReport.csv" file.
-
The headings are added as shown below in sample output. Include comments in your program
Sample screenshot of the output "CityReport.csv" file
1 CityID
City Name
2
1566979497 Abeokuta
Country
Nigeria
Population
City Category
888924 MEDIUM
3
1682452296 Ad DammÄm
Saudi Arabia
903312 MEDIUM
4
1356304381 Ahmedabad
India
7717000 LARGE
5
1566568277 Akure
Nigeria
847903 MEDIUM
6
1608824345 Antipolo
Philippines
887399 MEDIUM
789
1368838855 Ar RamÄdÄ<<
Iraq
874543 MEDIUM
1356410365 Bangalore
1764068610 Bangkok
India
13999000 MEGA
Thailand
17573000 MEGA
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
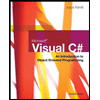
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
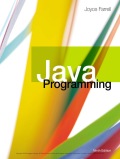
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
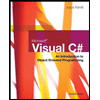
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
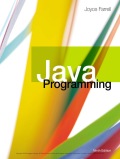
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
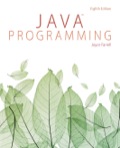
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
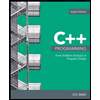
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
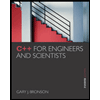
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr